Let try to build GWT MVP Pattern example. This will ask user to enter two number and in return will give addition of this two number.
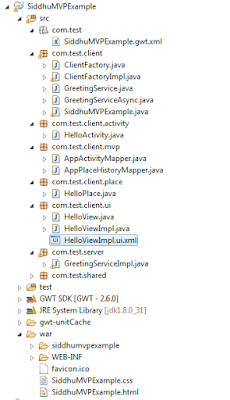 |
Eclipse View of GWT MVP Project |
This are the code of the files :-
SiddhuMVPExample.html
SiddhuMVPExample.gwt.xml
HelloViewImpl.ui.xml
HelloView
package com.test.client.ui;
import com.google.gwt.place.shared.Place;
import com.google.gwt.user.client.ui.IsWidget;
public interface HelloView extends IsWidget
{
String getFirstNumber();
String getSecondNumber();
void setAnswer(String answer);
void setPresenter(Presenter listener);
public interface Presenter
{
void goTo(Place place);
void buttonAddClicked();
}
}
HelloViewImpl
package com.test.client.ui;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.uibinder.client.UiBinder;
import com.google.gwt.uibinder.client.UiField;
import com.google.gwt.uibinder.client.UiHandler;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.TextBox;
import com.google.gwt.user.client.ui.Widget;
public class HelloViewImpl extends Composite implements HelloView
{
private static HelloViewImplUiBinder uiBinder = GWT.create(HelloViewImplUiBinder.class);
interface HelloViewImplUiBinder extends UiBinder
{
}
@UiField TextBox FirstNumber;
@UiField TextBox SecondNumber;
@UiField TextBox Answer;
private Presenter listener;
public HelloViewImpl()
{
initWidget(uiBinder.createAndBindUi(this));
}
@Override
public void setPresenter(Presenter listener)
{
this.listener = listener;
}
@Override
public String getFirstNumber() {
return FirstNumber.getText();
}
@Override
public String getSecondNumber() {
return SecondNumber.getText();
}
@Override
public void setAnswer(String answer) {
Answer.setText(answer);
}
@UiHandler("buttonAdd")
void buttonAddClicked(ClickEvent e)
{
listener.buttonAddClicked();
}
}
HelloPlace
package com.test.client.place;
import com.google.gwt.place.shared.Place;
import com.google.gwt.place.shared.PlaceTokenizer;
public class HelloPlace extends Place
{
private String firstNumber;
private String secondNumber;
private String answer;
private String helloName;
public String getFirstNumber() {
return firstNumber;
}
public void setFirstNumber(String firstNumber) {
this.firstNumber = firstNumber;
}
public String getSecondNumber() {
return secondNumber;
}
public void setSecondNumber(String secondNumber) {
this.secondNumber = secondNumber;
}
public String getAnswer() {
return answer;
}
public void setAnswer(String answer) {
this.answer = answer;
}
public HelloPlace(String token)
{
this.helloName = token;
}
public String getHelloName()
{
return helloName;
}
public static class Tokenizer implements PlaceTokenizer
{
@Override
public String getToken(HelloPlace place)
{
return place.getHelloName();
}
@Override
public HelloPlace getPlace(String token)
{
return new HelloPlace(token);
}
}
}
AppActivityMapper
package com.test.client.mvp;
import com.google.gwt.activity.shared.Activity;
import com.google.gwt.activity.shared.ActivityMapper;
import com.google.gwt.place.shared.Place;
import com.test.client.ClientFactory;
import com.test.client.activity.HelloActivity;
import com.test.client.place.HelloPlace;
public class AppActivityMapper implements ActivityMapper {
private ClientFactory clientFactory;
/**
* AppActivityMapper associates each Place with its corresponding
* {@link Activity}
*
* @param clientFactory
* Factory to be passed to activities
*/
public AppActivityMapper(ClientFactory clientFactory) {
super();
this.clientFactory = clientFactory;
}
/**
* Map each Place to its corresponding Activity. This would be a great use
* for GIN.
