In this example we will show how the microservice communicate with each other in Docker environment.
We will have two microservice project
1- We will create a producer microservice that will produce the data.
2- Frist one will create consumer microservice which will consume rest service by producer microservice.
3- for making commnication between this two microservice we will create a network called con-pro-communication
Lets first create prodcuer MS.
1- We will create a producer microservice that will produce the data.
Follow below step relegiously
Add following files
1-SpringbootDockerProducerApplication
1 2 3 4 5 6 7 8 9 10 11 12 13 | package com.siddhu; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class SpringbootDockerProducerApplication { public static void main(String[] args) { SpringApplication.run(SpringbootDockerProducerApplication.class, args); } } |
2- SpringbootDockerProducerController
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | package com.siddhu.controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; import com.siddhu.model.Employee; @RestController public class SpringbootDockerProducerController { @RequestMapping(value = "/employee", method = RequestMethod.GET) public Employee firstPage() { Employee emp = new Employee(); emp.setEmpId("1"); emp.setName("Siddhu"); emp.setSalary(3000); return emp; } } |
3- Employee
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | package com.siddhu.model; public class Employee { private String empId; private String name; private double salary; public Employee() { } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getSalary() { return salary; } public void setSalary(double salary) { this.salary = salary; } public String getEmpId() { return empId; } public void setEmpId(String empId) { this.empId = empId; } @Override public String toString() { return "Employee [empId=" + empId + ", name=" + name + ", salary=" + salary + "]"; } } |
4- application.properties
server.port=8091
compile the project and run and hit the url
http://localhost:8091/employee
you will get this output.
{
“empId”: “1”,
“name”: “Siddhu”,
“salary”: 3000
}

Now lets create consumer microservices
2- Frist one will create consumer microservice which will consume rest service by producer microservice.
Follow below step religiously
Now create following files
1- SpringbootDockerConsumerApplication
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | package com.siddhu; import java.io.IOException; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ApplicationContext; import org.springframework.web.client.RestClientException; @SpringBootApplication public class SpringbootDockerConsumerApplication { public static void main(String[] args) throws RestClientException, IOException { ApplicationContext ctx = SpringApplication.run( SpringbootDockerConsumerApplication.class, args); } } |
2- SiddhuConsumerController
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | package com.siddhu.controller; import java.io.IOException; import org.springframework.http.HttpEntity; import org.springframework.http.HttpHeaders; import org.springframework.http.HttpMethod; import org.springframework.http.MediaType; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate; @RestController public class SiddhuConsumerController { @GetMapping("/getEmployee") public ResponseEntity getEmployeeDetails() { String baseUrl = "http://localhost:8091/employee"; RestTemplate restTemplate = new RestTemplate(); ResponseEntity<String> response=null; try{ response=restTemplate.exchange(baseUrl, HttpMethod.GET, getHeaders(),String.class); }catch (Exception ex) { System.out.println(ex); } System.out.println("Simple data message showing success call :- " + response.getBody()); return response; } private static HttpEntity<?> getHeaders() throws IOException { HttpHeaders headers = new HttpHeaders(); headers.set("Accept", MediaType.APPLICATION_JSON_VALUE); return new HttpEntity<>(headers); } } |
3- application.properties
server.port=8090
Now lets run first our Producer MS and then run our above Consumer MS.
Hit the url of the consumer and check if we are able to get the resoponse from the producer.
Now lets create a docker files for the producer and consumer projects.
By doing this we will be able to create the docker image in docker engine and will be able to call the producer Ms from consumer MS.
For this we need to make some changes in the code. In docker we need to change our consumer as it is calling producer with localhost:9081 but in docker we will call it with name.
String baseUrl = “http://siddhuproducer:8091/employee”;
now once again run the maven build for consumer.
For consumer
From openjdk:8
copy ./target/springboot-docker-consumer-0.0.1-SNAPSHOT.jar springboot-docker-consumer-0.0.1-SNAPSHOT.jar
CMD [“java”,”-jar”,”springboot-docker-consumer-0.0.1-SNAPSHOT.jar”]
for producer
From openjdk:8
copy ./target/springboot-docker-producer-0.0.1-SNAPSHOT.jar springboot-docker-producer-0.0.1-SNAPSHOT.jar
CMD [“java”,”-jar”,”springboot-docker-producer-0.0.1-SNAPSHOT.jar”]
Now in docker to make the different MS to call eachother we need to make them run in same network.
So lets creat a network using below command
docker network create siddhu-ms-consumer-producer
Note:- We are using windows machine so using docker desktop.
check docker is installed
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | C:\Users\Siddhartha>docker Usage: docker [OPTIONS] COMMAND A self-sufficient runtime for containers Options: --config string Location of client config files (default "C:\\Users\\Siddhartha\\.docker") -c, --context string Name of the context to use to connect to the daemon (overrides DOCKER_HOST env var and default context set with "docker context use") -D, --debug Enable debug mode -H, --host list Daemon socket(s) to connect to -l, --log-level string Set the logging level ("debug"|"info"|"warn"|"error"|"fatal") (default "info") --tls Use TLS; implied by --tlsverify --tlscacert string Trust certs signed only by this CA (default "C:\\Users\\Siddhartha\\.docker\\ca.pem") --tlscert string Path to TLS certificate file (default "C:\\Users\\Siddhartha\\.docker\\cert.pem") --tlskey string Path to TLS key file (default "C:\\Users\\Siddhartha\\.docker\\key.pem") --tlsverify Use TLS and verify the remote -v, --version Print version information and quit |
now start the docker desktop using belwo configurations.

