1- Create folder
mkdir siddhu-docker-app
2- Provide all rights to this folder
chmod 77 -R siddhu-docker-app
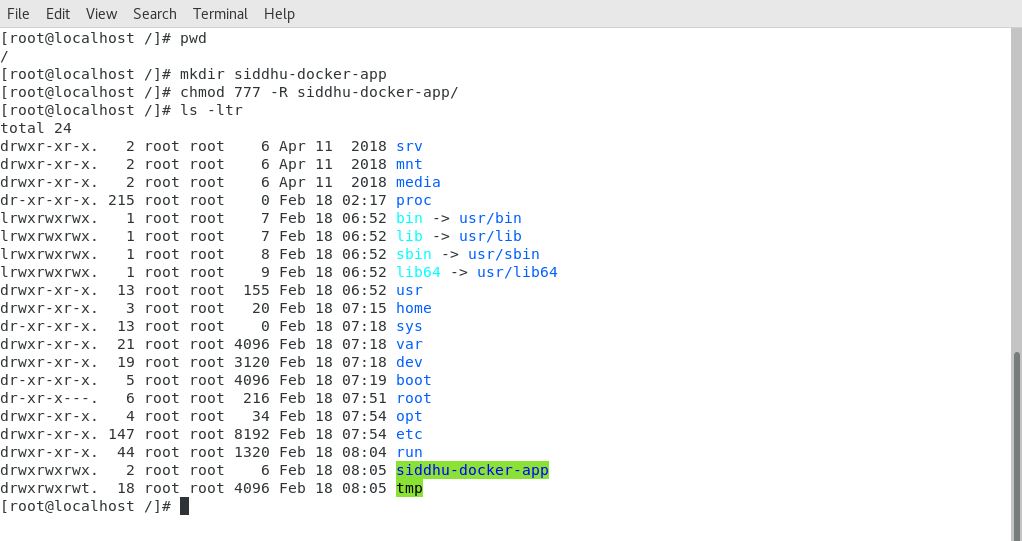
3- Create simple helloworld java programe
class sidduhello{
public static void main(String[] args){
System.out.println("Hello from using Docker");
}
}
Save it inside the directory siddhu-docker-app as sidduhello.java.
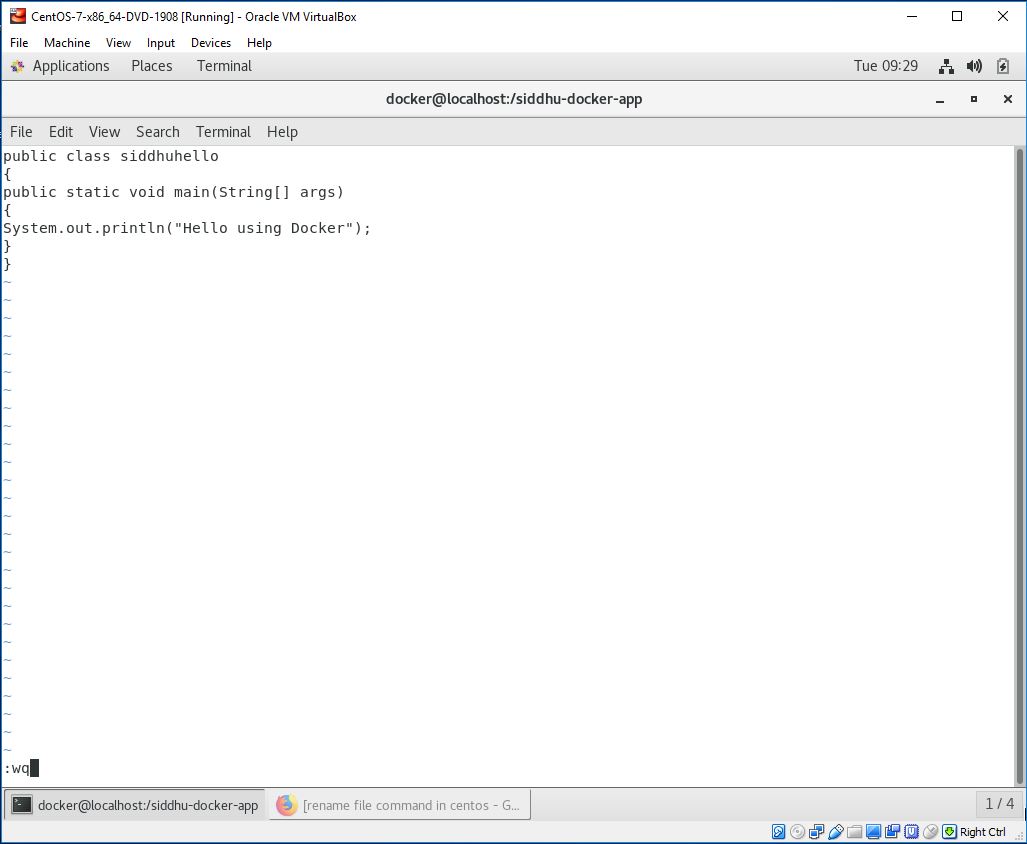
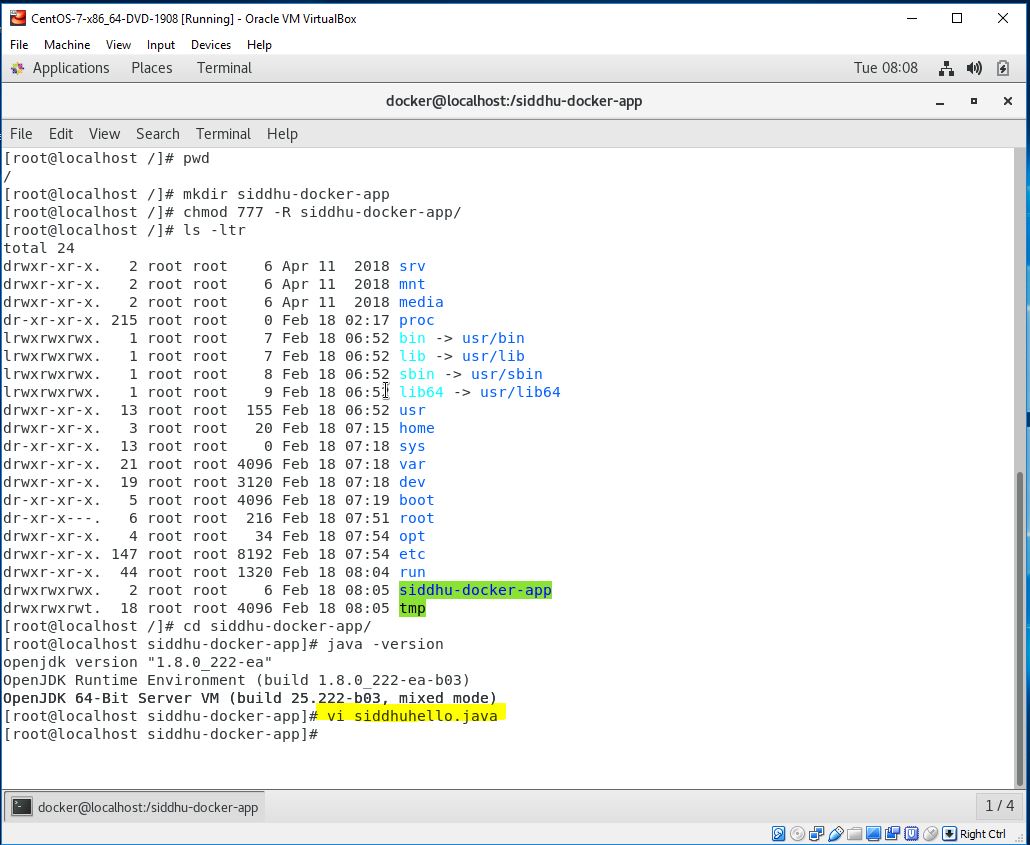
4- Lets Create a Dockerfile with name as "Dockerfile"
