Spring Activiti is a lightweight, open-source BPMN (Business Process Model and Notation) platform designed for building and executing business workflows and processes. It is part of the larger Spring ecosystem and is commonly used in Java applications.
Here are some common use cases and benefits of using Spring Activiti:
Workflow Automation: Spring Activiti allows you to model and automate complex business processes, helping to streamline operations and reduce manual intervention.
Process Orchestration: It provides a framework for orchestrating various tasks and activities within a business process, ensuring they are executed in the correct sequence.
Human Task Management: It includes features for managing human tasks within a workflow, allowing for user interaction and decision-making as part of the process.
Integration with Spring Framework: Spring Activiti integrates seamlessly with the Spring framework, making it a popular choice for Java applications built using the Spring ecosystem.
Version Control: It supports versioning of process definitions, allowing for easy management of changes in business processes over time.
Monitoring and Analytics: Spring Activiti provides tools for monitoring the execution of processes, including dashboards and reporting features.
Multi-Tenancy Support: It allows for the deployment of multiple isolated process engines within a single application, making it suitable for scenarios where multiple tenants or clients need their own workflows.
Security and Authentication: It integrates with Spring Security for authentication and authorization, ensuring that processes are executed securely.
Extendable and Customizable: Spring Activiti is designed to be extensible, allowing developers to customize and extend its functionality to meet specific business requirements.
Integration with Other Systems: It supports integration with various external systems and services, making it possible to incorporate existing applications into the workflow.
Spring Activiti is used to consume bpmn file using java code. BMPN file are the business process notation model file that can be created either online or using Eclipse plugin
For Online use below url:-
In order to design the BPMN 2.0 process there are multiple options. You can try the an online Process designer http://demo.bpmn.io/
For configuring in Eclipse use the below concept.
Installing the Activiti Process Designer
In order to design the BPMN 2.0 processes we will install the Activiti Eclipse plugin so that we can design our process from a Development IDE.
From the Eclipse upper menu, select Help | Install New Software. Choose to add the following Repository: http://activiti.org/designer/update


