During unit testing of the application manytimes it is not possible to replicate exact production environment or to connect DB it become difficult to do the unit testing. To deal with such limitations Mockitto provide api to create mock for these unavailable resources.Mockito is a mocking framework that tastes really good. It lets you write beautiful tests with a clean & simple API. It is open source https://site.mockito.org/ and code can be downloaded from https://github.com/mockito/mockito
Few of the below example gives you details how we can use the concept in real world
1- SiddhuAddServiceTest
package siddhuetheremwebexample.Mockito;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.mockito.Spy;
import org.mockito.junit.MockitoJUnitRunner;
//Refer to https://javacodehouse.com/blog/mockito-tutorial/ for more example.
//1- to add @RunWith(MockitoJUnitRunner.class) so that inner mock class object is created first.
@RunWith(MockitoJUnitRunner.class)
public class SiddhuAddServiceTest {
//use to call the original function rather then mockup.
@Spy
SiddhuAddServiceImpl objSpyAddServiceImpl;
//2- This is the place till where we want our code to be executed and rest code after this class need to be mocked.
@InjectMocks
SiddhuAddService objSiddhuAddService;
//3- this indicate which class need to be mocked. Generally it should be the all class/interface object which we are creating in @InjectMocks class
@Mock
SiddhuAddServiceInterface objSiddhuAddServiceInterface;
//4-This will indicate method/function from where junit will be executed.
//we can also use the below method to initialize mocks if we did not want to do with line 1-
/*
* @Before public void setUp() throws Exception {
*
* MockitoAnnotations.initMocks(this); }
*/ @Test
public void testSiddhuCalc() {
System.out.println("Test testSiddhuCalc Started");
