Step 1:- Down load FTP Server from below link
https://projects.apache.org/project.html?mina-ftpserver
Step 2:- You can start the using following command
C:\apache-ftpserver-1.1.1\apache-ftpserver-1.1.1\bin>ftpd.bat
Using default configuration
FtpServer started
Stopping server...
Step 3:- We are using java code to start the server at perticular port and then use below java code to do upload and download functionality
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import org.apache.commons.net.PrintCommandListener;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import org.apache.commons.net.ftp.FTPReply;
import org.apache.ftpserver.FtpServer;
import org.apache.ftpserver.FtpServerFactory;
import org.apache.ftpserver.listener.ListenerFactory;
import org.apache.ftpserver.usermanager.PropertiesUserManagerFactory;
public class FTPFunctions {
// Creating FTP Client instance
FTPClient ftp = null;
public FTPFunctions()
{
}
public void StartServer()
{
try {
FtpServerFactory serverFactory = new FtpServerFactory();
ListenerFactory factory = new ListenerFactory();
// set the port of the listener
factory.setPort(21);
// define SSL configuration
/*SslConfigurationFactory ssl = new SslConfigurationFactory();
ssl.setKeystoreFile(new File("C:\\Test\\ftpserver.jks"));
ssl.setKeystorePassword("password");*/
// set the SSL configuration for the listener
/*factory.setSslConfiguration(ssl.createSslConfiguration());
factory.setImplicitSsl(true);*/
// replace the default listener
serverFactory.addListener("default", factory.createListener());
PropertiesUserManagerFactory userManagerFactory = new PropertiesUserManagerFactory();
userManagerFactory.setFile(new File("C:\\Test\\users.properties"));
serverFactory.setUserManager(userManagerFactory.createUserManager());
// start the server
FtpServer server = serverFactory.createServer();
server.start();
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println("Exception occured ---------------------");
e.printStackTrace();
}
}
// Constructor to connect to the FTP Server
/**
* @param host
* @param port
* @param username
* @param password
* @throws Exception
*/
public FTPFunctions(String host, int port, String username, String password) throws Exception{
ftp = new FTPClient();
ftp.addProtocolCommandListener(new PrintCommandListener(new PrintWriter(System.out)));
int reply;
ftp.connect(host,port);
System.out.println("FTP URL is:"+ftp.getDefaultPort());
reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
ftp.disconnect();
throw new Exception("Exception in connecting to FTP Server");
}
ftp.login(username, password);
ftp.setFileType(FTP.LOCAL_FILE_TYPE);
ftp.enterLocalPassiveMode();
}
// Method to upload the File on the FTP Server
public void uploadFTPFile(String localFileFullName, String fileName, String uploadDir)
throws Exception
{
try {
InputStream input = new FileInputStream(new File(localFileFullName));
this.ftp.storeFile(uploadDir + fileName, input);
}
catch(Exception e){
}
}
// Download the FTP File from the FTP Server
public void downloadFTPFile(String ftpSource, String destination) {
try (FileOutputStream fos = new FileOutputStream(destination)) {
this.ftp.retrieveFile(ftpSource, fos);
} catch (IOException e) {
e.printStackTrace();
}
}
// list the files in a specified directory on the FTP
public boolean listFTPFiles(String directory, String fileName) throws IOException {
// lists files and directories in the current working directory
boolean verificationFilename = false;
FTPFile[] files = ftp.listFiles(directory);
for (FTPFile file : files) {
String details = file.getName();
System.out.println(details);
if(details.equals(fileName))
{
System.out.println("Correct Filename");
verificationFilename=details.equals(fileName);
//assertTrue("Verification Failed: The filename is not updated at the CDN end.",details.equals(fileName));
}
}
return verificationFilename;
}
// Disconnect the connection to FTP
public void disconnect(){
if (this.ftp.isConnected()) {
try {
this.ftp.logout();
this.ftp.disconnect();
} catch (IOException f) {
// do nothing as file is already saved to server
}
}
}
// Main method to invoke the above methods
public static void main(String[] args) {
try {
FTPFunctions objFTPFunctions = new FTPFunctions();
objFTPFunctions.StartServer();
FTPFunctions ftpobj = new FTPFunctions("127.0.0.1", 21, "admin", "admin");
ftpobj.uploadFTPFile("C:\\Test\\siddhu.txt", "siddhuupload.txt", "/uploadftp/");
ftpobj.downloadFTPFile("/uploadftp/siddhuupload.txt","C:\\Test_New\\downloadftp\\siddhudownload.txt");
System.out.println("FTP File downloaded successfully");
//boolean result = ftpobj.listFTPFiles("C:\\Test_New\\downloadftp", "siddhu.txt");
//System.out.println(result);
ftpobj.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
We are starting the FTP server on localhost with 21 port and user id and password as admin admin.
