React Java script build by facebook for view part of the MVC. This means it itself did not give or suggest you any frame work to incorporate like Angular which comes with inbuild Framework to build an application. Having said this it becomes important to have a fram work to follow religiously so that project maintainability and scalability can be addressed in long term goal. As in long time after working with any application if no frame work is maintained it will be the main cause of failure along with hard to maintain and understand the project.
Flux is another open source Frame work provided by Facebook and suggested to use in React. As we have MVC framework in java application explained below
Controller :- This is the central hub of the MVC framework. It acts as an intermediate between model and view. Generally we always have one controller per application. Its main function is to handle all the request from the view in terms of action. Controller decide what to do with data either to send it to view part for showing to the user or it need to go to model to perform some DB or REST call.
View :- This represent the view part of the application and never talk directly with Model. It has to go through controller.
Model :- This represent the data that is coming from Controller. It never talk directly with View. It either take data from Controller and perform W/S or DB call or take the data from DB or REST and give to controller by informing to provide it to view for rendering.
Action --> Controller
| |
View Model
Flux is also similar to MVC but unidirectional. It has following part
Action --> Dispatcher --> Store -->View
Lets discuss few of the aspect of all describe above
'- Action send the action using dispatch to dispatcher
'- Dispacther take the action and send the data and action type to store
'- store responds to the dispatched action using register, switch and case.
'- Store finally emits change to view and view update as per the need.
In short user perform the action i.e. clicking on button this action is send to Action class which call the Dispacther class by sending the action type (to differentiate different action) and data and then dispatcher using dispatch this action and data to all the store who has already register them self with this dispatcher. Finally when the data is taken by the store class it perform the operation and send event to View to do changes.
Please refer to the working code as given below
You can also download the code from URL
