One of the best use of GraphQL is its ability to react onspot i.e. as in old JDK we have the concept of Observable Class and Observer Interface where in whenever any observable class change it notify all the observer class to perform some action. Same is done using RXJAVA
package com.siddhugraphql.java.siddhuuserdetails.util;
import com.siddhugraphql.java.siddhuuserdetails.resolvers.User;
import io.reactivex.Observable;
public class RxJava2Example
{
public static void main(String[] args)
{
User obj = new User("One","two",3);
//producer
Observable<User> observable = Observable.just(obj);
//consumer
//Consumer<? super String> consumer = System.out::println;
SiddhuConsumer objSiddhuConsumer = new SiddhuConsumer();
//Attaching producer to consumer
observable.subscribe(objSiddhuConsumer);
}
}
package com.siddhugraphql.java.siddhuuserdetails.util;
import com.siddhugraphql.java.siddhuuserdetails.resolvers.User;
import io.reactivex.functions.Consumer;
public class SiddhuConsumer implements Consumer<User> {
@Override
public void accept(User t) throws Exception {
// TODO Auto-generated method stub
System.out.println("--------------------"+t.toString());
}
}
As shown above for RX we have
Producer :- That produce the data and emit. It use observable class and subscribe the Consumber
Consumer :- That consume the data and work on it. For doing this it implements Consumer interface and override accept() method.
Now lets come to our GraphQL example. Lets say we want to demo an subscription example where in when we do any mutation operaion i.e createUser it will be catch by our consumber and will display it on the screen
Let me show you the screen shot
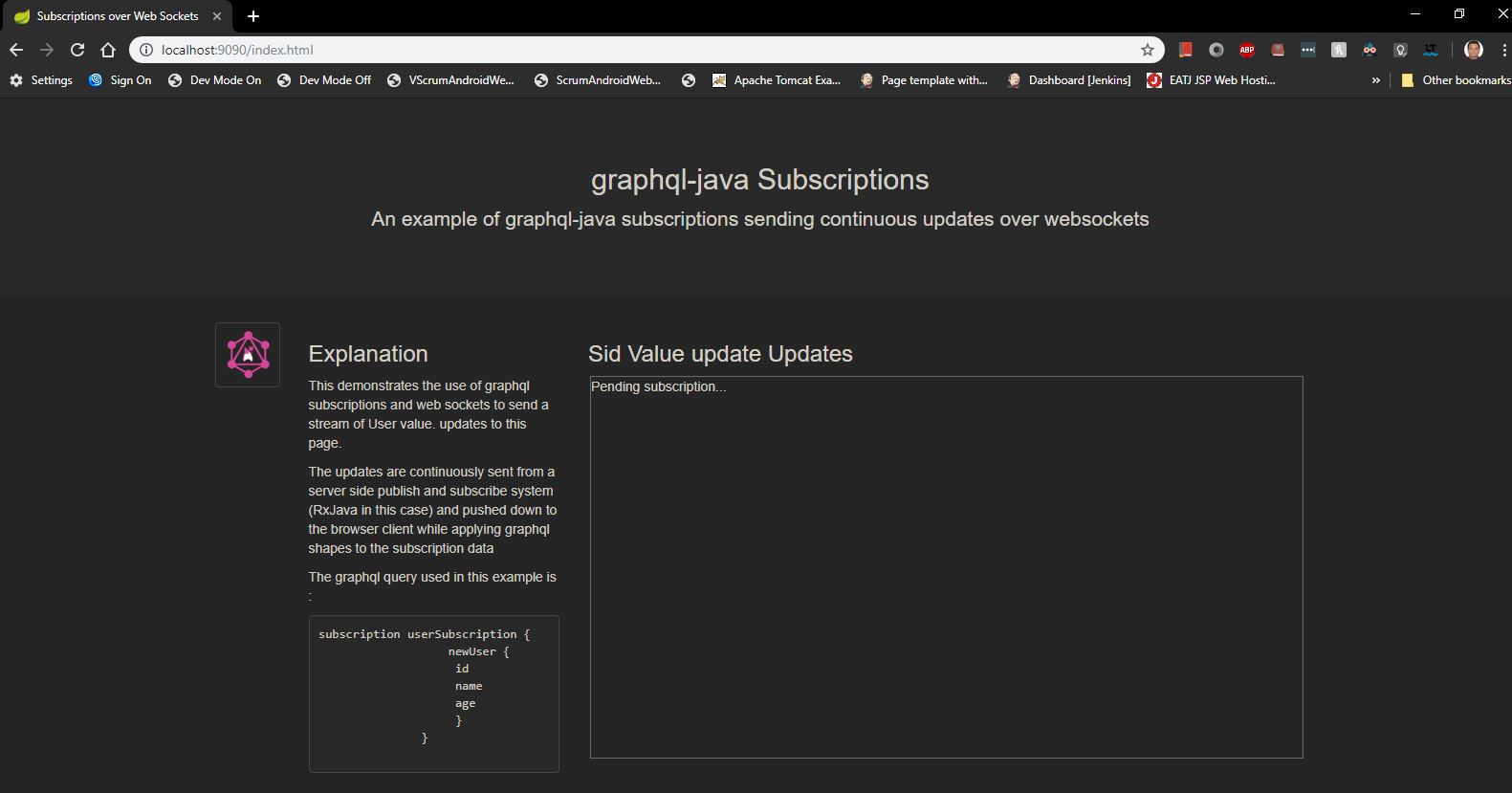
Through Post man we hit the Graph Mutation request.