Make sure you take users.properties from you C:\apache-ftpserver-1.1.1\apache-ftpserver-1.1.1\res\conf folder and place it on perticular location. This files we are using for login.
By defualt password of admin is admin
Note:First run the java program and then try to connect using FileZilla tool.

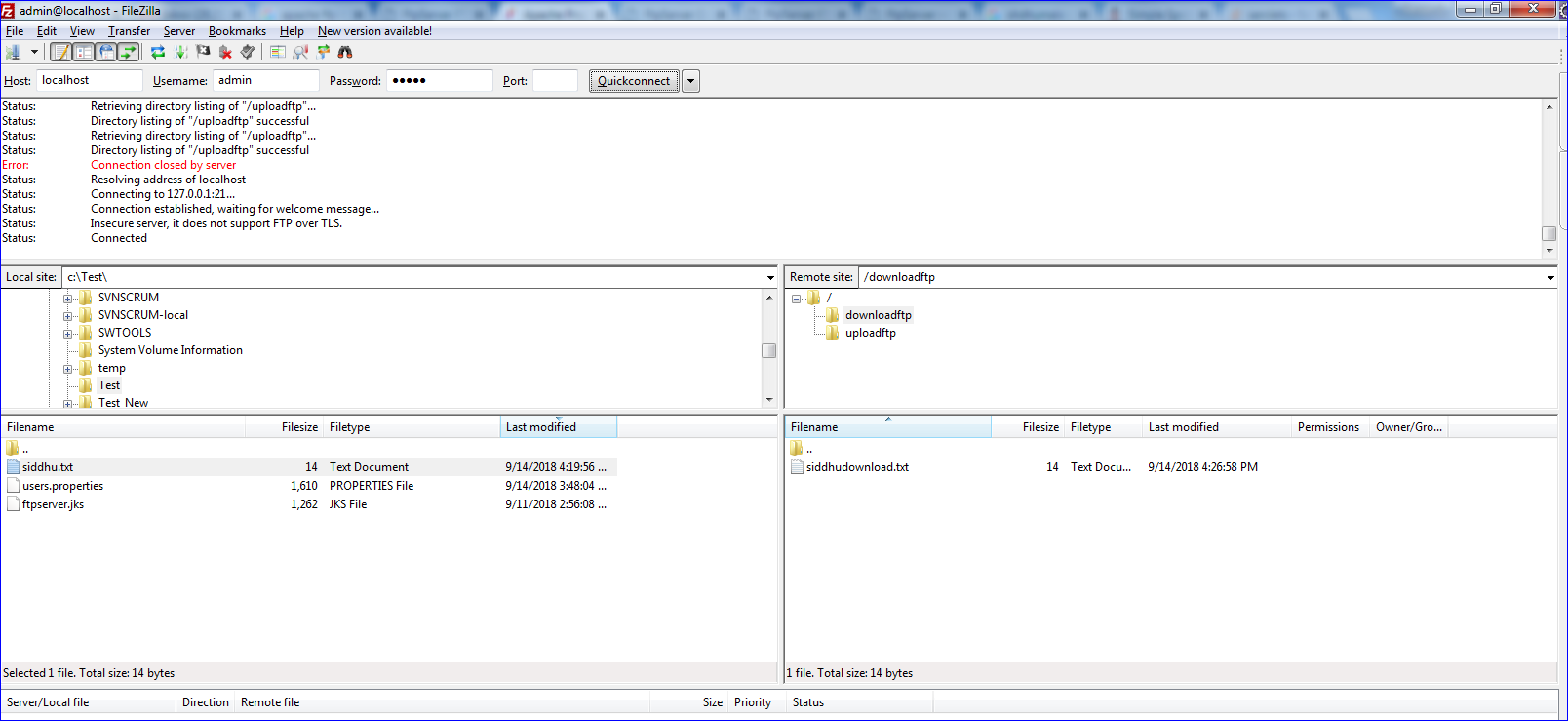

https://projects.apache.org/project.html?mina-ftpserver
Step 2:- You can start the using following command
C:\apache-ftpserver-1.1.1\apache-ftpserver-1.1.1\bin>ftpd.bat
Using default configuration
FtpServer started
Stopping server...