//we have done mock using annotation above at 3- above method else we can also do the mock like below inside the method.
//addService = Mockito.mock(AddService.class);
objSiddhuAddService = new SiddhuAddService(objSiddhuAddServiceInterface);
int oneNum = 5;
int secondNum = 6;
int expected = 11;
//5-As we had said in 3 that objSiddhuAddServiceInterface are mocked then we need to make sure it should return mock value when ever it is called.
when(objSiddhuAddServiceInterface.addMethod(oneNum, secondNum)).thenReturn(expected);
//this will check addService add method is called depending on times(). As it is times(0) this means it is never called as it is mocked.
verify(objSiddhuAddServiceInterface, times(0)).addMethod(oneNum, secondNum);
//Here we called the real calc method of calcService which call addService.add(num1, num2); but as it is mocked and written in 5- we will always
//expected value as output and original call which implement this method AddServiceImpl will never be called.
int actual = objSiddhuAddService.calc(oneNum, secondNum);
assertEquals(expected, objSpyAddServiceImpl.addMethod(oneNum, secondNum));
// verify(objSpyAddServiceImpl).add(num1, num2);
assertEquals(expected, actual);
}
}
2-SiddhuAddServiceInterface
package siddhuetheremwebexample.Mockito;
public interface SiddhuAddServiceInterface {
public int addMethod(int oneNum, int secondNum);
}
3- SiddhuAddService
package siddhuetheremwebexample.Mockito;
public class SiddhuAddService {
private SiddhuAddServiceInterface objSiddhuAddServiceInterface;
public SiddhuAddService(SiddhuAddServiceInterface objSiddhuAddServiceInterface) {
this.objSiddhuAddServiceInterface = objSiddhuAddServiceInterface;
}
public int calc(int num1, int num2) {
System.out.println("**--- SiddhuAddService calc executed ---**");
return objSiddhuAddServiceInterface.addMethod(num1, num2);
}