Dockerfile contains instructions for the Docker. It did not have any file extension.
// Start of Dockerfile text
FROM java:8
COPY . /var/www/java
WORKDIR /var/www/java
RUN javac sidduhello.java
CMD ["java", "sidduhello"]
// End of Dockerfile text
Note:- Write all instructions in uppercase because it is convention.
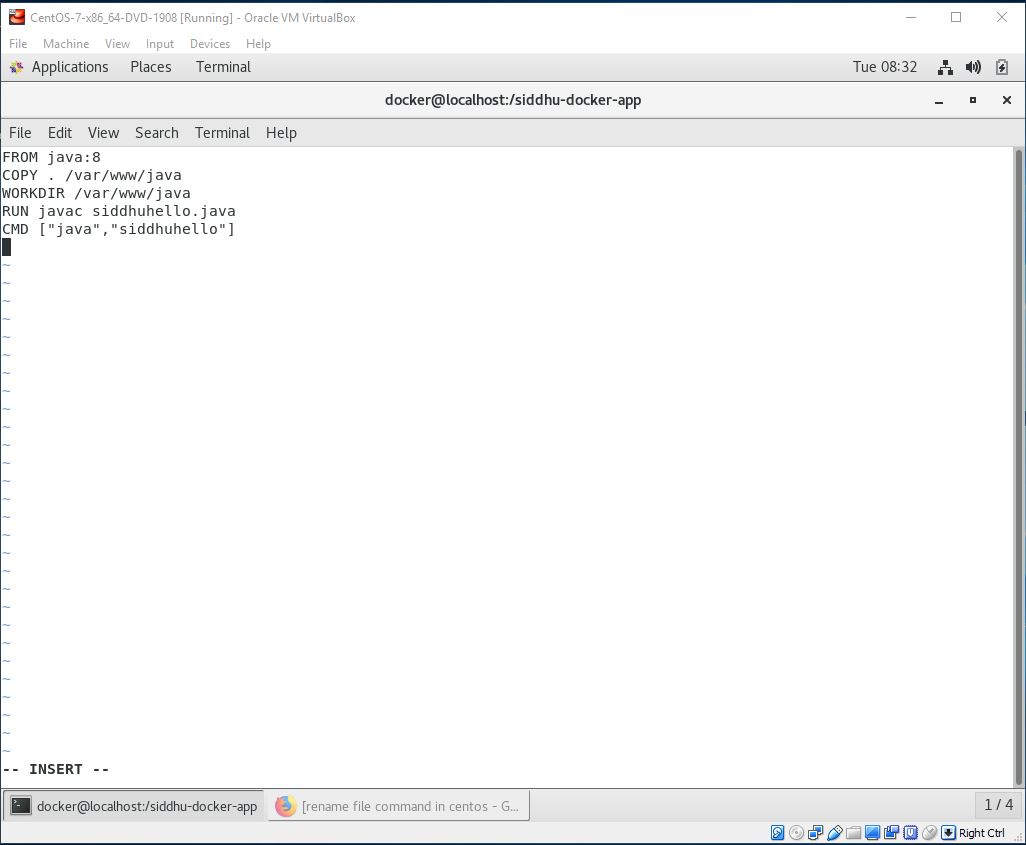
Now make sure to have our both files i.e. Dockerfile and sidduhello.java in out siddhu-docker-app directory.