Step 3:- We are using java code to start the server at perticular port and then use below java code to do upload and download functionality
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import org.apache.commons.net.PrintCommandListener;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import org.apache.commons.net.ftp.FTPReply;
import org.apache.ftpserver.FtpServer;
import org.apache.ftpserver.FtpServerFactory;
import org.apache.ftpserver.listener.ListenerFactory;
import org.apache.ftpserver.usermanager.PropertiesUserManagerFactory;
public class FTPFunctions {
// Creating FTP Client instance
FTPClient ftp = null;
public FTPFunctions()
{
}
public void StartServer()
{
try {
FtpServerFactory serverFactory = new FtpServerFactory();
ListenerFactory factory = new ListenerFactory();
// set the port of the listener
factory.setPort(21);
// define SSL configuration
/*SslConfigurationFactory ssl = new SslConfigurationFactory();
ssl.setKeystoreFile(new File("C:\\Test\\ftpserver.jks"));
ssl.setKeystorePassword("password");*/
// set the SSL configuration for the listener
/*factory.setSslConfiguration(ssl.createSslConfiguration());
factory.setImplicitSsl(true);*/
// replace the default listener
serverFactory.addListener("default", factory.createListener());
PropertiesUserManagerFactory userManagerFactory = new PropertiesUserManagerFactory();
userManagerFactory.setFile(new File("C:\\Test\\users.properties"));
serverFactory.setUserManager(userManagerFactory.createUserManager());
// start the server
FtpServer server = serverFactory.createServer();
server.start();
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println("Exception occured ---------------------");
e.printStackTrace();
}
}
// Constructor to connect to the FTP Server
/**
* @param host
* @param port
* @param username
* @param password
* @throws Exception
*/
public FTPFunctions(String host, int port, String username, String password) throws Exception{
ftp = new FTPClient();
ftp.addProtocolCommandListener(new PrintCommandListener(new PrintWriter(System.out)));
int reply;
ftp.connect(host,port);
System.out.println("FTP URL is:"+ftp.getDefaultPort());
reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
ftp.disconnect();
throw new Exception("Exception in connecting to FTP Server");
}
ftp.login(username, password);
ftp.setFileType(FTP.LOCAL_FILE_TYPE);
ftp.enterLocalPassiveMode();
}
// Method to upload the File on the FTP Server
public void uploadFTPFile(String localFileFullName, String fileName, String uploadDir)
throws Exception
{
try {
InputStream input = new FileInputStream(new File(localFileFullName));
this.ftp.storeFile(uploadDir + fileName, input);
}
catch(Exception e){
}
}
// Download the FTP File from the FTP Server
public void downloadFTPFile(String ftpSource, String destination) {
try (FileOutputStream fos = new FileOutputStream(destination)) {
this.ftp.retrieveFile(ftpSource, fos);
} catch (IOException e) {
e.printStackTrace();
}
}
// list the files in a specified directory on the FTP
public boolean listFTPFiles(String directory, String fileName) throws IOException {
// lists files and directories in the current working directory
boolean verificationFilename = false;
FTPFile[] files = ftp.listFiles(directory);
for (FTPFile file : files) {
String details = file.getName();
System.out.println(details);
if(details.equals(fileName))
{
System.out.println("Correct Filename");
verificationFilename=details.equals(fileName);
//assertTrue("Verification Failed: The filename is not updated at the CDN end.",details.equals(fileName));
}
}
return verificationFilename;
}
// Disconnect the connection to FTP
public void disconnect(){
if (this.ftp.isConnected()) {
try {
this.ftp.logout();
this.ftp.disconnect();
} catch (IOException f) {
// do nothing as file is already saved to server
}
}
}
// Main method to invoke the above methods
public static void main(String[] args) {
try {
FTPFunctions objFTPFunctions = new FTPFunctions();
objFTPFunctions.StartServer();
FTPFunctions ftpobj = new FTPFunctions("127.0.0.1", 21, "admin", "admin");
ftpobj.uploadFTPFile("C:\\Test\\siddhu.txt", "siddhuupload.txt", "/uploadftp/");
ftpobj.downloadFTPFile("/uploadftp/siddhuupload.txt","C:\\Test_New\\downloadftp\\siddhudownload.txt");
System.out.println("FTP File downloaded successfully");
//boolean result = ftpobj.listFTPFiles("C:\\Test_New\\downloadftp", "siddhu.txt");
//System.out.println(result);
ftpobj.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
We are starting the FTP server on localhost with 21 port and user id and password as admin admin.
