First we will install express generator to have express project in nodejs
Express comes in build with expressgenerator that helps us to set the default framework for creating Nodejs application using ExpressJS frame work.
https://expressjs.com/en/starter/generator.html
Lets try to use it
First create package.json using
npm init
then install express using
npm install express
check in package.json if express is install
install nodemon for auto refresh
npm install -g nodemon
then install express-generator using below command
npx express-generator
or
npm install -g express-generator
I prefer to use second command as it help me to further take view engine as my need i.e.
npm install -g express-generator
Note:- Use below command to create a project name as myapp and view as .pug or any of the template you want as an view engine.
express --view=ejs myapp
As we have our project folder and we dont want to create a new folder and want EJS as view, so we will first install EJS view using below command.
npm install ejs
Then we will create Folder structure
express --view=ejs
PS C:\Visual_Source_Code_WorkSpace_NodeJS\tailwindnodejs> express --view=ejs
destination is not empty, continue? [y/N] y
create : public\
create : public\javascripts\
create : public\images\
create : public\stylesheets\
create : public\stylesheets\style.css
create : routes\
create : routes\index.js
create : routes\users.js
create : views\
create : views\error.ejs
create : views\index.ejs
create : app.js
create : package.json
create : bin\
create : bin\www
install dependencies:
> npm install
run the app:
> SET DEBUG=tailwindnodejs:* & npm start
Finally confirm the our project created by Express Generator is working fine by executing the below command.
npm install
SET DEBUG=tailwindnodejs:* ; npm start
Check the url http://localhost:3000/ and you will be able to see
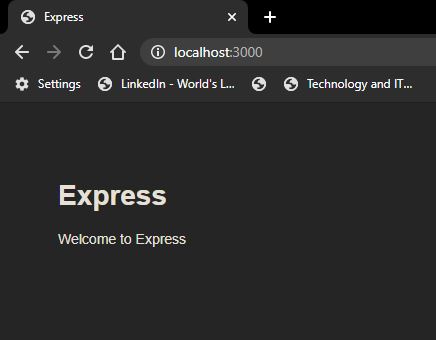
Now lets work on integration of tailwind with in our project
I want to keep my CSS files project.css intact and want to have one more CSS file specially for TailwindCSS.
So let's create a new CSS file inside \public\stylesheets\ and name it as source.css which is dedicated to TailwindCSS
1- Install tailwind
npm install tailwindcss
PS C:\Visual_Source_Code_WorkSpace_NodeJS\tailwindnodejs> npm install tailwindcss
+ tailwindcss@1.4.6
added 85 packages from 80 contributors and audited 140 packages in 42.052s
7 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
2- Add following lines in source.css files under \public\stylesheets\
@tailwind base;
@tailwind components;
@tailwind utilities;
3- As we want to generate our style.css file so you can actually use in your project. Simply use npx and generate the file.
npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css
they above command will create a new style.css that contains css of tailwindCSS
Good we have installed now tailwindcss. Now simply go into the file that hosts your layout for the project and add the stylesheet in your header
<link rel="stylesheet" href="/stylesheets/style.css" />
We can also added this command to my package.json and made it look like so :
"scripts": {
"start": "npm build:css && node ./bin/www",
"build:css": "npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css"
}
or
"scripts": {
"start": "node ./bin/www",
"build:css": "npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css"
}
Note:-
Optionally, if we would like to add extra configurations to our tailwindcss setup, you can initialize a settings file via npx:
npx tailwindcss init
This will create tailwind.config.js put this file into your root directory
// tailwind.config.js
module.exports = {
theme: {},
variants: {},
plugins: [
require('tailwindcss'),
require('autoprefixer')
],
};
Now let's have one simple TailwindBlock component added to our application from https://mertjf.github.io/tailblocks/.
We will have following components
1- Header
2- Footer
3- Blog
4- Contact
5- Content
6- CTA
7- E-Commerce
8- Feature
9- Gallery
10- Hero
11- Pricing
12- Statistic
13- Team
14- Testimonial
but we will use header, ecommerce and footer. After integration, we will be able to see he screen as shown below.