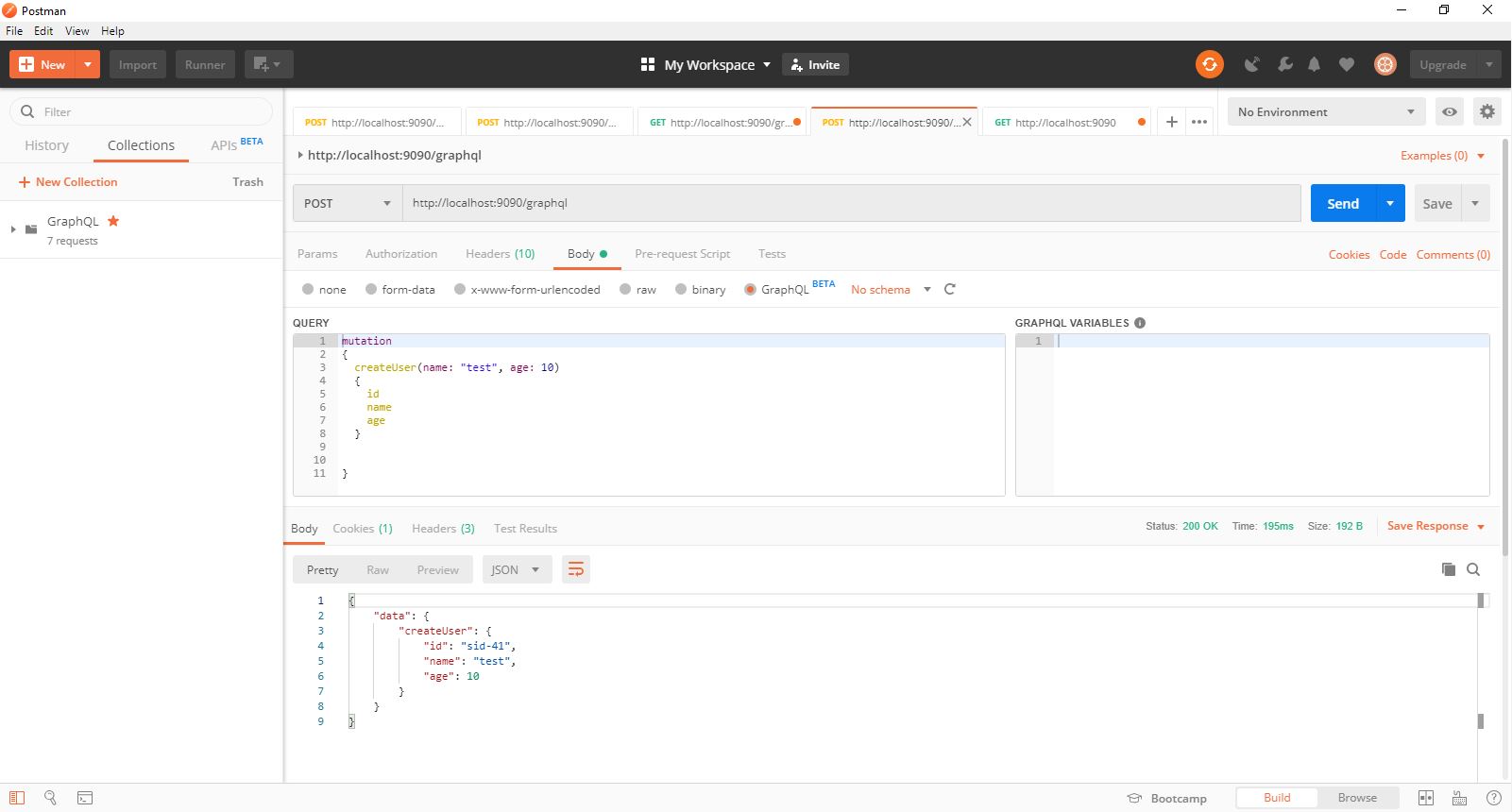
Below image shows that as soon as the mutation request is fired we find the data present in the UI
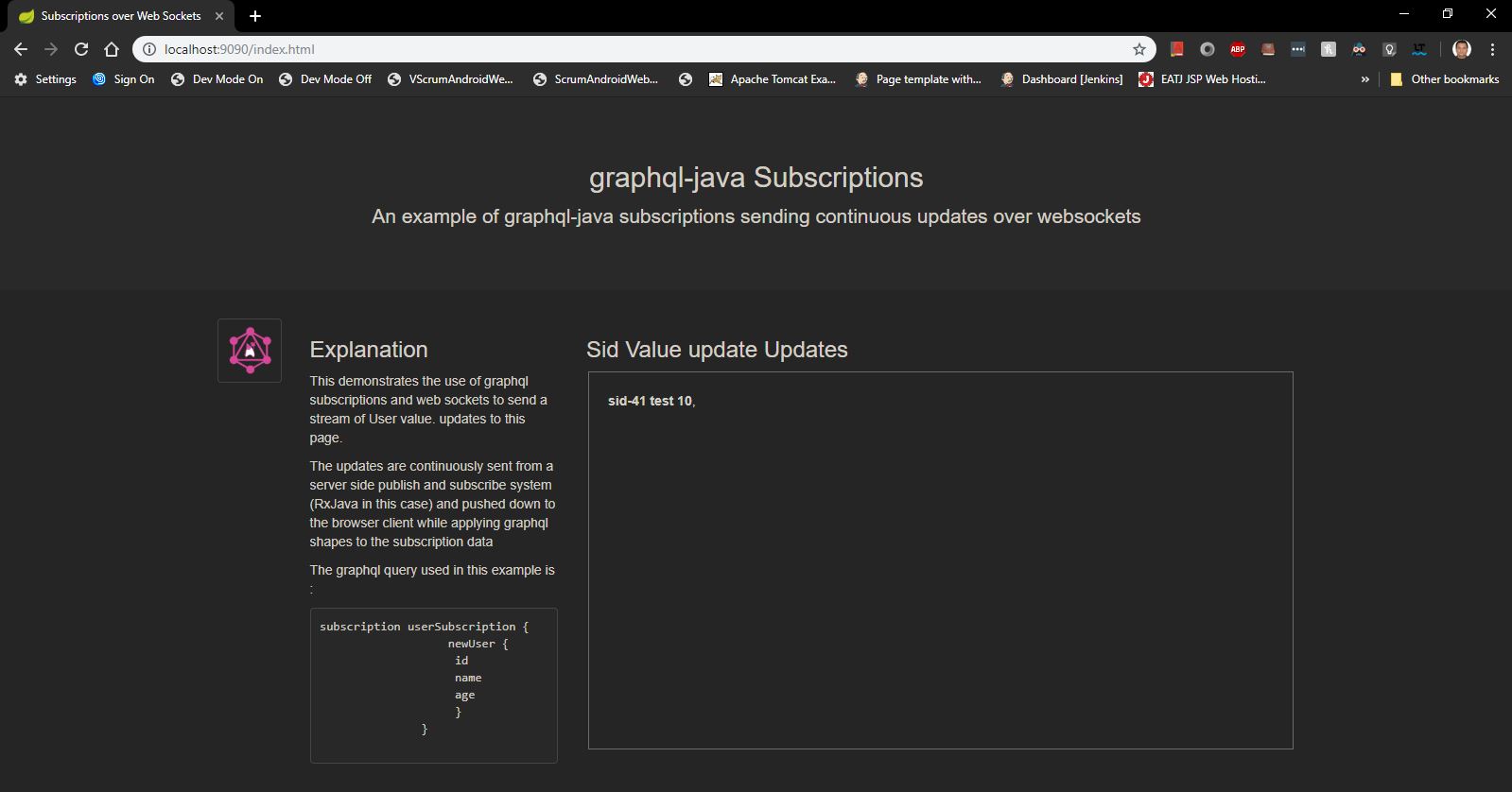
Hurrey we are done with simple GraphQL Subscription example
Now lets go in details of what we had done in code
You can download the complete running example from below GIT location
https://github.com/shdhumale/siddhu-user-details
We have use the inbuild example of graphql Subscrition example html to request subsription request.
1- index.html
Following lines are modified to hit our Subscription request
function subscribeToStocks() {
var exampleSocket = new WebSocket("ws://localhost:9090/stockticker");
networkBlip();
exampleSocket.onopen = function () {
networkBlip();
console.log("web socket opened");
var query = 'subscription userSubscription { \n' +
' newUser {' +
' id\n' +
' name\n' +
' age\n' +
' }' +
'}';
var graphqlMsg = {
query: query,
variables: {}
};
exampleSocket.send(JSON.stringify(graphqlMsg));
};
var STOCK_CODES_UPDATES = {};
exampleSocket.onmessage = function (event) {
networkBlip();
var data = JSON.parse(event.data);
console.log("data----------------->"+data);
var age = data.newUser.age;
var id = data.newUser.id;
var name = data.newUser.name;
console.log("id----------------->"+id);
console.log("age----------------->"+age);
console.log("name----------------->"+name);
var htmlStr = '';
htmlStr += '<div class="stockWrapper">';
htmlStr += '<span class="stockSymbol"> ' + id + ' </span>'
htmlStr += '<span class="stockSymbol"> ' + name + '</span>';
htmlStr += '<span class="stockSymbol"> ' + age + '</span>';
htmlStr += ', ';
htmlStr += '</div>';
$('.stockTicker').html(htmlStr)
};
}
window.addEventListener("load", subscribeToStocks);
First file which is hit when we run this url on the browser http://localhost:9090/index.html is SiddhuWebSocketConfig
From here we execute SiddhuWebSocketHandler and initialize SiddhuGraphqlPublisher.
SiddhuWebSocketHandler class is used to make the websocket connection and listen to that socket if any request is coming
SiddhuGraphqlPublisher class we read our schema.graphqls and execute it so that we can use this code as our Graphql server code to serve the request.