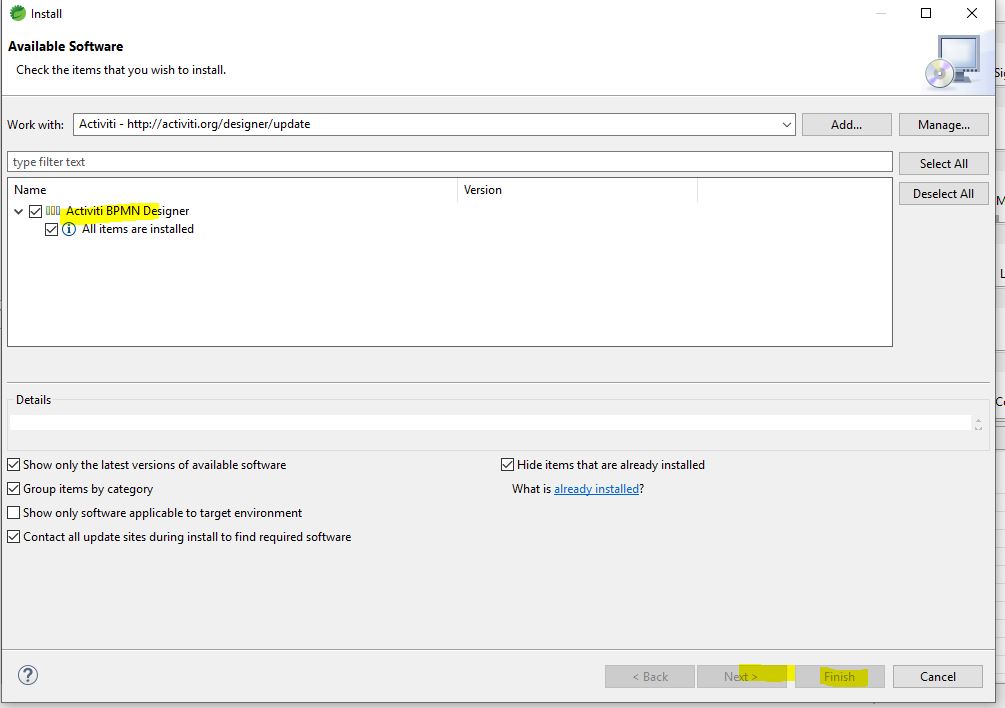
Once you install Activity in eclipse open the
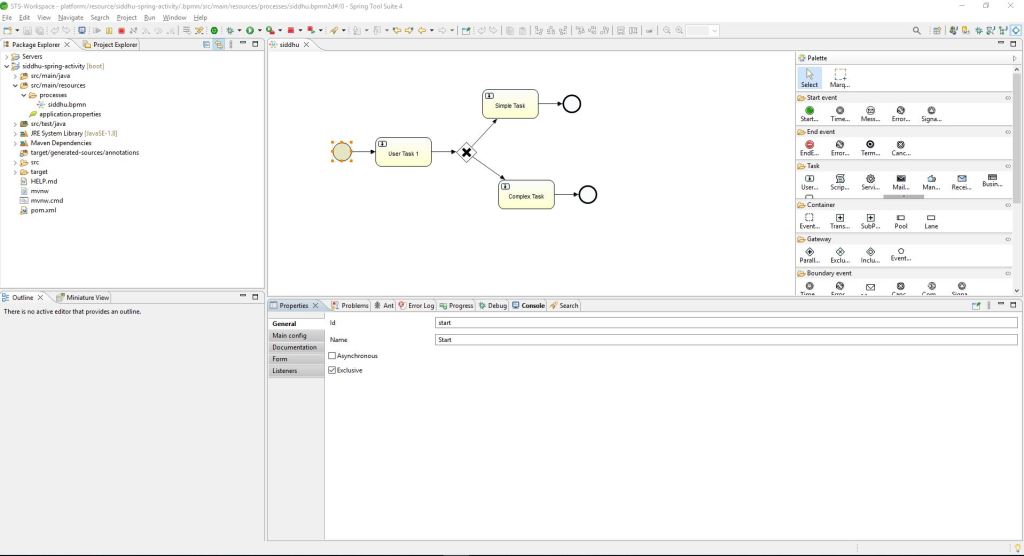
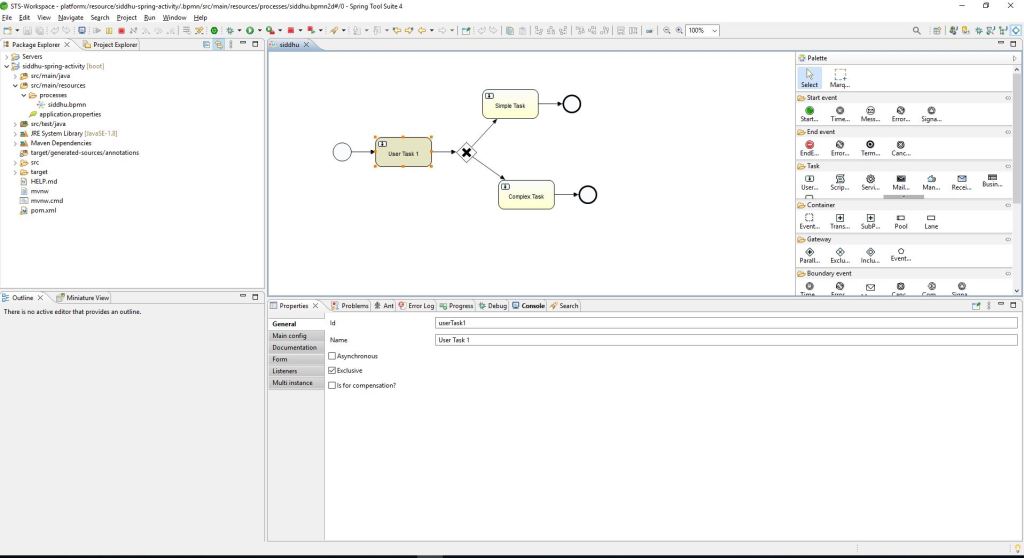