*/
@Override
public Activity getActivity(Place place) {
// This is begging for GIN
if (place instanceof HelloPlace)
return new HelloActivity((HelloPlace) place, clientFactory);
else
return null;
}
}
AppPlaceHistoryMapper
package com.test.client.mvp;
import com.google.gwt.place.shared.PlaceHistoryMapper;
import com.google.gwt.place.shared.WithTokenizers;
import com.test.client.place.HelloPlace;
/**
* PlaceHistoryMapper interface is used to attach all places which the
* PlaceHistoryHandler should be aware of. This is done via the @WithTokenizers
* annotation or by extending PlaceHistoryMapperWithFactory and creating a
* separate TokenizerFactory.
*/
@WithTokenizers( { HelloPlace.Tokenizer.class })
public interface AppPlaceHistoryMapper extends PlaceHistoryMapper {
}
HelloActivity
package com.test.client.activity;
import com.google.gwt.activity.shared.AbstractActivity;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.shared.EventBus;
import com.google.gwt.place.shared.Place;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.google.gwt.user.client.ui.AcceptsOneWidget;
import com.test.client.ClientFactory;
import com.test.client.GreetingService;
import com.test.client.GreetingServiceAsync;
import com.test.client.place.HelloPlace;
public class HelloActivity extends AbstractActivity implements com.test.client.ui.HelloView.Presenter {
// Used to obtain views, eventBus, placeController
// Alternatively, could be injected via GIN
private ClientFactory clientFactory;
// Name that will be appended to "Hello,"
//private String answer;
com.test.client.ui.HelloView helloView;
private final GreetingServiceAsync greetingService = GWT
.create(GreetingService.class);
public HelloActivity(HelloPlace place, ClientFactory clientFactory) {
//this.answer = place.getAnswer();
this.clientFactory = clientFactory;
}
/**
* Invoked by the ActivityManager to start a new Activity
*/
@Override
public void start(AcceptsOneWidget containerWidget, EventBus eventBus) {
helloView = clientFactory.getHelloView();
helloView.setPresenter(this);
containerWidget.setWidget(helloView.asWidget());
}
/**
* Ask user before stopping this activity
*/
@Override
public String mayStop() {
return "Please hold on. This activity is stopping.";
}
/**
* Navigate to a new Place in the browser
*/
public void goTo(Place place) {
clientFactory.getPlaceController().goTo(place);
}
@Override
public void buttonAddClicked() {
String fnumber = helloView.getFirstNumber();
String snumber = helloView.getSecondNumber();
// to check if code is coming here we can add following below line and can check we are getting answer
// From here only our RPC call is remaining.
/*int answer = Integer.parseInt(fnumber) + Integer.parseInt(snumber);
helloView.setAnswer(""+answer);*/
greetingService.getAddValue(fnumber, snumber, new AsyncCallback() {
@Override
public void onSuccess(String result) {
helloView.setAnswer(result);
}
@Override
public void onFailure(Throwable caught) {
helloView.setAnswer(""+caught);
}
});
}
}
ClientFactory
package com.test.client;
import com.google.gwt.event.shared.EventBus;
import com.google.gwt.place.shared.PlaceController;
import com.test.client.ui.HelloView;
public interface ClientFactory
{
EventBus getEventBus();
PlaceController getPlaceController();
HelloView getHelloView();
}
ClientFactoryImpl
package com.test.client;
import com.google.gwt.event.shared.EventBus;
import com.google.gwt.event.shared.SimpleEventBus;
import com.google.gwt.place.shared.PlaceController;
import com.test.client.ui.HelloView;
import com.test.client.ui.HelloViewImpl;
public class ClientFactoryImpl implements ClientFactory
{
private static final EventBus eventBus = new SimpleEventBus();
private static final PlaceController placeController = new PlaceController(eventBus);
private static final HelloView helloView = new HelloViewImpl();
@Override
public EventBus getEventBus()
{
return eventBus;
}
@Override
public HelloView getHelloView()
{
return helloView;
}
@Override
public PlaceController getPlaceController()
{
return placeController;
}
}
GreetingService
package com.test.client;
import com.google.gwt.user.client.rpc.RemoteService;
import com.google.gwt.user.client.rpc.RemoteServiceRelativePath;
/**
* The client-side stub for the RPC service.
*/
@RemoteServiceRelativePath("greet")
public interface GreetingService extends RemoteService {
String getAddValue(String firstNumber, String secondNumber) throws IllegalArgumentException;
}
GreetingServiceAsync
package com.test.client;
import com.google.gwt.user.client.rpc.AsyncCallback;
/**
* The async counterpart of GreetingService
.
