Lets try to now call REST service from the camunda and dispaly the output on the prompt.
Friday, April 28, 2023
Tuesday, April 25, 2023
Calling Camunda process using PostMan REST API
Best of camunda is we can perform operatio using REST API call. i.e. let say you have one bpnm file and uploaded on Camunda server and you want to access the process then you can do using REST api call.
Base url to do it
[http://localhost:8080/engine-rest/process-definition/pricess-defincation-key/start]
As shown belwo we have one process running and its id is
siddhu_process_1:1:bc2e074e-e35d-11ed-8f2e-7e7a91225434

our url will be
for sending data we can use belwo JSON format
{
“variables”: {
“name”: {“value”:”Niall”,”type”:”String”},
“age”:{“value”:”100″,”type”:”long”}
}
}
Lets now start this process using POSTMAN as show below and click on Send button

you will see the our check user user task in process will have one item on the screen.

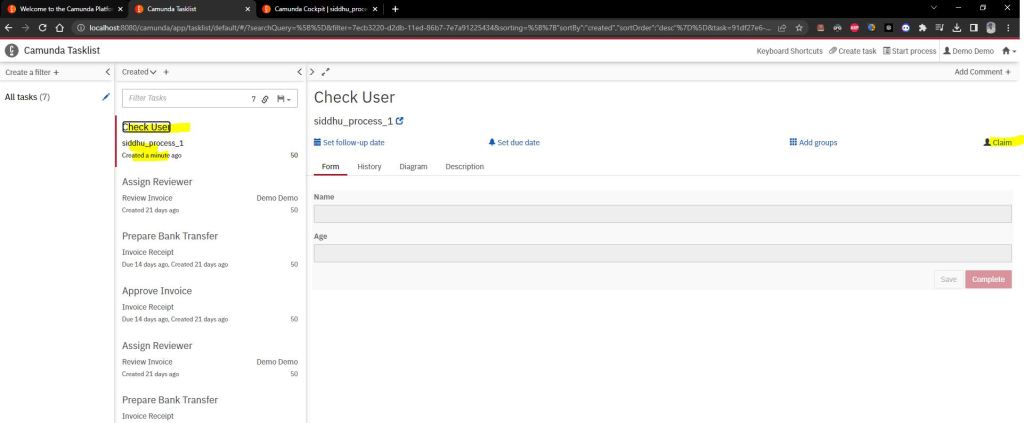


Passing data from External service task to Delegate Service task Camunda
In this we will try to do following things
1- we will create a sevice task (external) that will send data to another service task (delegate) and we will check if the data is send properly or not using embedded camunda.
In past example https://shdhumale.wordpress.com/2023/04/24/calling-java-class-from-camunda-task-process/
we had tried to create bpmn file that will ask for user inpur when uploaded on the camunda server and when we start our springboot application it will contant this service task in bmnp file and fetch the value that is entered by the user using ExternalTaskHandler.
Now lets talk about the second concept we had talk above i.e. using Delegate in sprigboot.
In this exercise we wil get the input from bpmn files and then modify it in our java class and then we are transeferring the two values from spring boot class to another Service task in bpmn file service task that will send that values to our other java class using delegate.
Please flow below step religiously
1- Create a bpmn file
2- upload that file on local standalone camunda server.
3- Write java class with ExternalTaskHandler that will take input parameter from bpmn files.
4- finally we will modify this input paramter and send it back to another bpmn service which send the data to java class using delegate and will dispaly the modified values.
lets start
1- Create a bpmn file:-
Please follow below steps.