When we execute our SiddhuUserDetailsApplication spring applications
GraphQLProvider execute our schema.graphqls so that it will be available for all the request given by client.
In our mutation call we make a call publish with our User object.
//by siddhu for Mutation start[
public DataFetcher createUserDataFetcher() {
System.out.println("here reached............");
return dataFetchingEnvironment -> {
String name = dataFetchingEnvironment.getArgument("name");
System.out.println("here reached..name.........."+name);
int age = dataFetchingEnvironment.getArgument("age");
System.out.println("here reached..age.........."+""+age);
objUser = new com.siddhugraphql.java.siddhuuserdetails.resolvers.User("sid-41",name,age);
SiddhuGraphqlPublisher.STOCK_TICKER_PUBLISHER.publish(objUser);
return objUser;
};
}
//by siddhu for Mutaion end]
finally in our UserPublisher constructor
public UserPublisher(){
Observable<User> commentUpdateObservable = Observable.create(emitter -> {
this.emitter = emitter;
});
ConnectableObservable<User> connectableObservable = commentUpdateObservable.share().publish();
connectableObservable.connect();
publisher = connectableObservable.toFlowable(BackpressureStrategy.BUFFER);
}
public Flowable<User> getPublisher() {
return publisher;
}
public void publish(final User user) {
emitter.onNext(user);
}
that's it and you will be able to see our subscription example is running with simple java code.
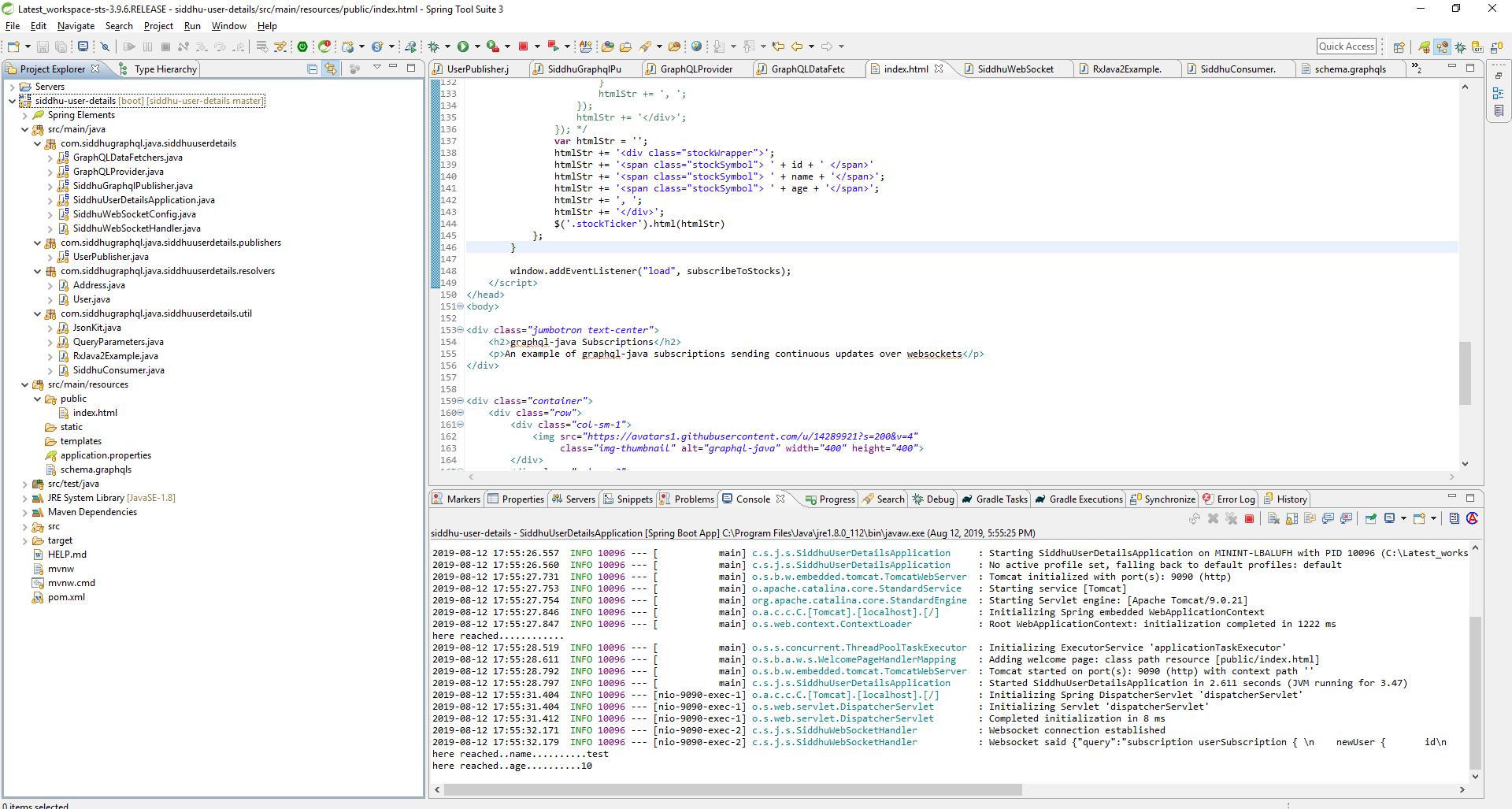
package com.siddhugraphql.java.siddhuuserdetails.util;
import com.siddhugraphql.java.siddhuuserdetails.resolvers.User;
import io.reactivex.Observable;
public class RxJava2Example
{
public static void main(String[] args)
{
User obj = new User("One","two",3);
//producer
Observable<User> observable = Observable.just(obj);
//consumer
//Consumer<? super String> consumer = System.out::println;
SiddhuConsumer objSiddhuConsumer = new SiddhuConsumer();
//Attaching producer to consumer
observable.subscribe(objSiddhuConsumer);
}
}
package com.siddhugraphql.java.siddhuuserdetails.util;
import com.siddhugraphql.java.siddhuuserdetails.resolvers.User;
import io.reactivex.functions.Consumer;
public class SiddhuConsumer implements Consumer<User> {
@Override
public void accept(User t) throws Exception {
// TODO Auto-generated method stub
System.out.println("--------------------"+t.toString());
}
}
As shown above for RX we have
Producer :- That produce the data and emit. It use observable class and subscribe the Consumber
Consumer :- That consume the data and work on it. For doing this it implements Consumer interface and override accept() method.