5- Lest Build Docker Image
After creating the docker Files we need to create the docker images.Docker image contains all the code+lib+class+packages etc that is required to be executed in docker containers.
Now change the working directory i.e. move inside our siddhu-docker-app folder
Give chmod 777 to both these file as shown below
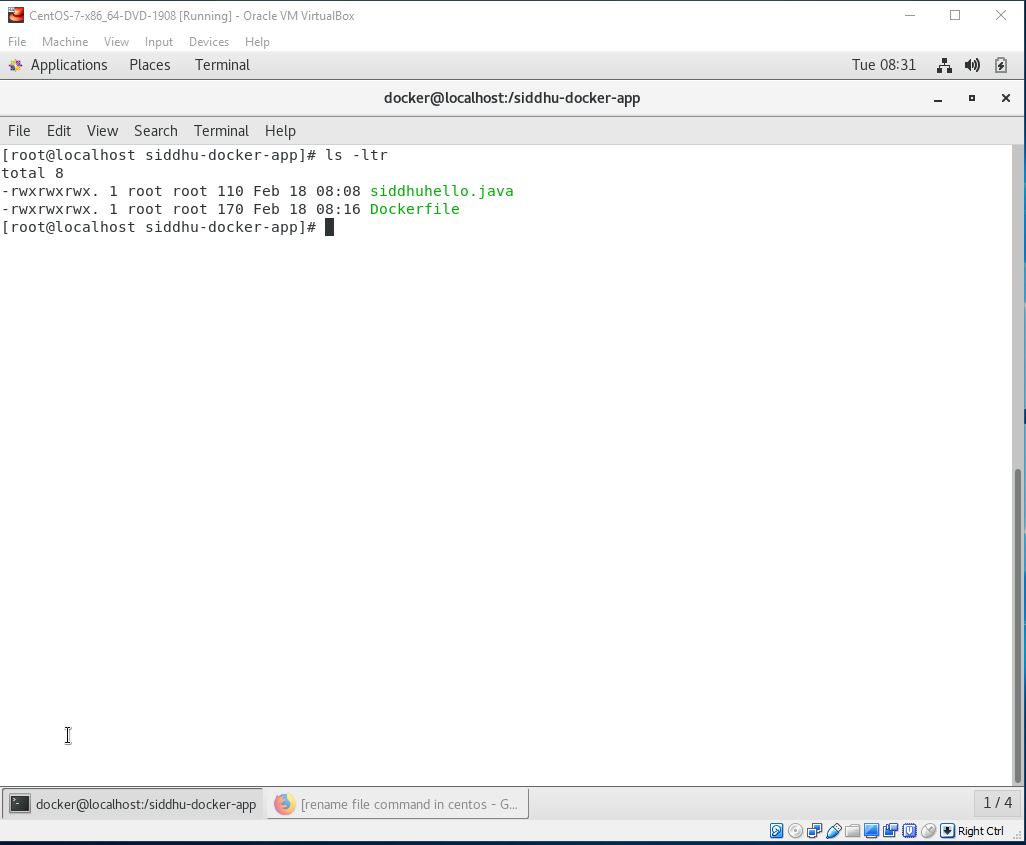
Execute below command
docker build -t siddhu-hello-java-app .
Descriptions:-
docker:- this command tell installed docker progrmae to take some action
build :- This command is used to create the Images
siddhu-hello-java-app :- This is the name of the images and its upto you what name you want to give.