*/
public interface GreetingServiceAsync {
void getAddValue(String firstNumber, String secondNumber, AsyncCallback callback)
throws IllegalArgumentException;
}
SiddhuMVPExample
package com.test.client;
import com.google.gwt.activity.shared.ActivityManager;
import com.google.gwt.activity.shared.ActivityMapper;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.shared.EventBus;
import com.google.gwt.place.shared.Place;
import com.google.gwt.place.shared.PlaceController;
import com.google.gwt.place.shared.PlaceHistoryHandler;
import com.google.gwt.user.client.ui.RootPanel;
import com.google.gwt.user.client.ui.SimplePanel;
import com.test.client.mvp.AppActivityMapper;
import com.test.client.mvp.AppPlaceHistoryMapper;
import com.test.client.place.HelloPlace;
/**
* Entry point classes define onModuleLoad()
.
*/
public class SiddhuMVPExample implements EntryPoint {
/**
* Create a remote service proxy to talk to the server-side Greeting service.
*/
private final GreetingServiceAsync greetingService = GWT
.create(GreetingService.class);
private Place defaultPlace = new HelloPlace("World!");
private SimplePanel appWidget = new SimplePanel();
/**
* This is the entry point method.
*
*/
public void onModuleLoad() {
// Create ClientFactory using deferred binding so we can replace with different
// impls in gwt.xml
ClientFactory clientFactory = GWT.create(ClientFactory.class);
EventBus eventBus = clientFactory.getEventBus();
PlaceController placeController = clientFactory.getPlaceController();
// Start ActivityManager for the main widget with our ActivityMapper
ActivityMapper activityMapper = new AppActivityMapper(clientFactory);
ActivityManager activityManager = new ActivityManager(activityMapper, eventBus);
activityManager.setDisplay(appWidget);
// Start PlaceHistoryHandler with our PlaceHistoryMapper
AppPlaceHistoryMapper historyMapper= GWT.create(AppPlaceHistoryMapper.class);
PlaceHistoryHandler historyHandler = new PlaceHistoryHandler(historyMapper);
historyHandler.register(placeController, eventBus, defaultPlace);
RootPanel.get().add(appWidget);
// Goes to place represented on URL or default place
historyHandler.handleCurrentHistory();
}
}
FieldVerifier
package com.test.shared;
/**
*
* FieldVerifier validates that the name the user enters is valid.
*
*
* This class is in the shared
package because we use it in both
* the client code and on the server. On the client, we verify that the name is
* valid before sending an RPC request so the user doesn't have to wait for a
* network round trip to get feedback. On the server, we verify that the name is
* correct to ensure that the input is correct regardless of where the RPC
* originates.
*
*
* When creating a class that is used on both the client and the server, be sure
* that all code is translatable and does not use native JavaScript. Code that
* is not translatable (such as code that interacts with a database or the file
* system) cannot be compiled into client-side JavaScript. Code that uses native
* JavaScript (such as Widgets) cannot be run on the server.
*
*/
public class FieldVerifier {
/**
* Verifies that the specified name is valid for our service.
*
* In this example, we only require that the name is at least four
* characters. In your application, you can use more complex checks to ensure
* that usernames, passwords, email addresses, URLs, and other fields have the
* proper syntax.
*
* @param name the name to validate
* @return true if valid, false if invalid
*/
public static boolean isValidName(String name) {
if (name == null) {
return false;
}
return name.length() > 3;
}
}
package com.test.server;
import com.google.gwt.user.server.rpc.RemoteServiceServlet;
import com.test.client.GreetingService;
/**
* The server side implementation of the RPC service.
*/
@SuppressWarnings("serial")
public class GreetingServiceImpl extends RemoteServiceServlet implements GreetingService {
public String getAddValue(String firstNumber, String secondNumber) throws IllegalArgumentException {
String result;
int i=Integer.parseInt(firstNumber)+ Integer.parseInt(secondNumber);
result=String.valueOf(i);
return result;
}
}
You can get complete code file from :
https://drive.google.com/open?id=0B1EOBpl9Kvo1SzJOSmFBRTFkSzA