Now lets come to our GraphQL example. Lets say we want to demo an subscription example where in when we do any mutation operaion i.e createUser it will be catch by our consumber and will display it on the screen
Let me show you the screen shot
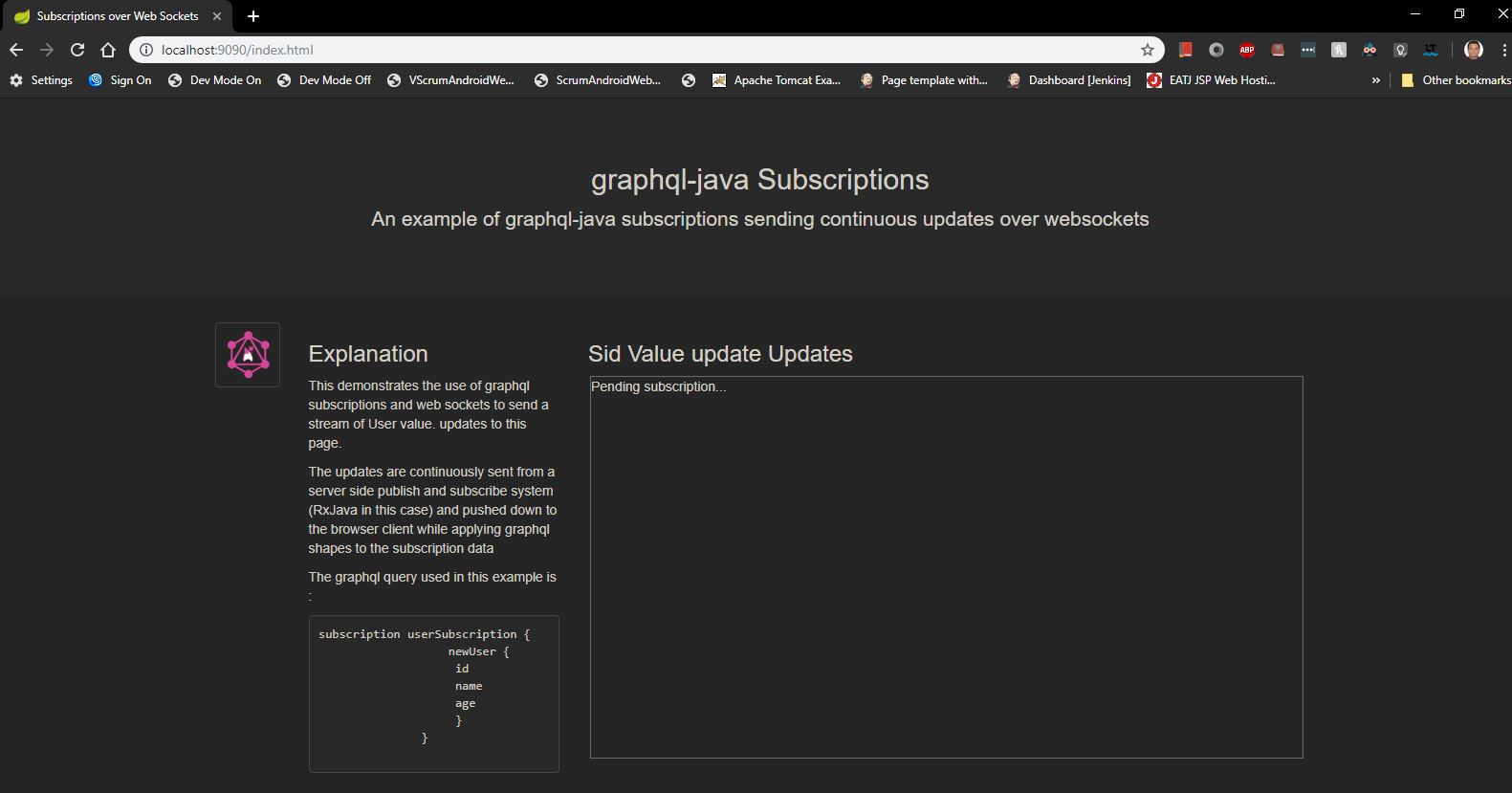
Through Post man we hit the Graph Mutation request.