}
4-SiddhuAddServiceImpl
package siddhuetheremwebexample.Mockito;
public class SiddhuAddServiceImpl implements SiddhuAddServiceInterface {
public int addMethod(int oneNum, int secondNum) {
System.out.println("----------------SiddhuAddServiceImpl addMethod called----------------");
return oneNum + secondNum;
}
}
5-POM.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>siddhuetheremwebexample</groupId>
<artifactId>Mockito</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>Mockito</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.3.3</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
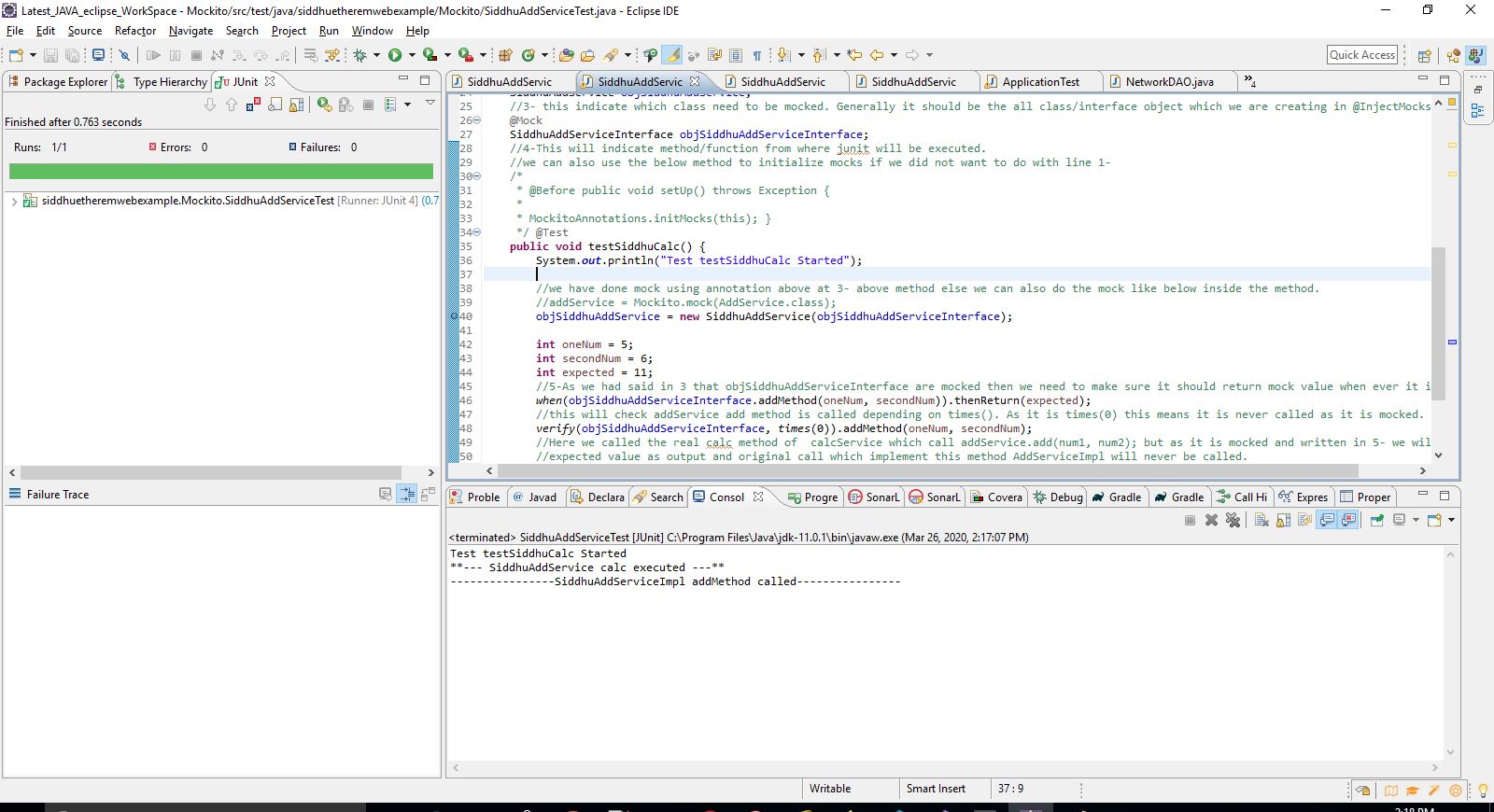
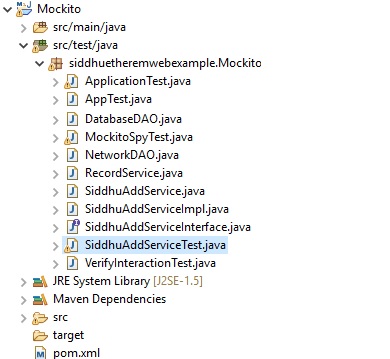
One of the good example I found from this site
https://howtodoinjava.com/mockito/junit-mockito-example/
1- ApplicationTest
package siddhuetheremwebexample.Mockito;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.Mockito;
import org.mockito.junit.MockitoJUnitRunner;
@RunWith(MockitoJUnitRunner.class)
public class ApplicationTest
{
@InjectMocks
RecordService recordService;
//@Mock
DatabaseDAO databaseMock = Mockito.mock(DatabaseDAO.class);
//@Mock
NetworkDAO networkMock= Mockito.mock(NetworkDAO.class);
@Test
public void saveTest()
{
boolean saved = recordService.save("temp.txt");
assertEquals(true, saved);
verify(databaseMock, times(1)).save("temp.txt");
verify(networkMock, times(1)).save("temp.txt");
}
}
2-RecordService
package siddhuetheremwebexample.Mockito;
public class RecordService
{
DatabaseDAO database;
NetworkDAO network;
//setters and getters
public boolean save(String fileName)
{
database.save(fileName);
System.out.println("Saved in database in Main class");
network.save(fileName);
System.out.println("Saved in network in Main class");
return true;
}
}
3-DatabaseDAO
package siddhuetheremwebexample.Mockito;
public class DatabaseDAO