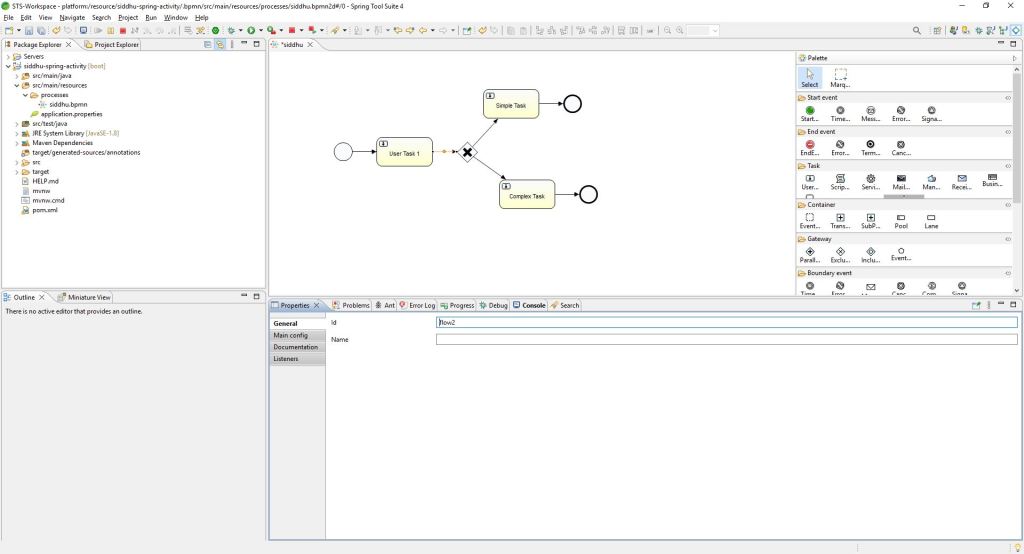







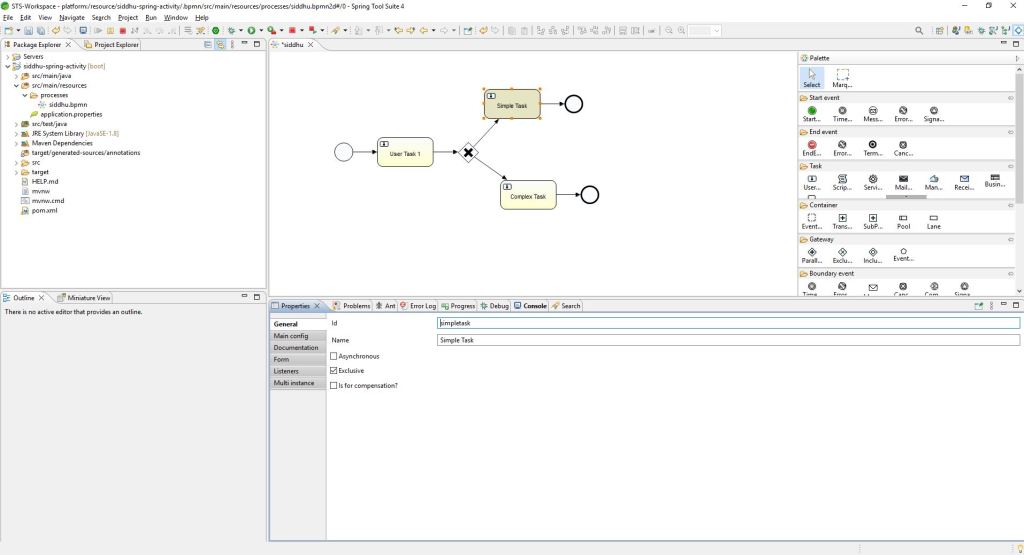



Now lets try to create simple bpmn file and excute that logic using springboot appication.
This bpmn file state
1- User task :- It collect all the task provided to this bpmn and depending on the user/employee assign it will be directed to simple or complex user task.
2- Simple Task :- This user task will contain all the simple task assinged to the perticular user.
3- Complex Task :- This user task will contain all the complex task assinged to the perticular user.
Lets first create simple springboot application using belwo steps.
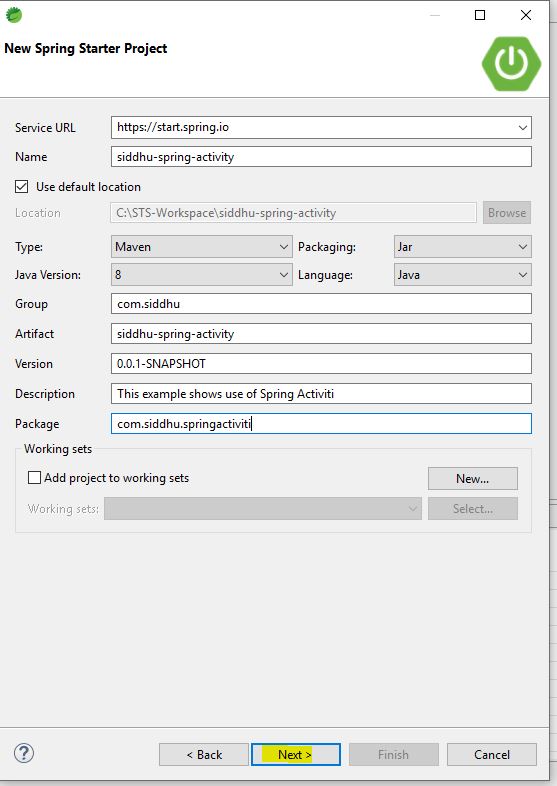
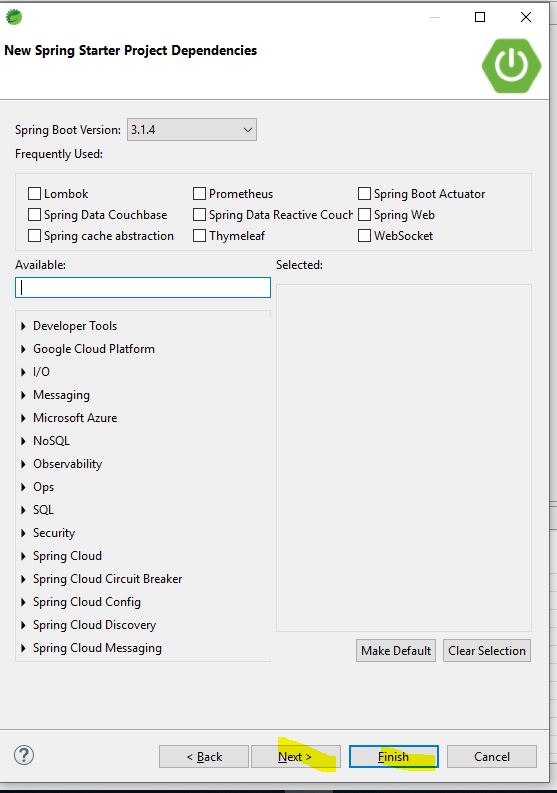
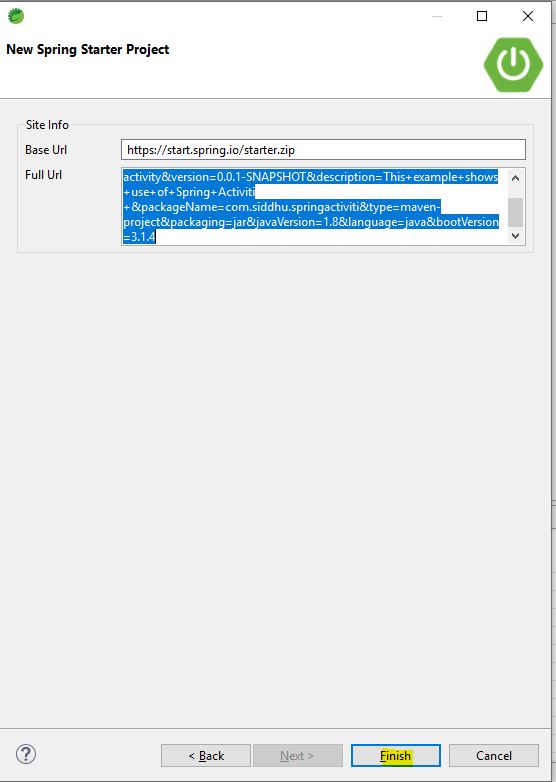
Finally modify our pom.xml add follwing dependecies in it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | <activiti.version>5.22.0</activiti.version> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.activiti</groupId> <artifactId>activiti-spring-boot-starter-basic</artifactId> <version>${activiti.version}</version> </dependency> <dependency> <groupId>org.activiti</groupId> <artifactId>activiti-spring-boot-starter-jpa</artifactId> <version>${activiti.version}</version> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> </dependency> |