Now lets create a network by executing below command
docker network create siddhu-ms-consumer-producer
C:\STS-Workspace\springboot-docker-producer>docker network create siddhu-ms-consumer-producer
700cf66bb0d2f696bb4f0e4c424a91082280b9ab448296ee8e0793e34232cb26

Now lets start producer first using below command.
Now open the terminal and go to the Spring Boot springboot-docker-producer project folder.
Next we will build an image with the name producer.
docker image build -t springboot-docker-producer .

Now open the terminal and go to the Spring Boot springboot-docker-consumer` project folder.
Next we will build an image with the name consumer.
1 | docker image build -t springboot-docker-consumer . |

Now let run both the images one by one. First we will run Producer
docker container run –network siddhu-ms-consumer-producer –name siddhuproducer -p 8091:8091 -d springboot-docker-producer
During our execution we get belwo error
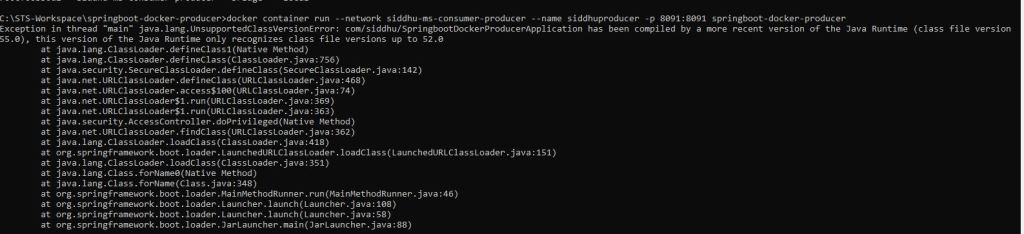
This indicate that image we are using is on less java version i.e. 8 and our war is build on jdk 11 so lets change the docker file again and build it again. i.e. in both the Dockerfile change the JDK to 11.

now again run the command for producer
docker container run –network siddhu-ms-consumer-producer –name siddhuproducer -p 8091:8091 -d springboot-docker-producer

hit the url on browser and you will get the output.
http://localhost:8091/employee

Now lets run the consumer
docker container run –network siddhu-ms-consumer-producer –name siddhuconsumer -p 8090:8090 -d springboot-docker-consumer

now finally hit the url and see we are able to fetch the producer output from consumer MS.

Lets push this both images on our siddhu docker hub using below command.
Login to our dockerhub site and creae a repo named as springboot-docker-consumer and springboot-docker-producer.
Now execute following below commmands on cmd prompts.
C:\STS-Workspace\springboot-docker-consumer>docker login –username shdhumale
Password:
Login Succeeded
C:\STS-Workspace\springboot-docker-consumer>docker tag springboot-docker-consumer shdhumale/springboot-docker-consumer
C:\STS-Workspace\springboot-docker-consumer>docker push shdhumale/springboot-docker-consumer
C:\STS-Workspace\springboot-docker-producer>docker tag springboot-docker-producer shdhumale/springboot-docker-producer
C:\STS-Workspace\springboot-docker-producer>docker push shdhumale/springboot-docker-producer
Now above thigs we had done using many command for docker. There is a concept called docker compose where we can combine both docker file into one and execute the same. Lets try to do the same.
First check we have docker compose available in our sytem
1 2 3 4 5 | C:\STS-Workspace\springboot-docker-consumer>docker-compose version docker-compose version 1.29.0, build 07737305 docker-py version: 5.0.0 CPython version: 3.9.0 OpenSSL version: OpenSSL 1.1.1g 21 Apr 2020 |
now create a docker compose file as shown below
version: “3”
services:
siddhuconsumer:
image: shdhumale/springboot-docker-consumer
ports:
– “8090:8090”
networks:
– siddhu-ms-consumer-producer
depends_on:
– siddhuproducer
siddhuproducer:
image: shdhumale/springboot-docker-producer
ports:
– “8091:8091”
networks:
– siddhu-ms-consumer-producer
networks:
siddhu-ms-consumer-producer:
Now lets delete all the images and container if running and then we will execute our docker-compose file
execute docker-compose up command.

If you want to stop all above containers at on go rather then using docker stop <nameofcontainer> using docker-decompose use belwo command
docker-compose down
note:- You can download the code from the given github location.
consumer :-
https://github.com/shdhumale/springboot-docker-consumer.git
Producer:-
https://github.com/shdhumale/springboot-docker-producer.git
No comments:
Post a Comment