{
public void save(String fileName) {
System.out.println("Saved in database");
}
}
4- NetworkDAO
package siddhuetheremwebexample.Mockito;
public class NetworkDAO
{
public void save(String fileName) {
System.out.println("Saved in network location");
}
}
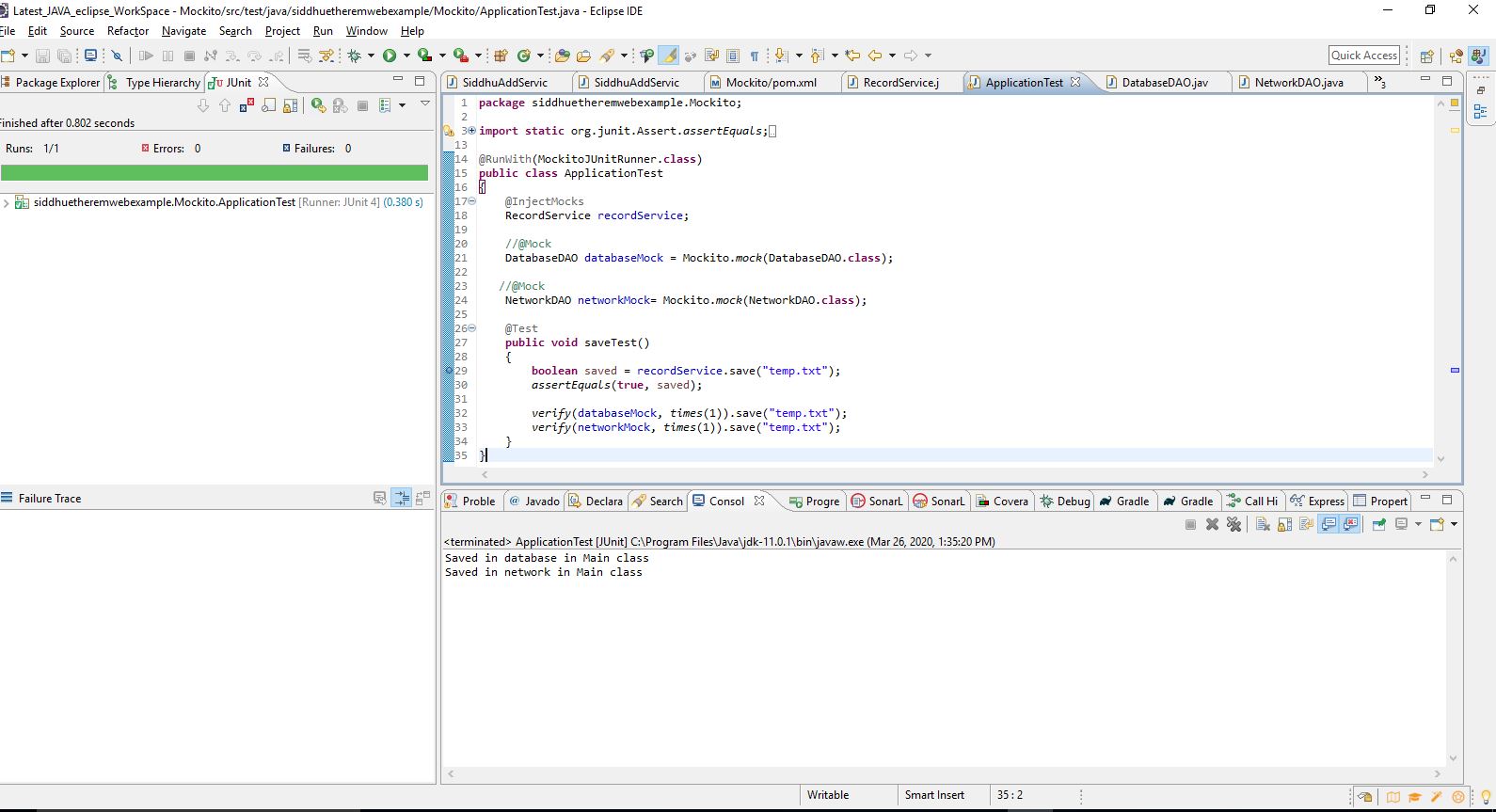
Reference:-
Refer to https://javacodehouse.com/blog/mockito-tutorial/ for more example.
Few of the below example gives you details how we can use the concept in real world
1- SiddhuAddServiceTest
package siddhuetheremwebexample.Mockito;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.mockito.Spy;
import org.mockito.junit.MockitoJUnitRunner;
//Refer to https://javacodehouse.com/blog/mockito-tutorial/ for more example.
//1- to add @RunWith(MockitoJUnitRunner.class) so that inner mock class object is created first.
@RunWith(MockitoJUnitRunner.class)
public class SiddhuAddServiceTest {
//use to call the original function rather then mockup.
@Spy
SiddhuAddServiceImpl objSpyAddServiceImpl;
//2- This is the place till where we want our code to be executed and rest code after this class need to be mocked.
@InjectMocks
SiddhuAddService objSiddhuAddService;
//3- this indicate which class need to be mocked. Generally it should be the all class/interface object which we are creating in @InjectMocks class
@Mock
SiddhuAddServiceInterface objSiddhuAddServiceInterface;
//4-This will indicate method/function from where junit will be executed.
//we can also use the below method to initialize mocks if we did not want to do with line 1-
/*
* @Before public void setUp() throws Exception {
*
* MockitoAnnotations.initMocks(this); }
*/ @Test
public void testSiddhuCalc() {
System.out.println("Test testSiddhuCalc Started");
//we have done mock using annotation above at 3- above method else we can also do the mock like below inside the method.