Our Pom.xml will be
1- Pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.3.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.siddhu</groupId> <artifactId>siddhu-spring-activity</artifactId> <version>0.0.1-SNAPSHOT</version> <name>siddhu-spring-activity</name> <description>This example shows use of Spring Activiti </description> <properties> <java.version>1.8</java.version> <activiti.version>5.22.0</activiti.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.activiti</groupId> <artifactId>activiti-spring-boot-starter-basic</artifactId> <version>${activiti.version}</version> </dependency> <dependency> <groupId>org.activiti</groupId> <artifactId>activiti-spring-boot-starter-jpa</artifactId> <version>${activiti.version}</version> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project> |
Now lets create our java class.
we have two service
1- EmployeeService:- use to create basic data for using our flow in h2 runtime cache db.
2- ProcessService:- use to start the task, get task details and comlete task so the flow can move forward.
3- ProcessController:- use to navigate the user as per the url hits.
4- SiddhuExecutionListener:- attached to our bmon condition flow. it will decide where to send the user i.e. simpletask or complextask flow depending on the employee data.
So lets start our sprintboot activiti example
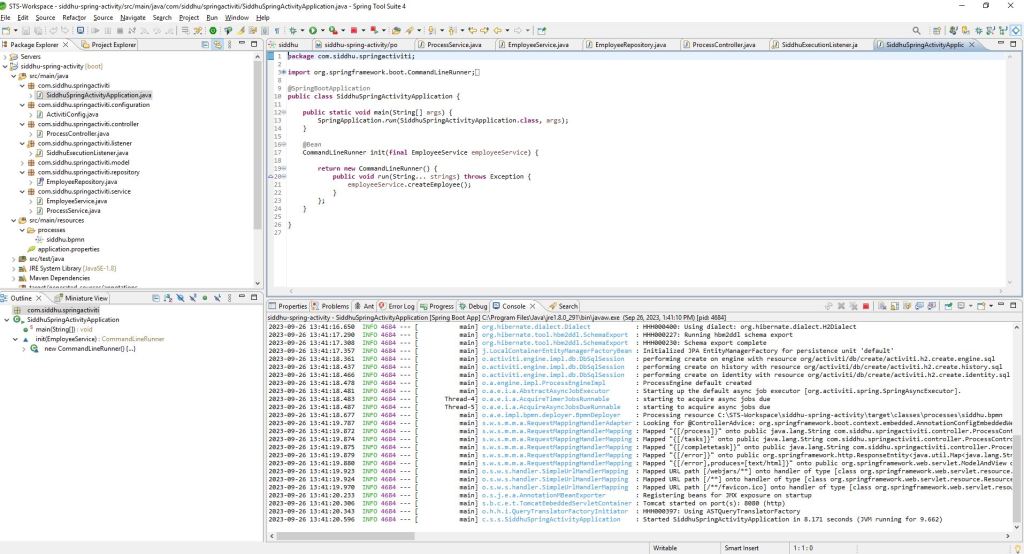
Lets hit the belwo url and you will find this output on the screen.
http://localhost:8080/process?assignee=emp1

our empl1 is having this data
employeeRepository.save(new Employee(“emp1”, “role1”, “simple”));
Number of process definition available: 1 | Task Details= ID: 9, Name: User Task 1, Assignee: emp1, Description: This is user task1
now check at what stage is our task using below url.
http://localhost:8080/tasks?assignee=emp1

[Task[id=9, name=User Task 1]]
here we can see that our employee 1 is at task 1 and his task id is 9.
Now lets complete this task so that our emp1 can move to condition blog using below url.
http://localhost:8080/completetask?taskId=9

Task with id 9 has been completed!
This output shows task 9 assigned to user emp1 is completed and it went to condition blog and as per the logic written in SiddhuExecutionListener and type of task emp1 has i.e. simple it is directed to the simpletask. To check this again hit below url.
http://localhost:8080/tasks?assignee=emp1

Now check the same with our emp2 having comlex task and verify that user is navigated to complex task as show below.




you can download this code from below given github location