. :- final dots say that docker files which need to be used to create image is located in the current directory
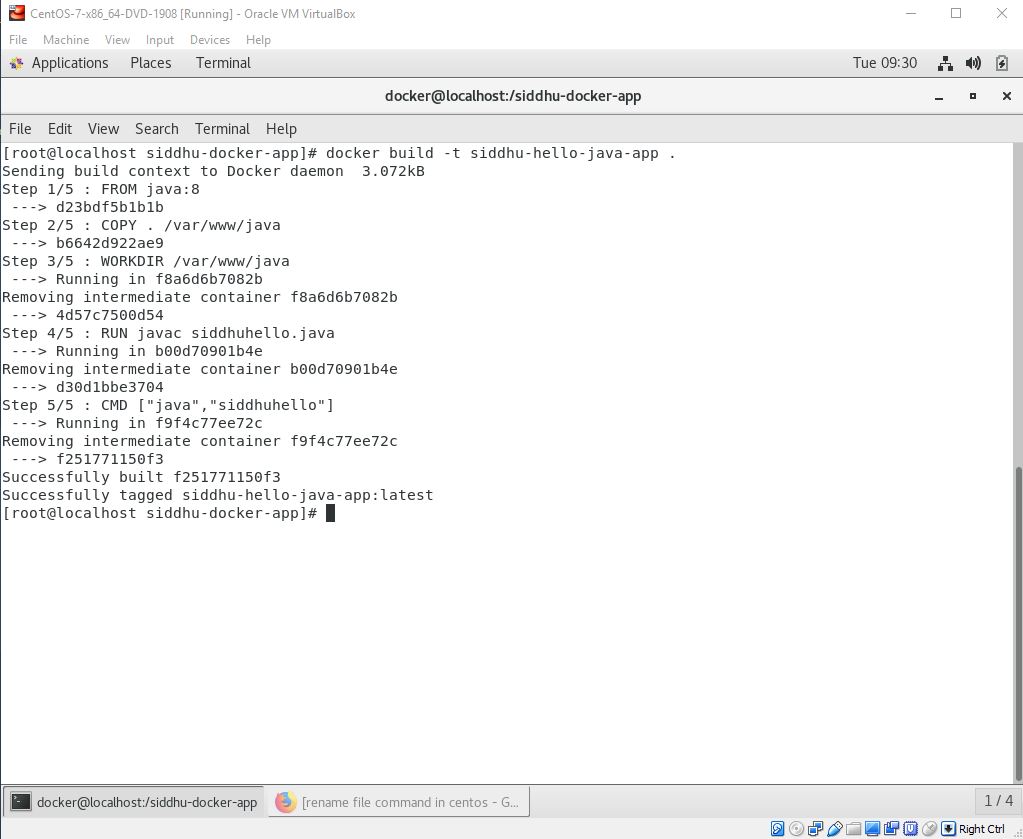
After successfully building the image. Now, we can run our docker image.
6- Now lets Run Docker Image
Once the docker image is build this can be taken as the core file to build docker container.
docker run siddhu-hello-java-app
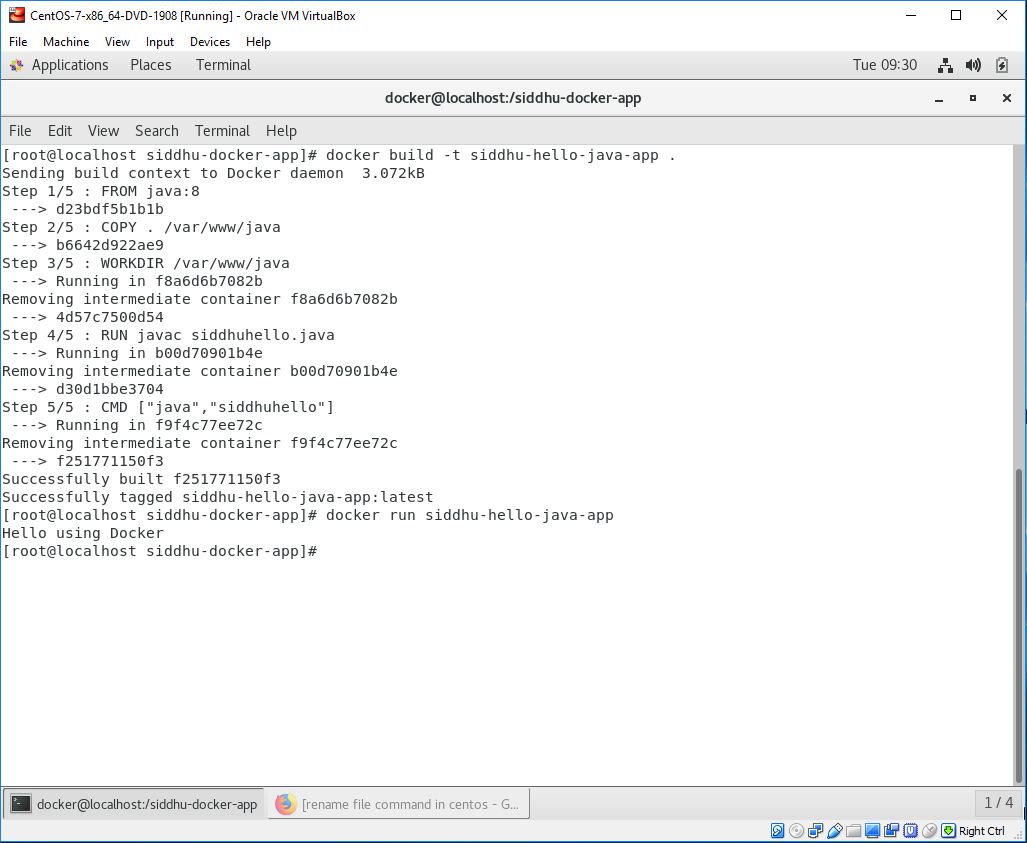
In general practise Docker Images are huge in size so Devops engineer chose the option to download the docker files from Docker hub/Git hub using