//addService = Mockito.mock(AddService.class);
objSiddhuAddService = new SiddhuAddService(objSiddhuAddServiceInterface);
int oneNum = 5;
int secondNum = 6;
int expected = 11;
//5-As we had said in 3 that objSiddhuAddServiceInterface are mocked then we need to make sure it should return mock value when ever it is called.
when(objSiddhuAddServiceInterface.addMethod(oneNum, secondNum)).thenReturn(expected);
//this will check addService add method is called depending on times(). As it is times(0) this means it is never called as it is mocked.
verify(objSiddhuAddServiceInterface, times(0)).addMethod(oneNum, secondNum);
//Here we called the real calc method of calcService which call addService.add(num1, num2); but as it is mocked and written in 5- we will always
//expected value as output and original call which implement this method AddServiceImpl will never be called.
int actual = objSiddhuAddService.calc(oneNum, secondNum);
assertEquals(expected, objSpyAddServiceImpl.addMethod(oneNum, secondNum));
// verify(objSpyAddServiceImpl).add(num1, num2);
assertEquals(expected, actual);
}
}
2-SiddhuAddServiceInterface
package siddhuetheremwebexample.Mockito;
public interface SiddhuAddServiceInterface {
public int addMethod(int oneNum, int secondNum);
}
3- SiddhuAddService
package siddhuetheremwebexample.Mockito;