Make sure you take users.properties from you C:\apache-ftpserver-1.1.1\apache-ftpserver-1.1.1\res\conf folder and place it on perticular location. This files we are using for login.
By defualt password of admin is admin
Note:First run the java program and then try to connect using FileZilla tool.

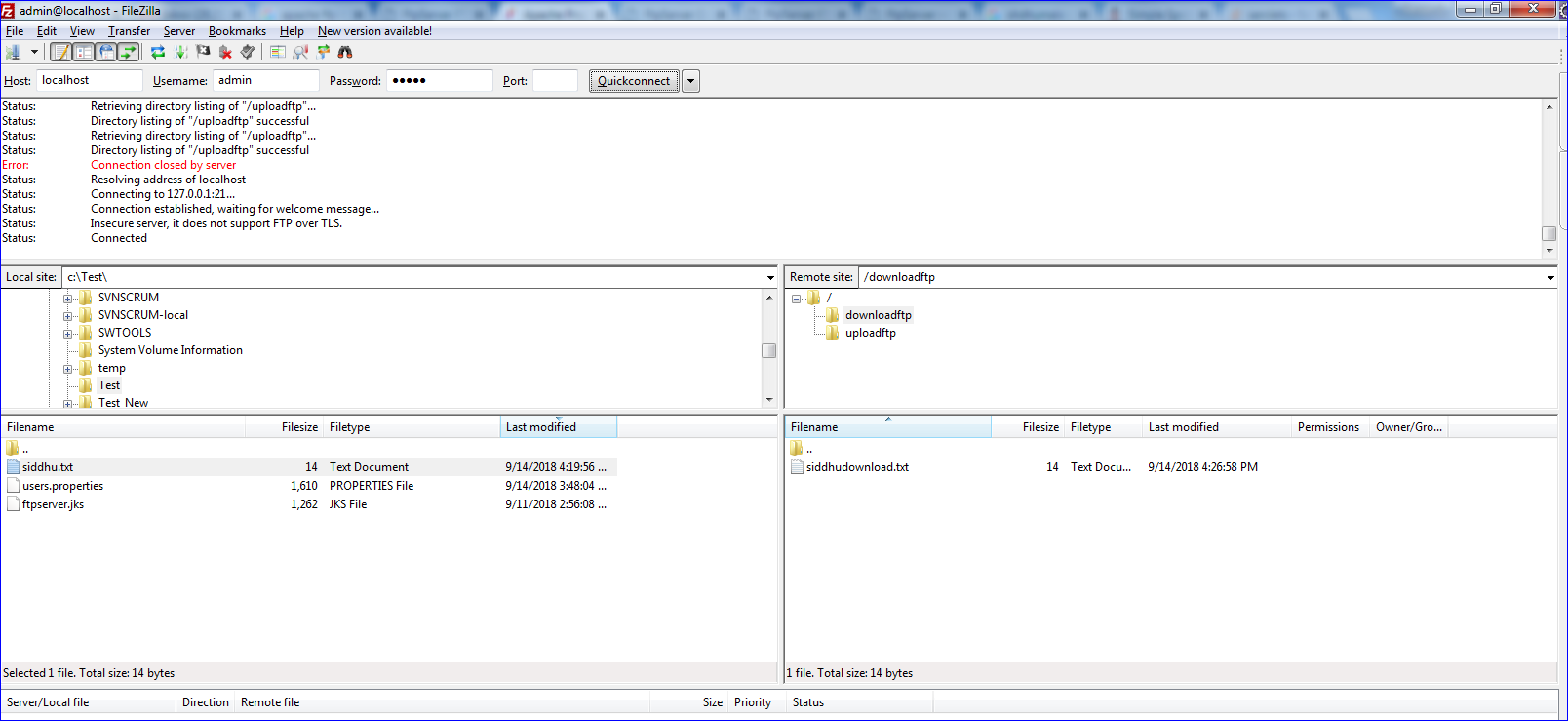

Also make sure that we need to map our local folder for FTP process as given below
ftpserver.user.admin.homedirectory=C:\\Test_New