https://github.com/shdhumale/SimpleReactFluxExample
Folder structure
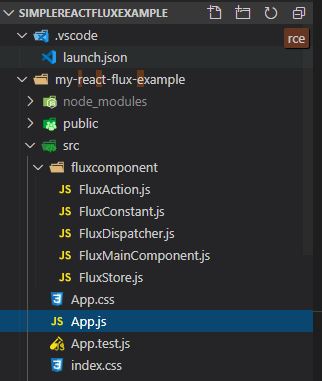
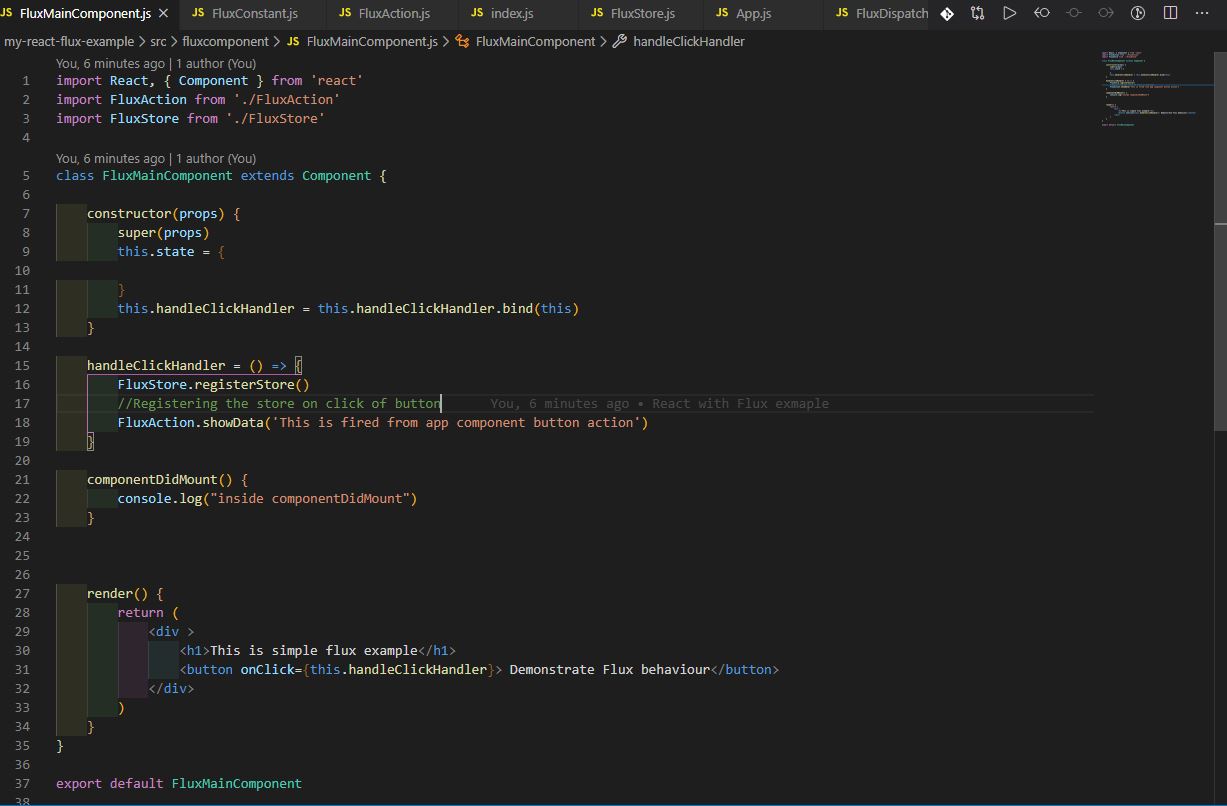


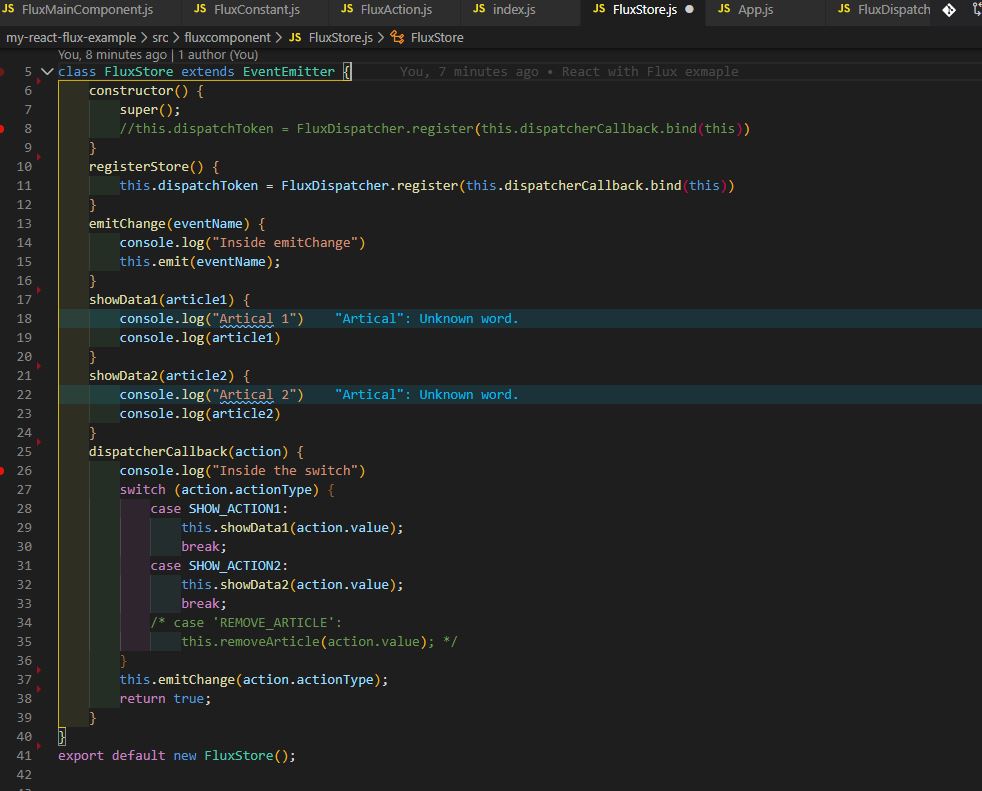


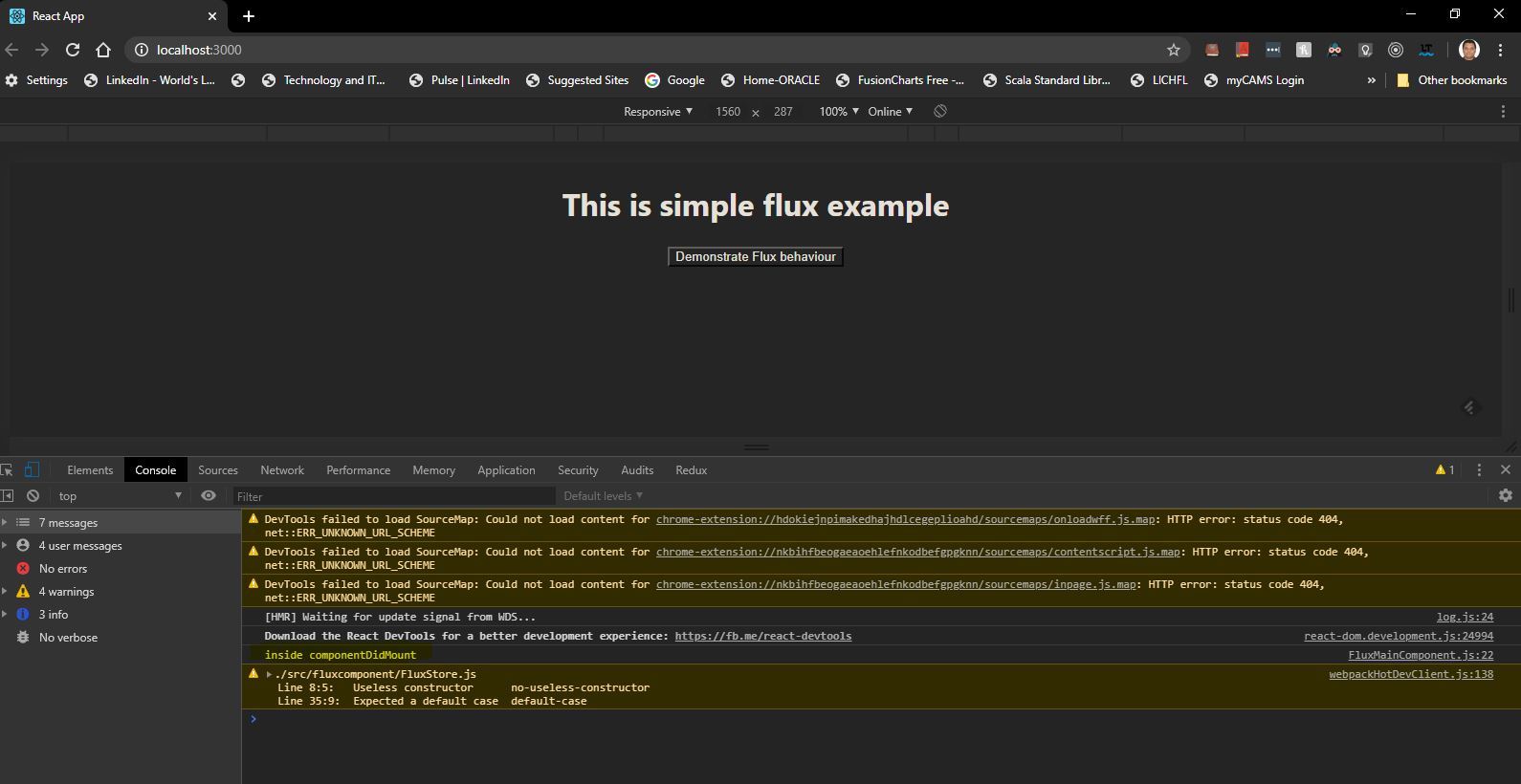
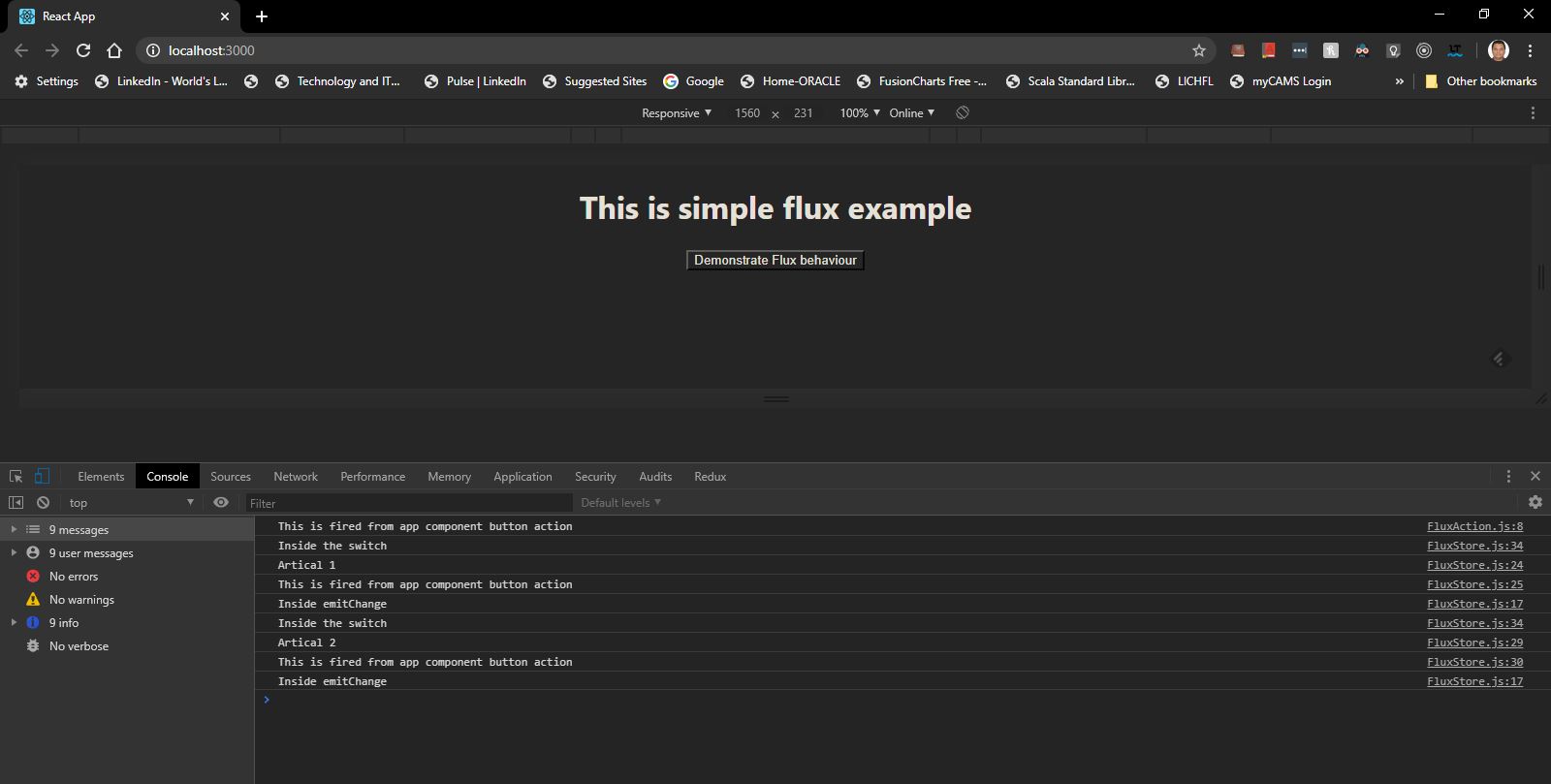
Flux is another open source Frame work provided by Facebook and suggested to use in React. As we have MVC framework in java application explained below
Controller :- This is the central hub of the MVC framework. It acts as an intermediate between model and view. Generally we always have one controller per application. Its main function is to handle all the request from the view in terms of action. Controller decide what to do with data either to send it to view part for showing to the user or it need to go to model to perform some DB or REST call.