public class SiddhuAddService {
private SiddhuAddServiceInterface objSiddhuAddServiceInterface;
public SiddhuAddService(SiddhuAddServiceInterface objSiddhuAddServiceInterface) {
this.objSiddhuAddServiceInterface = objSiddhuAddServiceInterface;
}
public int calc(int num1, int num2) {
System.out.println("**--- SiddhuAddService calc executed ---**");
return objSiddhuAddServiceInterface.addMethod(num1, num2);
}
}
4-SiddhuAddServiceImpl
package siddhuetheremwebexample.Mockito;
public class SiddhuAddServiceImpl implements SiddhuAddServiceInterface {
public int addMethod(int oneNum, int secondNum) {
System.out.println("----------------SiddhuAddServiceImpl addMethod called----------------");
return oneNum + secondNum;
}
}
5-POM.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>siddhuetheremwebexample</groupId>
<artifactId>Mockito</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>Mockito</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.3.3</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
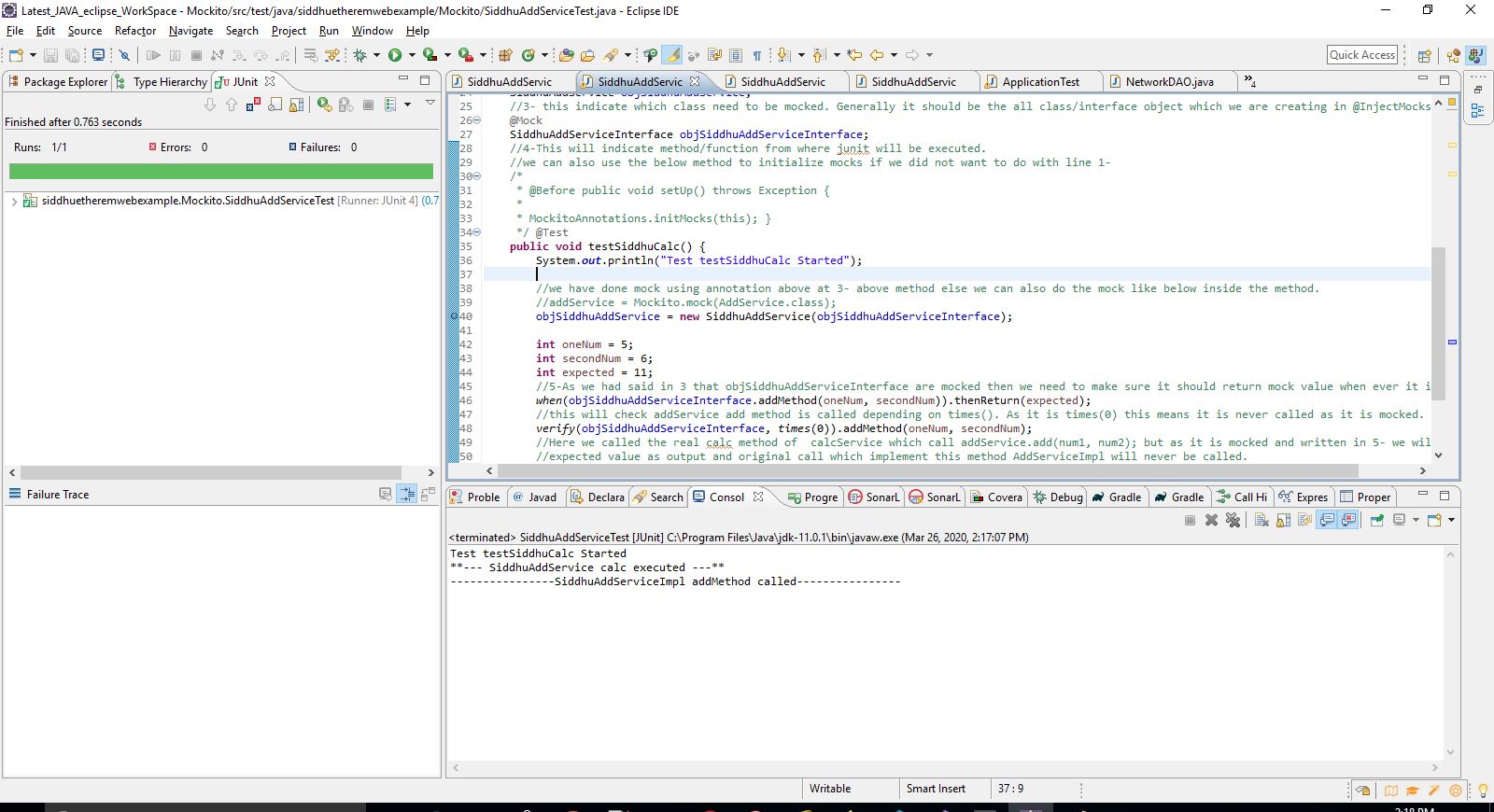
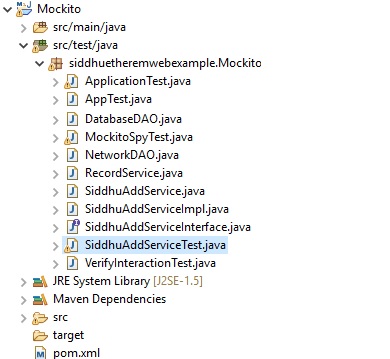
One of the good example I found from this site
https://howtodoinjava.com/mockito/junit-mockito-example/
1- ApplicationTest
package siddhuetheremwebexample.Mockito;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.Mockito;