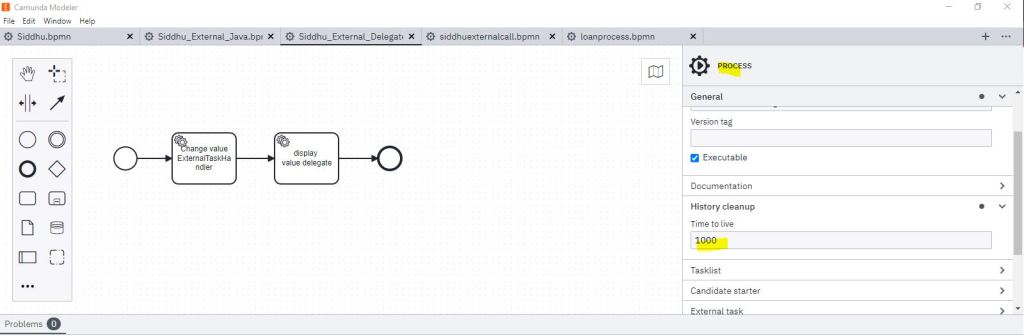
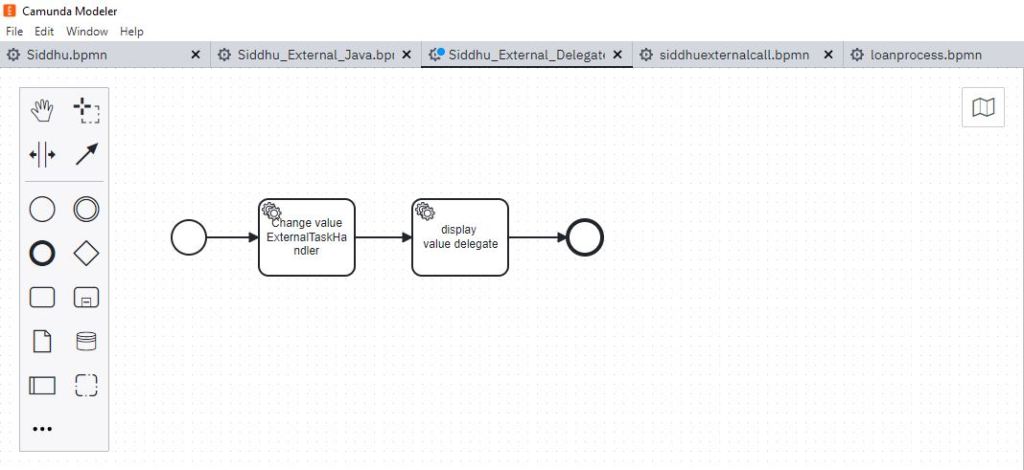

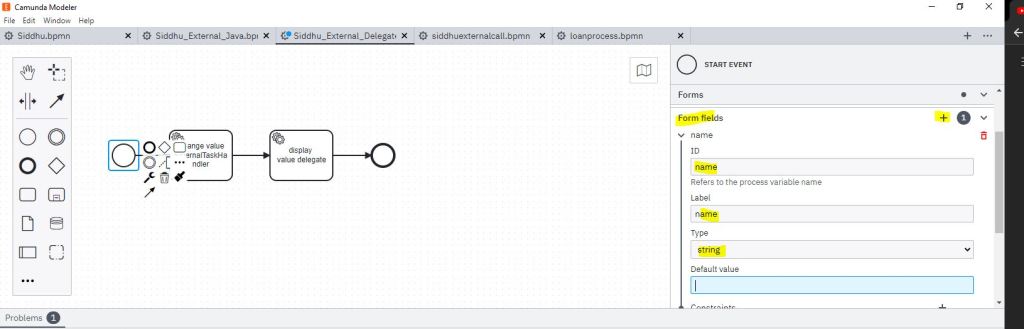
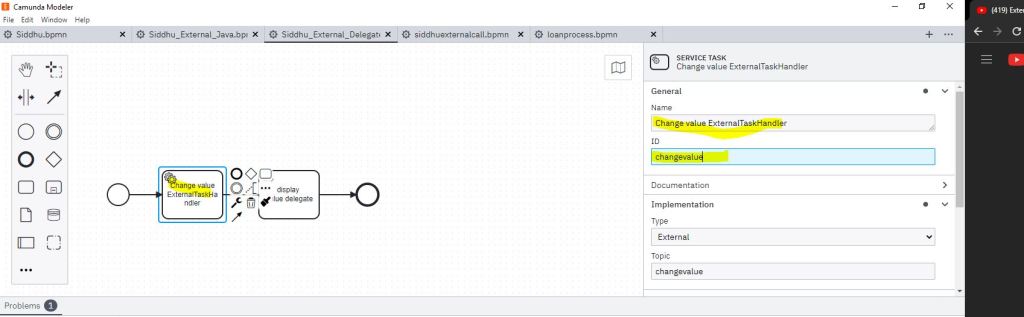