View :- This represent the view part of the application and never talk directly with Model. It has to go through controller.
Model :- This represent the data that is coming from Controller. It never talk directly with View. It either take data from Controller and perform W/S or DB call or take the data from DB or REST and give to controller by informing to provide it to view for rendering.
Action --> Controller
| |
View Model
Flux is also similar to MVC but unidirectional. It has following part
Action --> Dispatcher --> Store -->View
Lets discuss few of the aspect of all describe above
'- Action send the action using dispatch to dispatcher
'- Dispacther take the action and send the data and action type to store
'- store responds to the dispatched action using register, switch and case.
'- Store finally emits change to view and view update as per the need.
In short user perform the action i.e. clicking on button this action is send to Action class which call the Dispacther class by sending the action type (to differentiate different action) and data and then dispatcher using dispatch this action and data to all the store who has already register them self with this dispatcher. Finally when the data is taken by the store class it perform the operation and send event to View to do changes.
Please refer to the working code as given below
You can also download the code from URL
https://github.com/shdhumale/SimpleReactFluxExample
Folder structure
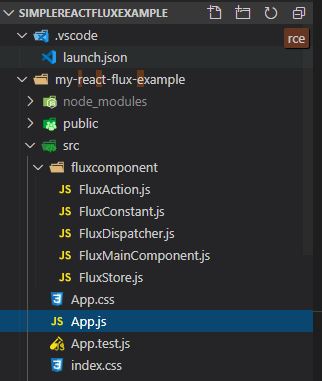
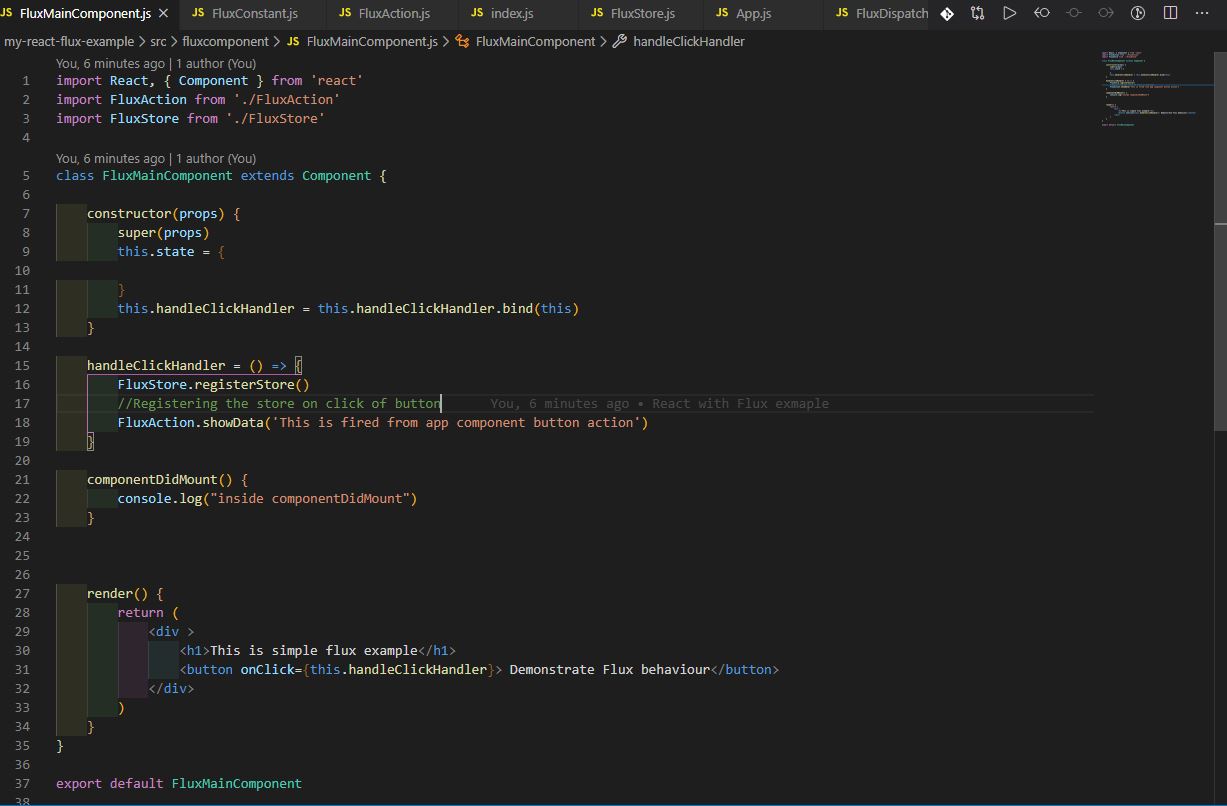


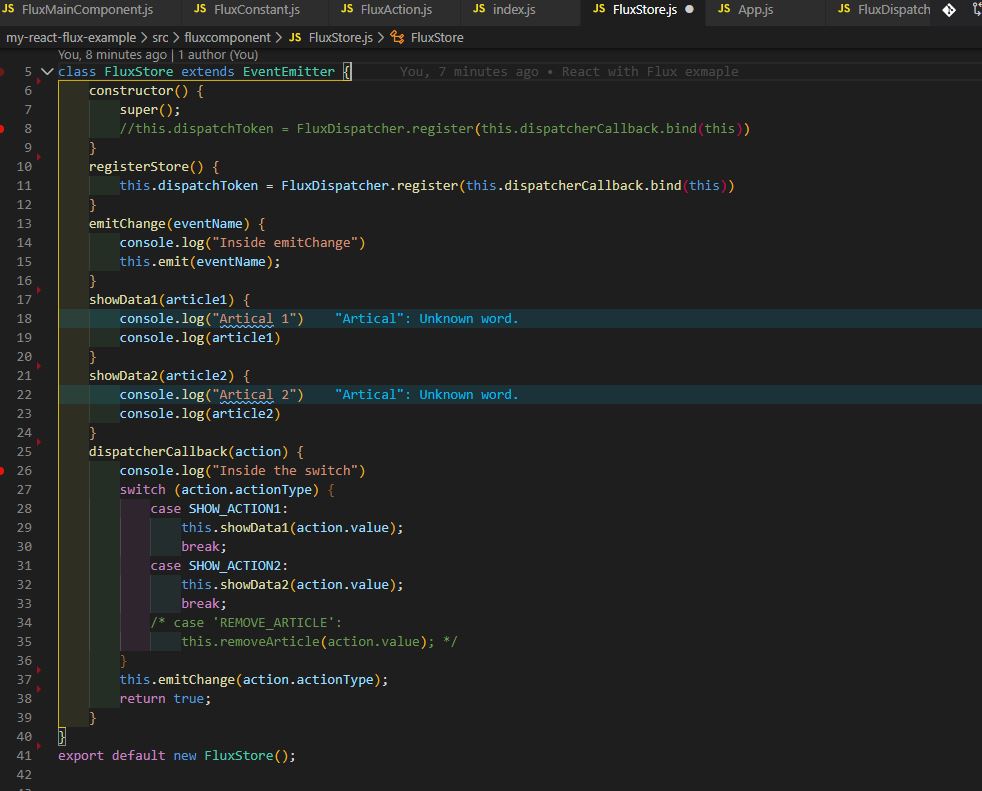


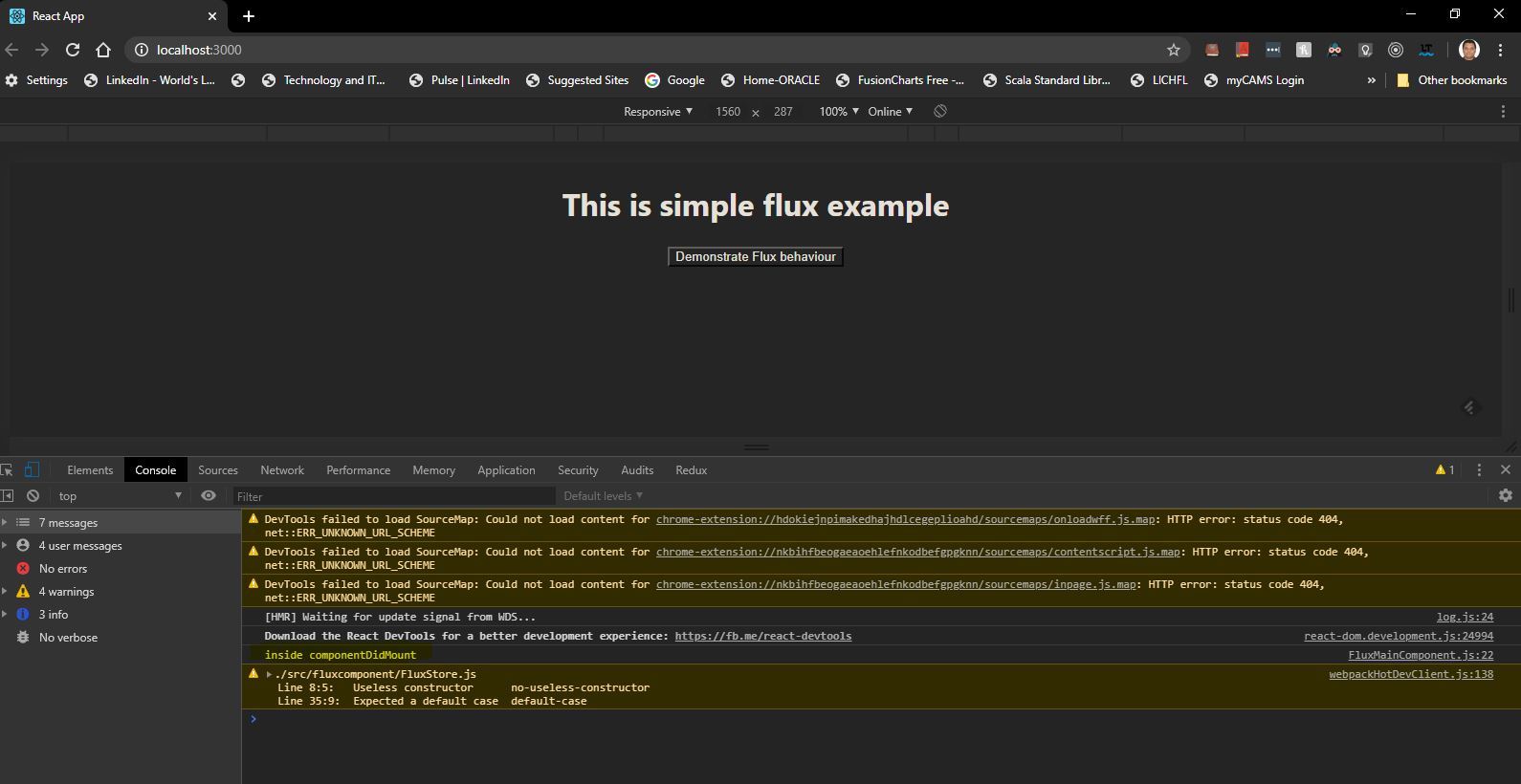
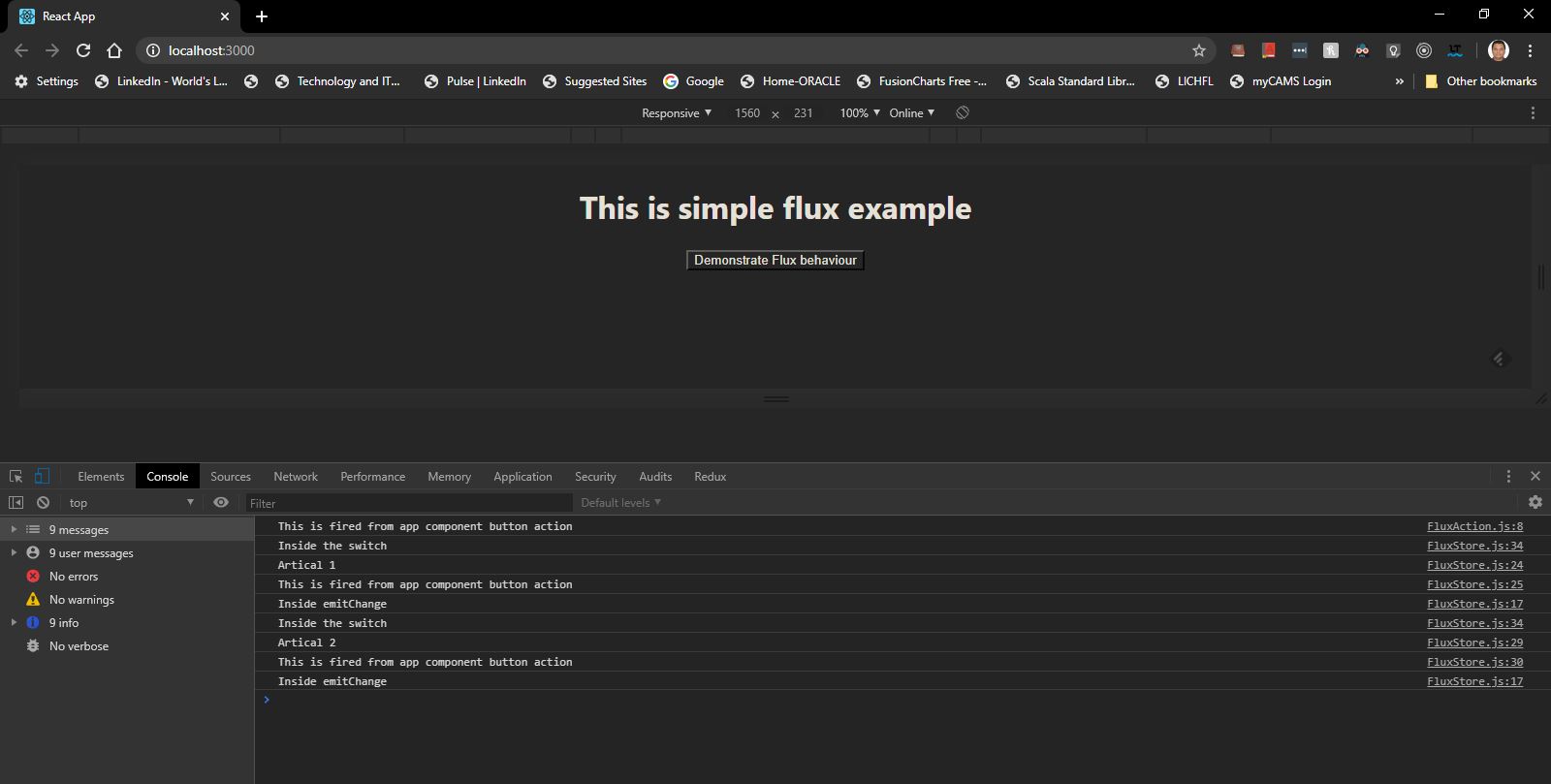