Now lets try to bring the hard code text from text files.
Let's create a new folder called as properties inside public folder and create following properties files for that we will use
https://www.npmjs.com/package/properties-reader
So first install properties module using below command
npm install properties-reader

Note: you can get downlaod the code from
https://github.com/shdhumale/tailwindnodejs
Express comes in build with expressgenerator that helps us to set the default framework for creating Nodejs application using ExpressJS frame work.
https://expressjs.com/en/starter/generator.html
Lets try to use it
First create package.json using
npm init
then install express using
npm install express
check in package.json if express is install
install nodemon for auto refresh
npm install -g nodemon
then install express-generator using below command
npx express-generator
or
npm install -g express-generator
I prefer to use second command as it help me to further take view engine as my need i.e.
npm install -g express-generator
Note:- Use below command to create a project name as myapp and view as .pug or any of the template you want as an view engine.
express --view=ejs myapp
As we have our project folder and we dont want to create a new folder and want EJS as view, so we will first install EJS view using below command.
npm install ejs
Then we will create Folder structure
express --view=ejs
PS C:\Visual_Source_Code_WorkSpace_NodeJS\tailwindnodejs> express --view=ejs
destination is not empty, continue? [y/N] y
create : public\
create : public\javascripts\
create : public\images\
create : public\stylesheets\
create : public\stylesheets\style.css
create : routes\
create : routes\index.js
create : routes\users.js
create : views\
create : views\error.ejs
create : views\index.ejs
create : app.js
create : package.json
create : bin\
create : bin\www
install dependencies:
> npm install
run the app:
> SET DEBUG=tailwindnodejs:* & npm start
Finally confirm the our project created by Express Generator is working fine by executing the below command.
npm install
SET DEBUG=tailwindnodejs:* ; npm start
Check the url http://localhost:3000/ and you will be able to see
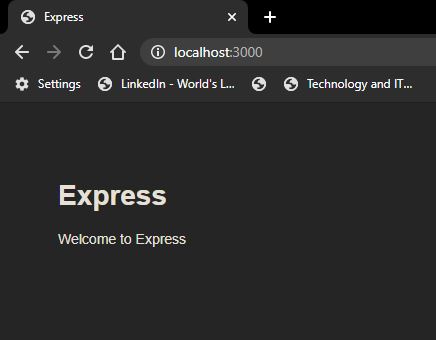
Now lets work on integration of tailwind with in our project
I want to keep my CSS files project.css intact and want to have one more CSS file specially for TailwindCSS.
So let's create a new CSS file inside \public\stylesheets\ and name it as source.css which is dedicated to TailwindCSS
1- Install tailwind
npm install tailwindcss
PS C:\Visual_Source_Code_WorkSpace_NodeJS\tailwindnodejs> npm install tailwindcss
+ tailwindcss@1.4.6
added 85 packages from 80 contributors and audited 140 packages in 42.052s
7 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
2- Add following lines in source.css files under \public\stylesheets\
@tailwind base;
@tailwind components;
@tailwind utilities;
3- As we want to generate our style.css file so you can actually use in your project. Simply use npx and generate the file.
npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css
they above command will create a new style.css that contains css of tailwindCSS
Good we have installed now tailwindcss. Now simply go into the file that hosts your layout for the project and add the stylesheet in your header
<link rel="stylesheet" href="/stylesheets/style.css" />
We can also added this command to my package.json and made it look like so :
"scripts": {
"start": "npm build:css && node ./bin/www",
"build:css": "npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css"
}
or
"scripts": {
"start": "node ./bin/www",
"build:css": "npx tailwindcss build ./public/stylesheets/source.css -o ./public/stylesheets/style.css"
}
Note:-
Optionally, if we would like to add extra configurations to our tailwindcss setup, you can initialize a settings file via npx:
npx tailwindcss init
This will create tailwind.config.js put this file into your root directory
// tailwind.config.js
module.exports = {
theme: {},
variants: {},
plugins: [
require('tailwindcss'),
require('autoprefixer')
],
};
Now let's have one simple TailwindBlock component added to our application from https://mertjf.github.io/tailblocks/.
We will have following components
1- Header
2- Footer
3- Blog
4- Contact
5- Content
6- CTA
7- E-Commerce
8- Feature
9- Gallery
10- Hero
11- Pricing
12- Statistic
13- Team
14- Testimonial
but we will use header, ecommerce and footer. After integration, we will be able to see he screen as shown below.

Now lets try to bring the hard code text from text files.
Let's create a new folder called as properties inside public folder and create following properties files for that we will use
https://www.npmjs.com/package/properties-reader
So first install properties module using below command
npm install properties-reader

Note: you can get downlaod the code from
https://github.com/shdhumale/tailwindnodejs