In some scenario when we exposed REST service user can perform the operation using that REST service and can upodate the record.
There are many ways to hold the user to do the same i.e using spring security and then using basic authorization but all this things just do the base64 encoding of the data which can be easily optained from network tab of the chrom and decoded from online site.
User can easily make the changes the refire the same request from the postman and can cheat the application ui.
The smart way is to use the combination of JWT JSON web token and Google Captcha combination to stop this.
Lets try to understand it.
JWT:- JSON web token is made up of three part
1- first part contain its name as alwasy JWT and its algoritium that it support i.e SHA256 etc
2- Second part contains the payload from the user to the server which we want the protect.
3- Third part is the comination of encrypting step 2 values Lets say this value as A and then ecrypting this A again with Secret key that is only present on server side. This make sure that the final JWT token that is created cannot be duplicated or modified for that perticular users.
Lets first develop a spring boot application with jwt.secret=siddhusecret.
You can download the code from belwo location
https://github.com/shdhumale/spring-boot-jwt.git
Note:- I had taken help from below url to understand the JWT and this code.
https://www.javainuse.com/spring/jwt
https://www.javainuse.com/spring/boot-jwt
Now let check if our jwt token thing is working fine
you can refer to the below screen of Postman in first screen we call api /authentication with our parameter in request body and we get JWT token. And in second screen we send this token as a header Bearer values to the server and get the out put Hello world.
Now lets create and Angular Project which support Google Captcha. You can refer to the previous blog for more understanding.
http://siddharathadhumale.blogspot.com/2021/11/gooogle-recaptcha-with-angular.html
Only change we had done is first we send request to the Springboot using Angular Js and then we get the JWT token as response. In addtion of getting JWT token we show the user to click Captcha "I am not robot" once the user click on this captcha check box we can play with business logic to send the data or JWT token back to the server as this JWT token contain that data for which user has click captcha.
FYI Captcha is not displayed in Postman and other tool so we are safe that no user can send data for creating JWT from Postman and then submit the request using Captcha.
You can use/download the AJS code from the belwo url.
https://github.com/shdhumale/angular-googlecaptcha-jwt.git
Please find belwo screen
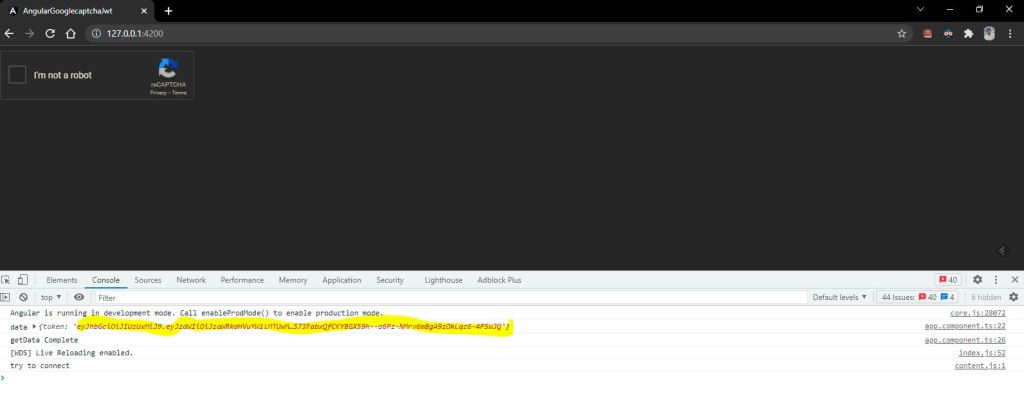
This screen show the user get the JWT token on from the server
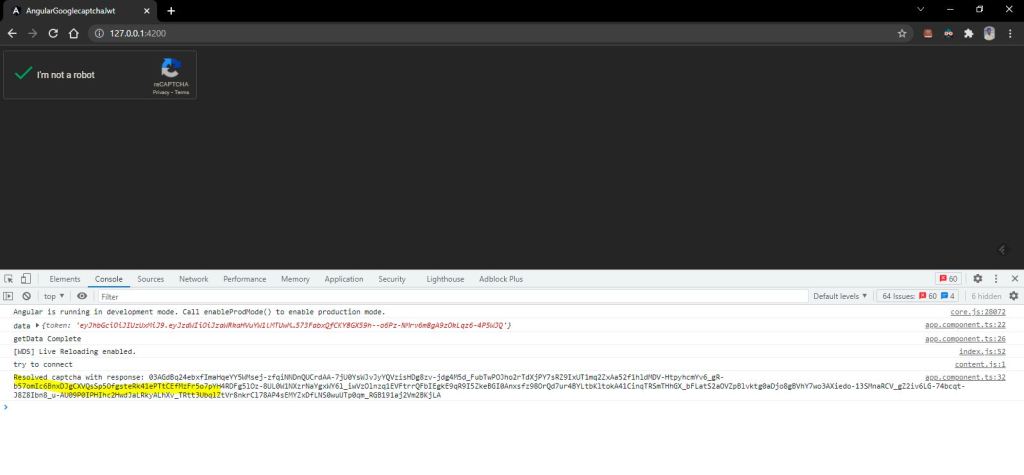
This image shows that we capture the click event on the Captcha check box and once we get he response we can send our JWT token back to the Server for insert/update in DB as at this time we are sure of following things.
1- JWT is create from the User that come from valid angular project
2- Captcha click use the above crated JWT(which cannot be modified) for final insert and update operation.