1- Add following code to your onCreate Method:-
ArrayAdapter adapter = new ArrayAdapter(this, R.layout.activity_listview, mobileArray);
final FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
Animation makeInAnimation = AnimationUtils.makeInAnimation(getBaseContext(), false);
makeInAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationEnd(Animation animation) { }
final FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
Animation makeInAnimation = AnimationUtils.makeInAnimation(getBaseContext(), false);
makeInAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationEnd(Animation animation) { }
@Override
public void onAnimationRepeat(Animation animation) { }
public void onAnimationRepeat(Animation animation) { }
@Override
public void onAnimationStart(Animation animation) {
fab.setVisibility(View.VISIBLE);
}
});
public void onAnimationStart(Animation animation) {
fab.setVisibility(View.VISIBLE);
}
});
Animation makeOutAnimation = AnimationUtils.makeOutAnimation(getBaseContext(), true);
makeOutAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationEnd(Animation animation) {
fab.setVisibility(View.INVISIBLE);
}
makeOutAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationEnd(Animation animation) {
fab.setVisibility(View.INVISIBLE);
}
@Override
public void onAnimationRepeat(Animation animation) { }
public void onAnimationRepeat(Animation animation) { }
@Override
public void onAnimationStart(Animation animation) { }
});
public void onAnimationStart(Animation animation) { }
});
// ...
if (fab.isShown()) {
fab.startAnimation(makeOutAnimation);
}
fab.startAnimation(makeOutAnimation);
}
if (!fab.isShown()) {
fab.startAnimation(makeInAnimation);
}
fab.startAnimation(makeInAnimation);
}
ListView listView = (ListView) findViewById(R.id.mobile_list);
listView.setAdapter(adapter);
listView.setOnScrollListener(new AbsListView.OnScrollListener() {
@Override
public void onScrollStateChanged(AbsListView absListView, int i) {
fab.show();
}
listView.setAdapter(adapter);
listView.setOnScrollListener(new AbsListView.OnScrollListener() {
@Override
public void onScrollStateChanged(AbsListView absListView, int i) {
fab.show();
}
@Override
public void onScroll(AbsListView absListView, int i, int i1, int i2) {
fab.hide();
}
});
2- Add follwing line in xml to get tag
public void onScroll(AbsListView absListView, int i, int i1, int i2) {
fab.hide();
}
});
2- Add follwing line in xml to get tag
android:layout_width="match_parent"
android:layout_height="wrap_content" >
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|right"
android:layout_margin="16dp"
android:src="@android:drawable/ic_dialog_email"
app:layout_anchor="@id/mobile_list"
app:layout_anchorGravity="bottom|right|end" />
3- Activity_list.xml view
android:id="@+id/label"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:padding="10dip"
android:textSize="16dip"
android:textStyle="bold" >
Default

OnScroll

After Scroll
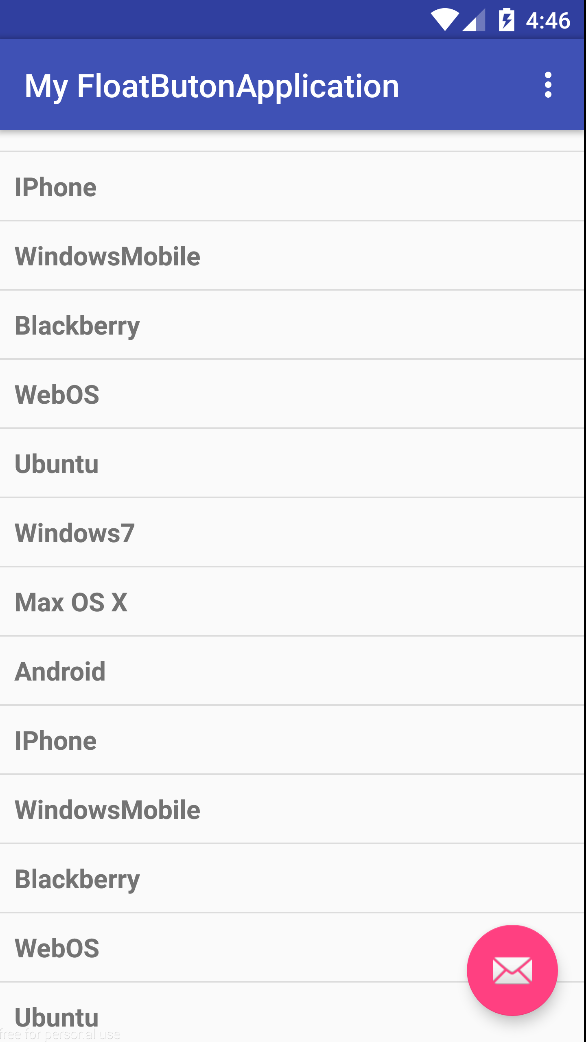