Jenkin and then using installed docker on the respective machine build docker files from it. And as on when required we execute this file using run command to create Docker container
mkdir siddhu-docker-app
2- Provide all rights to this folder
chmod 77 -R siddhu-docker-app
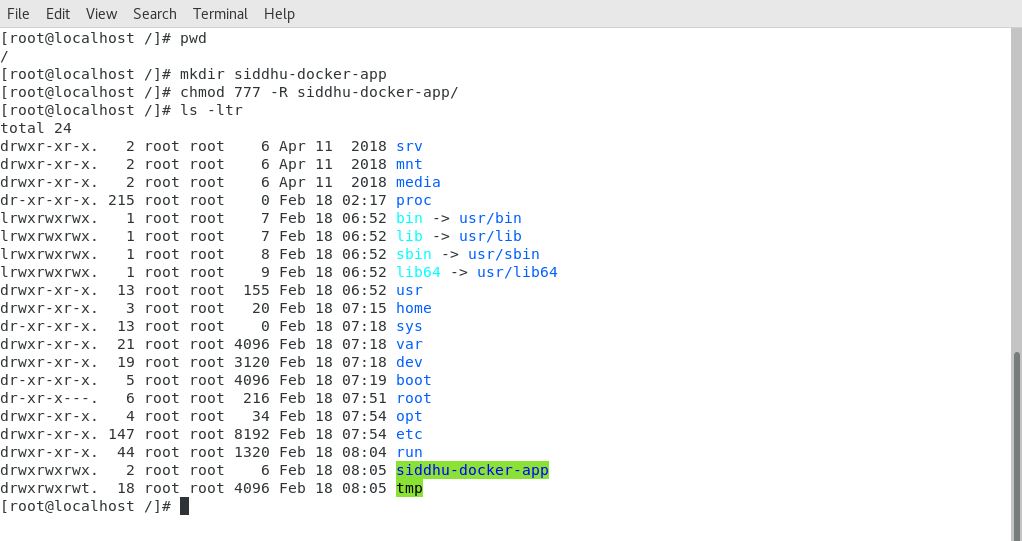
3- Create simple helloworld java programe
class sidduhello{
public static void main(String[] args){
System.out.println("Hello from using Docker");
}
}
Save it inside the directory siddhu-docker-app as sidduhello.java.
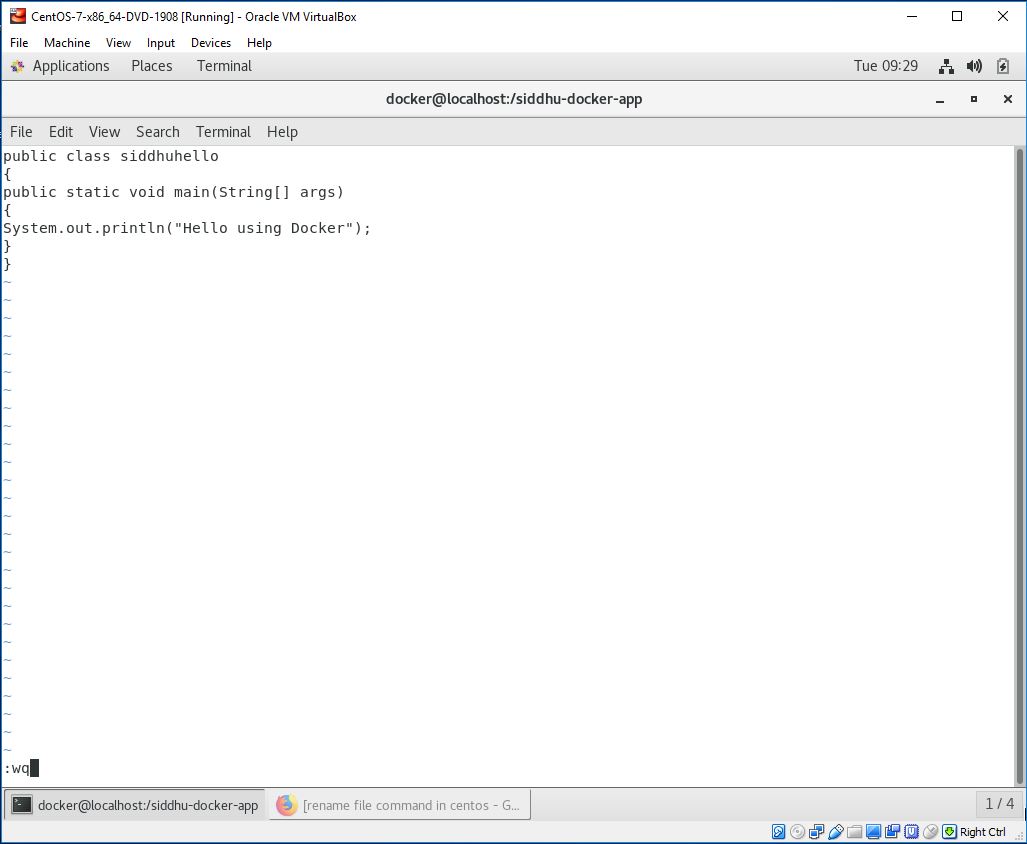