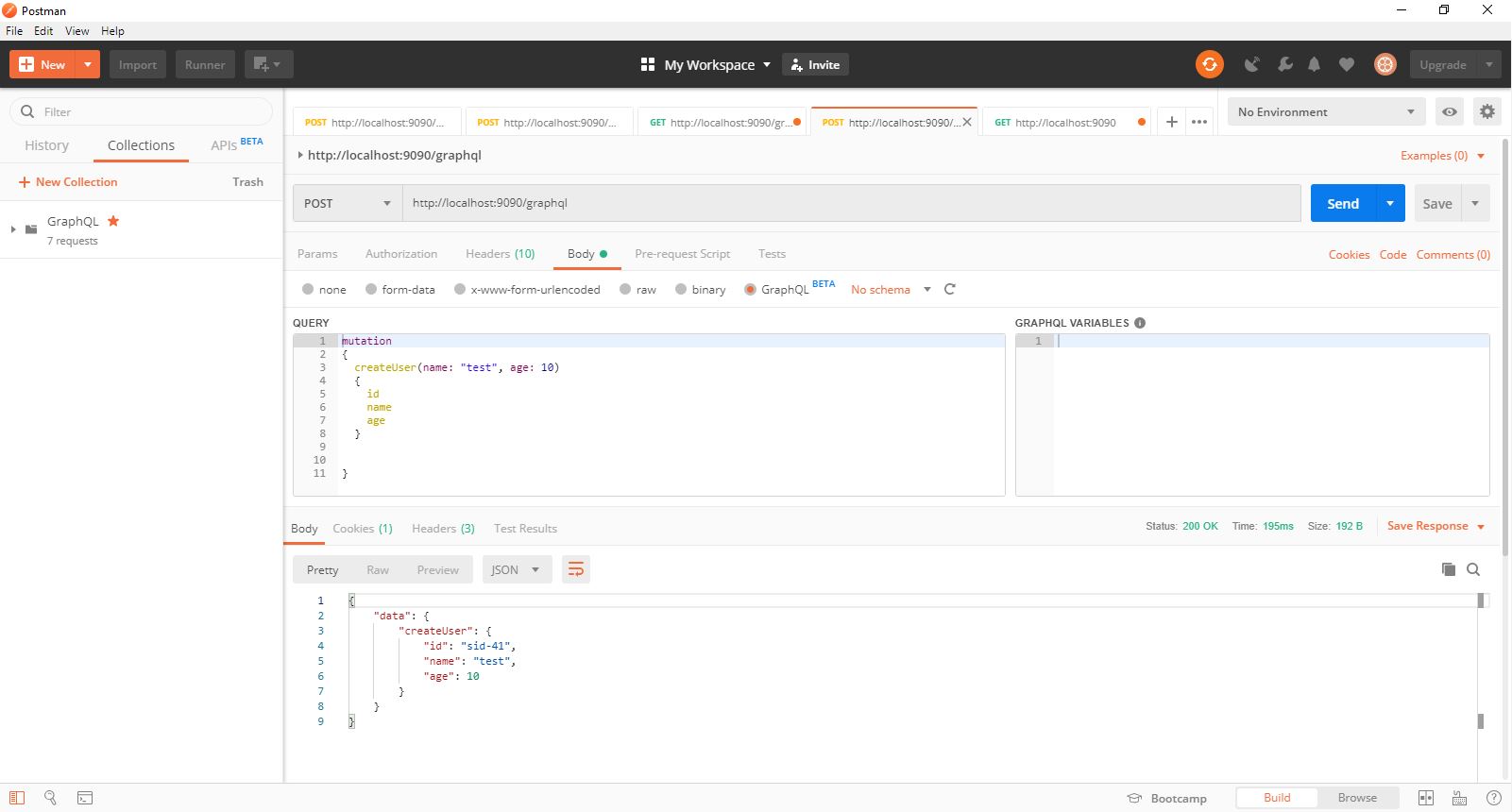
Below image shows that as soon as the mutation request is fired we find the data present in the UI
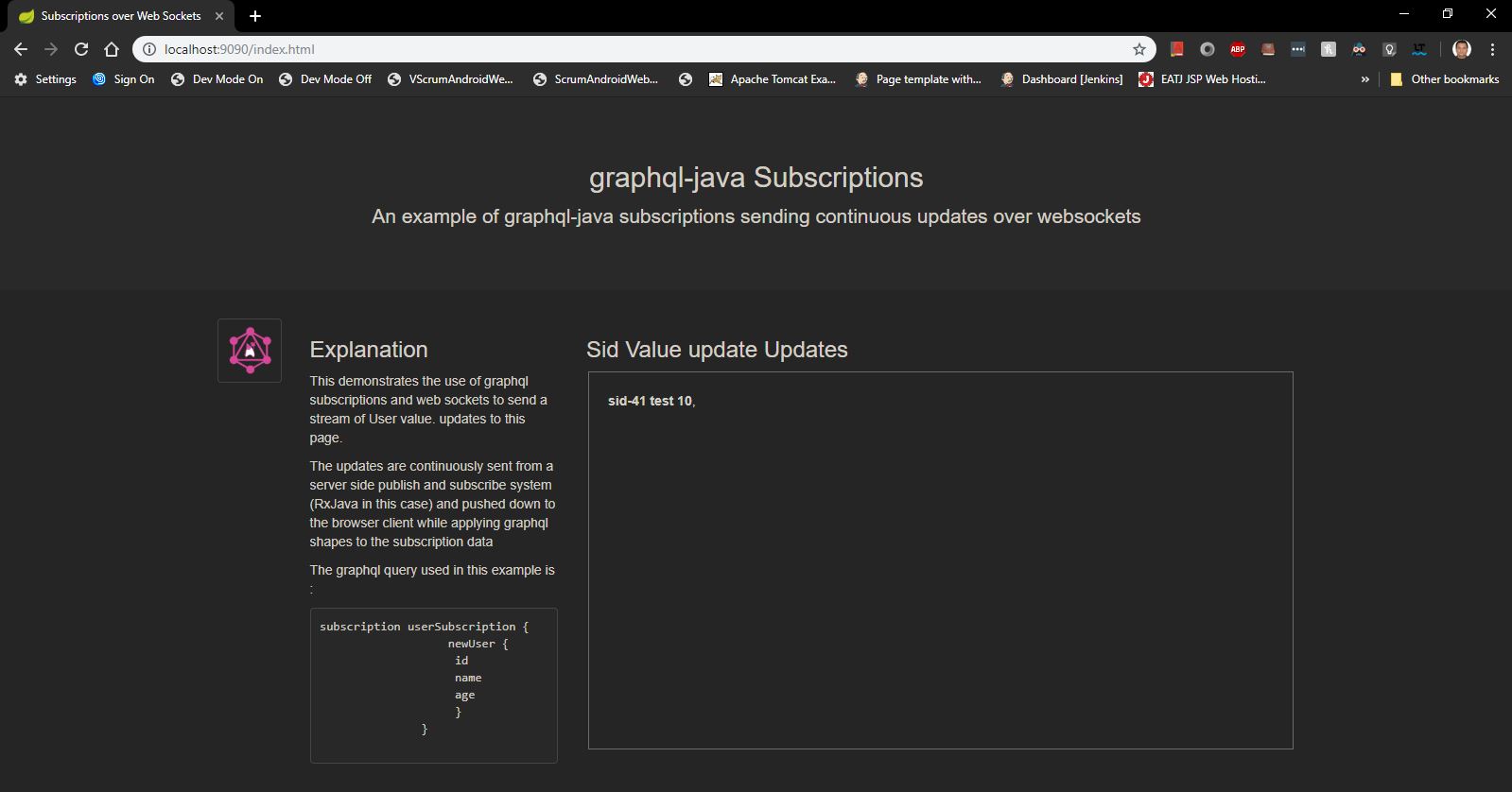
Hurrey we are done with simple GraphQL Subscription example
Now lets go in details of what we had done in code
You can download the complete running example from below GIT location
https://github.com/shdhumale/siddhu-user-details
We have use the inbuild example of graphql Subscrition example html to request subsription request.
1- index.html
Following lines are modified to hit our Subscription request
function subscribeToStocks() {
var exampleSocket = new WebSocket("ws://localhost:9090/stockticker");
networkBlip();
exampleSocket.onopen = function () {
networkBlip();
console.log("web socket opened");
var query = 'subscription userSubscription { \n' +
' newUser {' +
' id\n' +
' name\n' +
' age\n' +
' }' +
'}';
var graphqlMsg = {
query: query,
variables: {}
};
exampleSocket.send(JSON.stringify(graphqlMsg));
};
var STOCK_CODES_UPDATES = {};
exampleSocket.onmessage = function (event) {
networkBlip();
var data = JSON.parse(event.data);
console.log("data----------------->"+data);
var age = data.newUser.age;
var id = data.newUser.id;
var name = data.newUser.name;
console.log("id----------------->"+id);
console.log("age----------------->"+age);
console.log("name----------------->"+name);
var htmlStr = '';
htmlStr += '<div class="stockWrapper">';
htmlStr += '<span class="stockSymbol"> ' + id + ' </span>'
htmlStr += '<span class="stockSymbol"> ' + name + '</span>';
htmlStr += '<span class="stockSymbol"> ' + age + '</span>';
htmlStr += ', ';
htmlStr += '</div>';
$('.stockTicker').html(htmlStr)
};
}
window.addEventListener("load", subscribeToStocks);
First file which is hit when we run this url on the browser http://localhost:9090/index.html is SiddhuWebSocketConfig
From here we execute SiddhuWebSocketHandler and initialize SiddhuGraphqlPublisher.
SiddhuWebSocketHandler class is used to make the websocket connection and listen to that socket if any request is coming
SiddhuGraphqlPublisher class we read our schema.graphqls and execute it so that we can use this code as our Graphql server code to serve the request.
When we execute our SiddhuUserDetailsApplication spring applications
GraphQLProvider execute our schema.graphqls so that it will be available for all the request given by client.