import org.mockito.junit.MockitoJUnitRunner;
@RunWith(MockitoJUnitRunner.class)
public class ApplicationTest
{
@InjectMocks
RecordService recordService;
//@Mock
DatabaseDAO databaseMock = Mockito.mock(DatabaseDAO.class);
//@Mock
NetworkDAO networkMock= Mockito.mock(NetworkDAO.class);
@Test
public void saveTest()
{
boolean saved = recordService.save("temp.txt");
assertEquals(true, saved);
verify(databaseMock, times(1)).save("temp.txt");
verify(networkMock, times(1)).save("temp.txt");
}
}
2-RecordService
package siddhuetheremwebexample.Mockito;
public class RecordService
{
DatabaseDAO database;
NetworkDAO network;
//setters and getters
public boolean save(String fileName)
{
database.save(fileName);
System.out.println("Saved in database in Main class");
network.save(fileName);
System.out.println("Saved in network in Main class");
return true;
}
}
3-DatabaseDAO
package siddhuetheremwebexample.Mockito;
public class DatabaseDAO
{
public void save(String fileName) {
System.out.println("Saved in database");
}
}
4- NetworkDAO
package siddhuetheremwebexample.Mockito;
public class NetworkDAO
{
public void save(String fileName) {
System.out.println("Saved in network location");
}
}
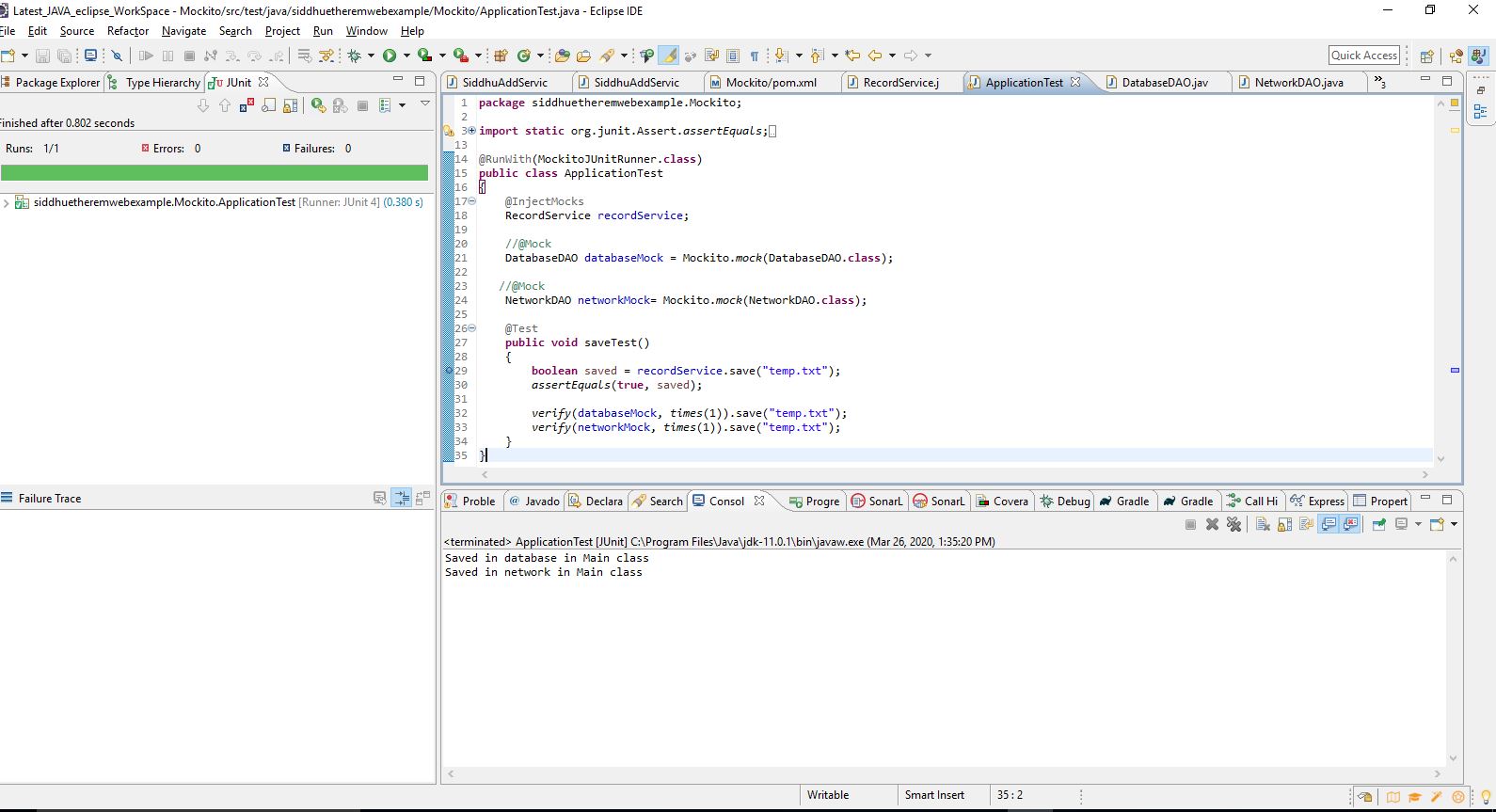
Reference:-
Refer to https://javacodehouse.com/blog/mockito-tutorial/ for more example.