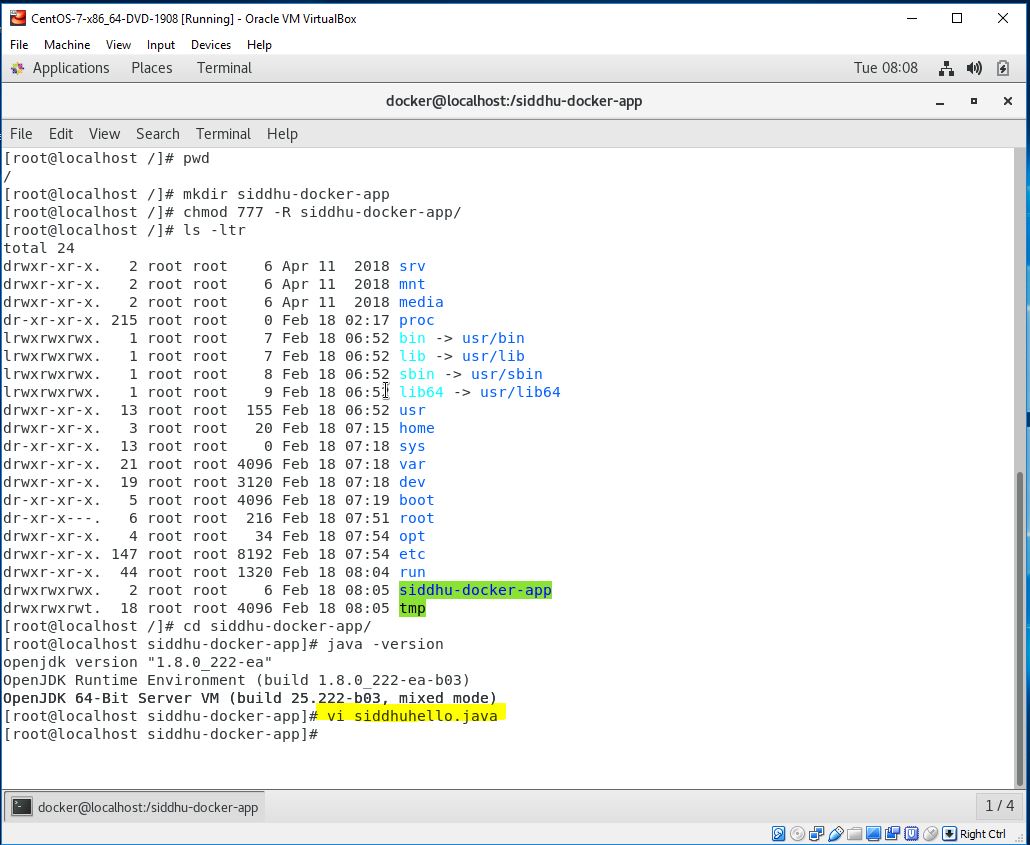
4- Lets Create a Dockerfile with name as "Dockerfile"
Dockerfile contains instructions for the Docker. It did not have any file extension.
// Start of Dockerfile text
FROM java:8
COPY . /var/www/java
WORKDIR /var/www/java
RUN javac sidduhello.java
CMD ["java", "sidduhello"]
// End of Dockerfile text
Note:- Write all instructions in uppercase because it is convention.
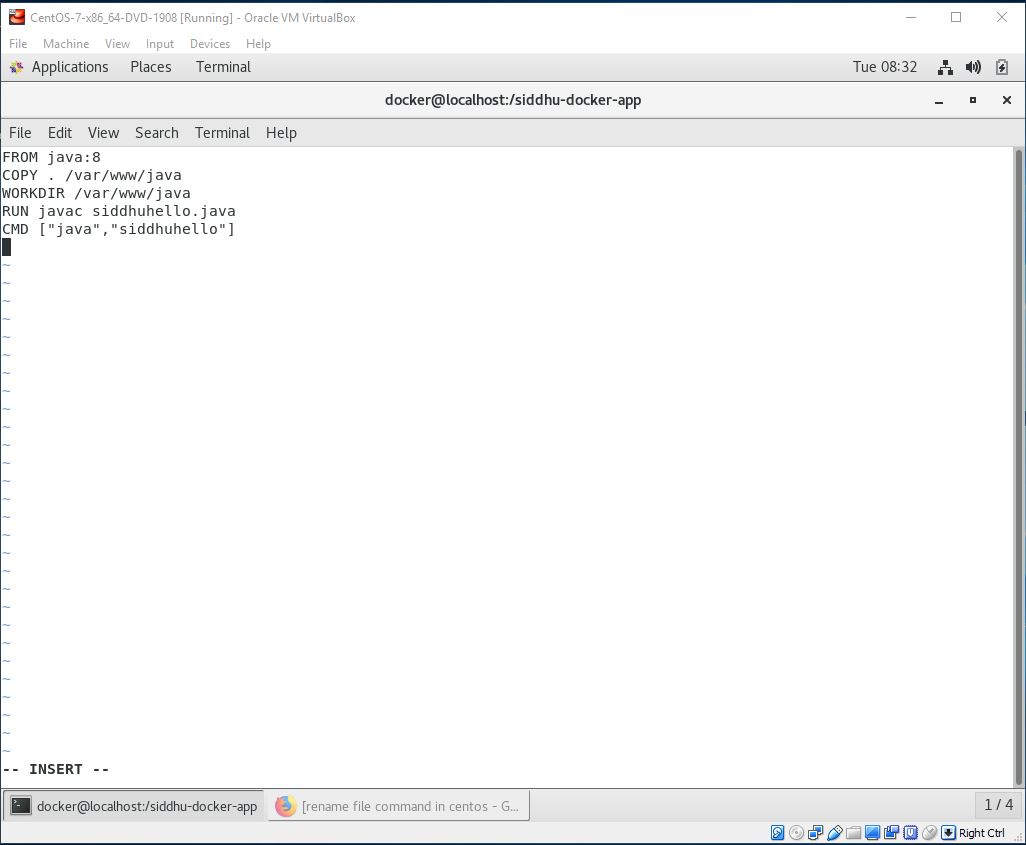
Now make sure to have our both files i.e. Dockerfile and sidduhello.java in out siddhu-docker-app directory.