In our mutation call we make a call publish with our User object.
//by siddhu for Mutation start[
public DataFetcher createUserDataFetcher() {
System.out.println("here reached............");
return dataFetchingEnvironment -> {
String name = dataFetchingEnvironment.getArgument("name");
System.out.println("here reached..name.........."+name);
int age = dataFetchingEnvironment.getArgument("age");
System.out.println("here reached..age.........."+""+age);
objUser = new com.siddhugraphql.java.siddhuuserdetails.resolvers.User("sid-41",name,age);
SiddhuGraphqlPublisher.STOCK_TICKER_PUBLISHER.publish(objUser);
return objUser;
};
}
//by siddhu for Mutaion end]
finally in our UserPublisher constructor
public UserPublisher(){
Observable<User> commentUpdateObservable = Observable.create(emitter -> {
this.emitter = emitter;
});
ConnectableObservable<User> connectableObservable = commentUpdateObservable.share().publish();
connectableObservable.connect();
publisher = connectableObservable.toFlowable(BackpressureStrategy.BUFFER);
}
public Flowable<User> getPublisher() {
return publisher;
}
public void publish(final User user) {
emitter.onNext(user);
}
that's it and you will be able to see our subscription example is running with simple java code.
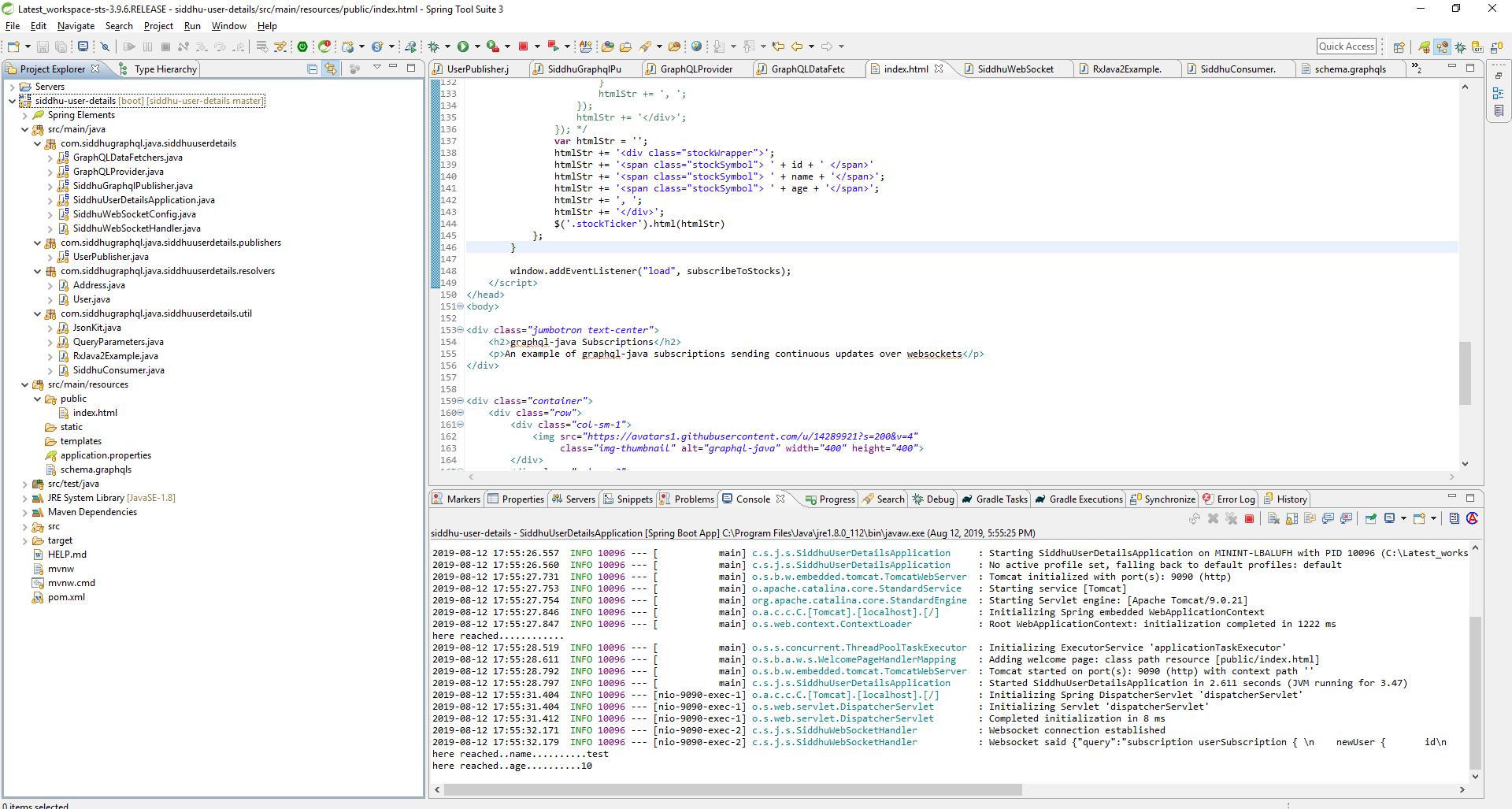