5- Lest Build Docker Image
After creating the docker Files we need to create the docker images.Docker image contains all the code+lib+class+packages etc that is required to be executed in docker containers.
Now change the working directory i.e. move inside our siddhu-docker-app folder
Give chmod 777 to both these file as shown below
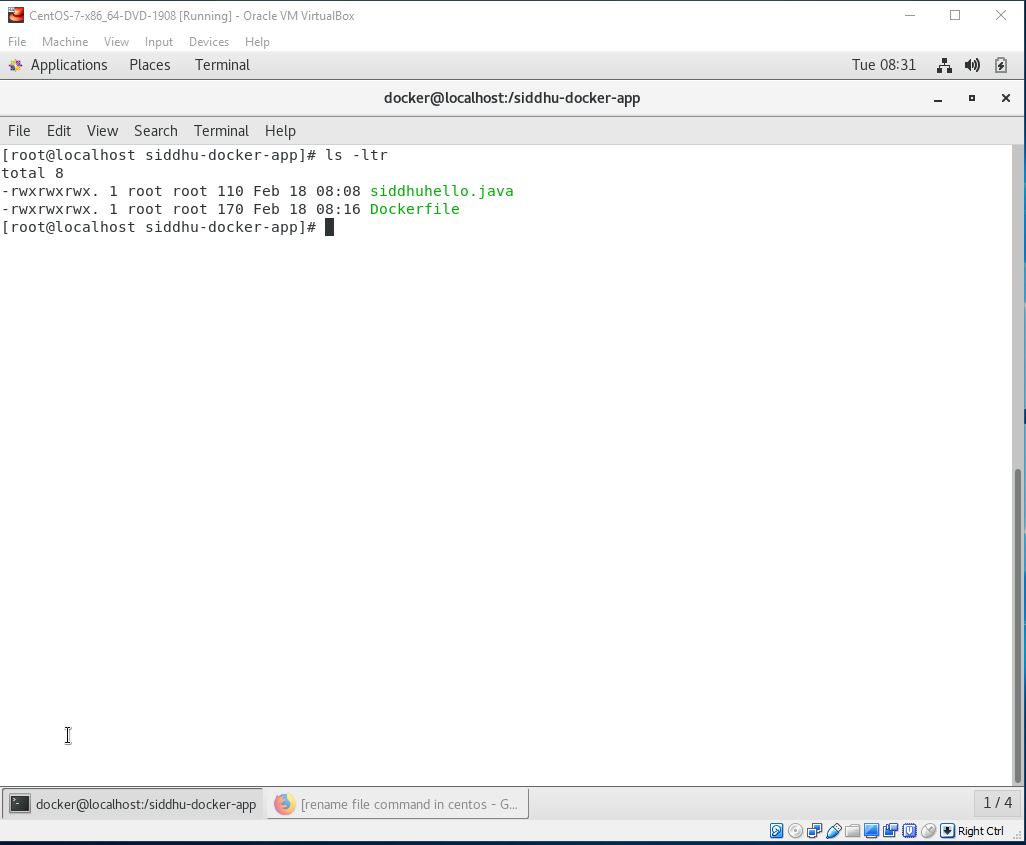
Execute below command
docker build -t siddhu-hello-java-app .
Descriptions:-
docker:- this command tell installed docker progrmae to take some action
build :- This command is used to create the Images
siddhu-hello-java-app :- This is the name of the images and its upto you what name you want to give.
. :- final dots say that docker files which need to be used to create image is located in the current directory
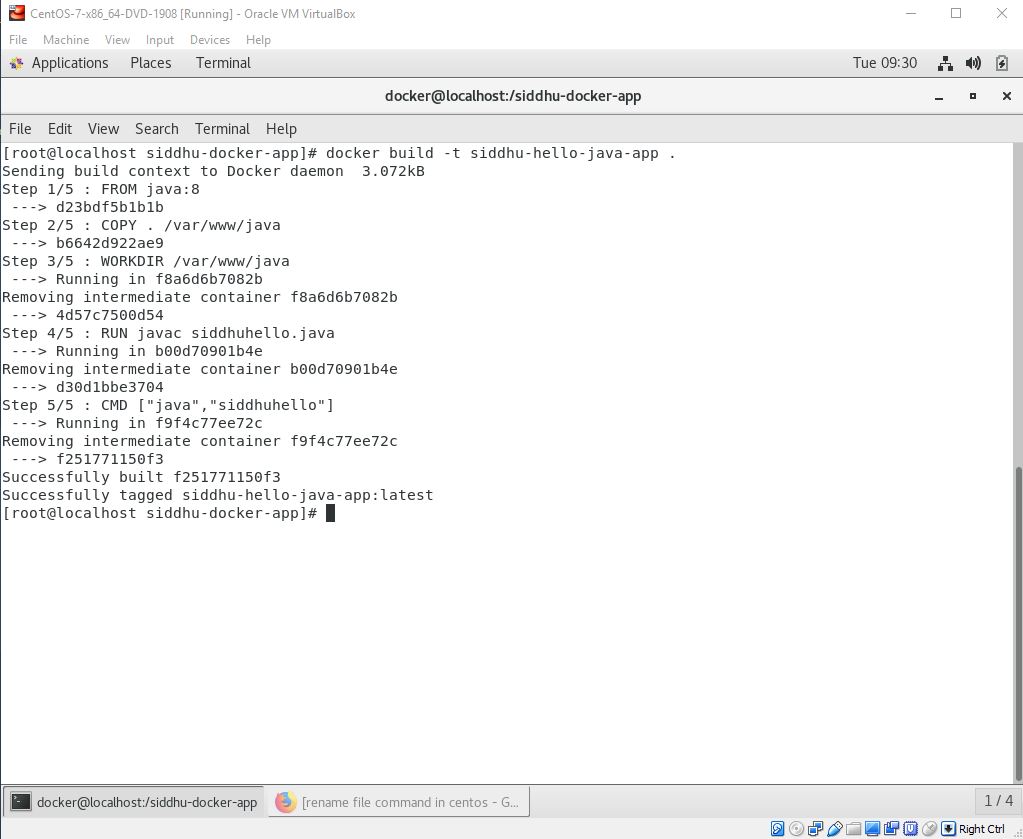
After successfully building the image. Now, we can run our docker image.
6- Now lets Run Docker Image
Once the docker image is build this can be taken as the core file to build docker container.
docker run siddhu-hello-java-app
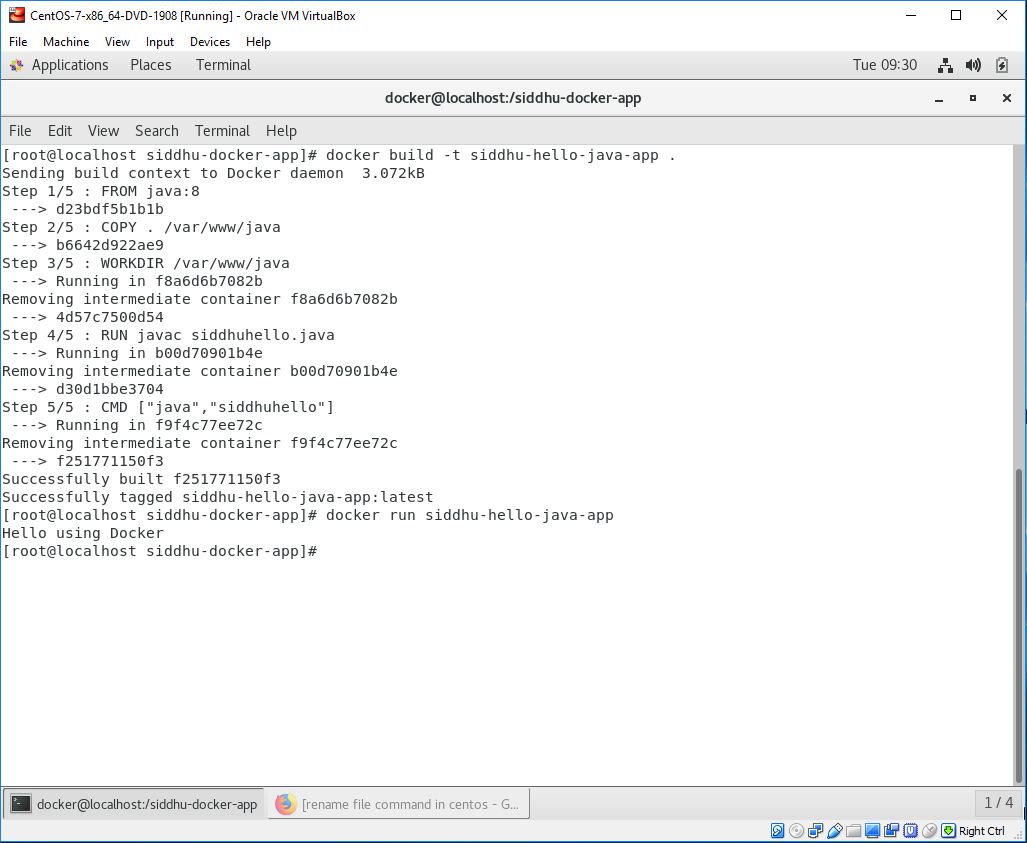
In general practise Docker Images are huge in size so Devops engineer chose the option to download the docker files from Docker hub/Git hub using Jenkin and then using installed docker on the respective machine build docker files from it. And as on when required we execute this file using run command to create Docker container
There are many commands in Docker but most of the time you will be working on following commands
1- Start
2- Stop
3- Pull
4- Push
5- PS
6- PS all
7- log
8- exec -it
9- rm
10- rmi
11- image