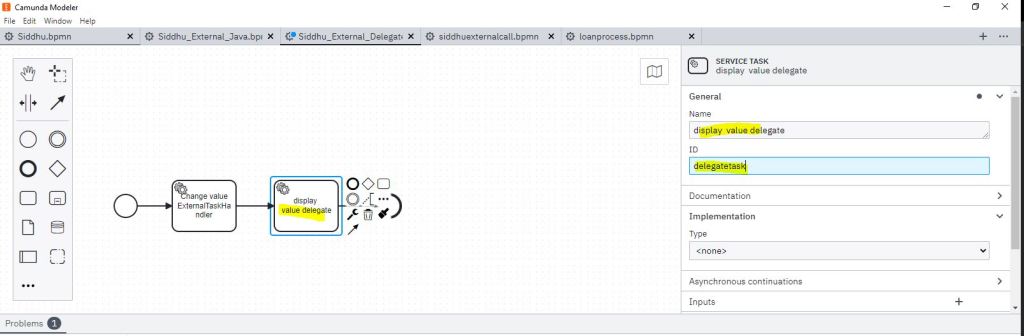

2- upload that file on local standalone camunda server.
Start you standalone camunda server and login to the tasklist using demo/demo.
follow below steps to upload bpmn files.



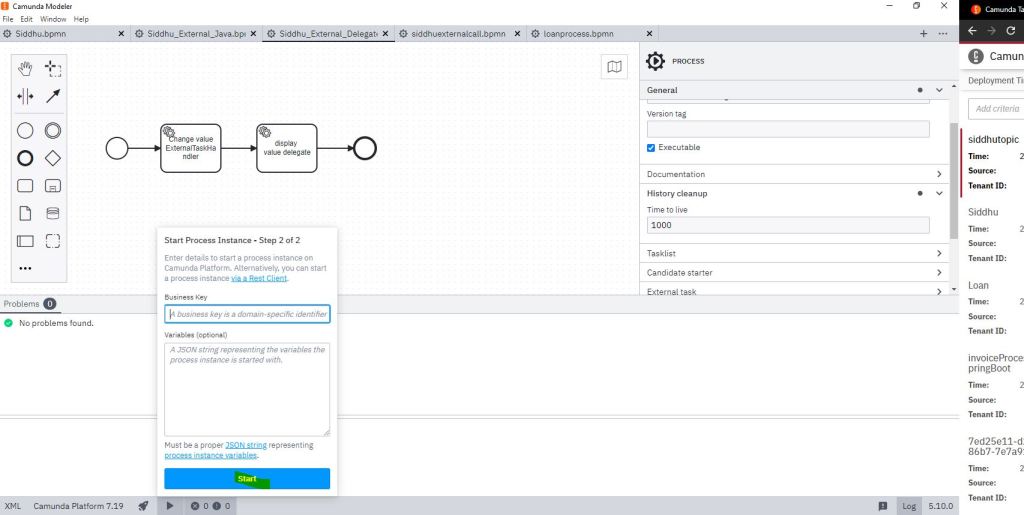
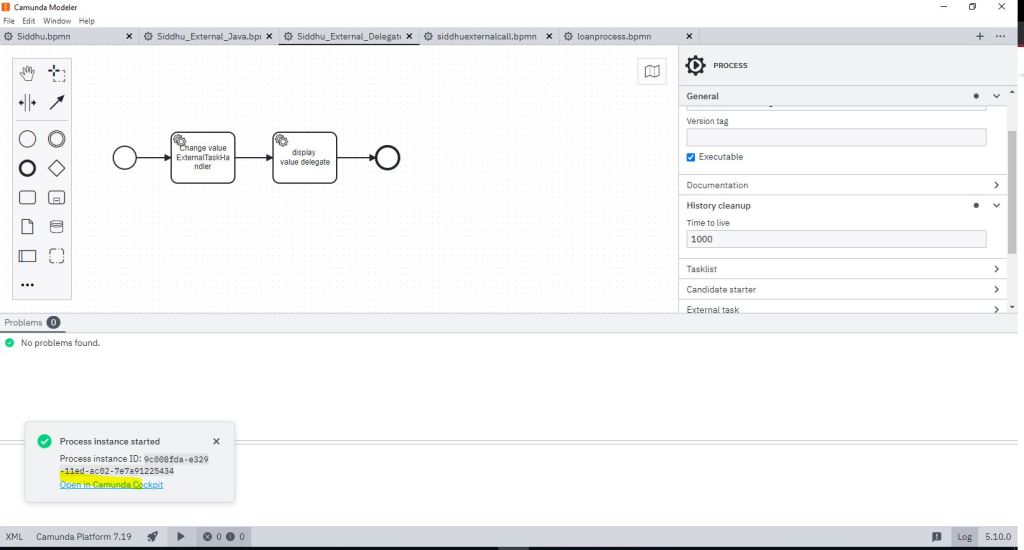
Go to below url and start the process.
check the process is imported properly using belwo url
http://localhost:8080/camunda/app/cockpit/default/#/processes

now lets start the process using belwo url
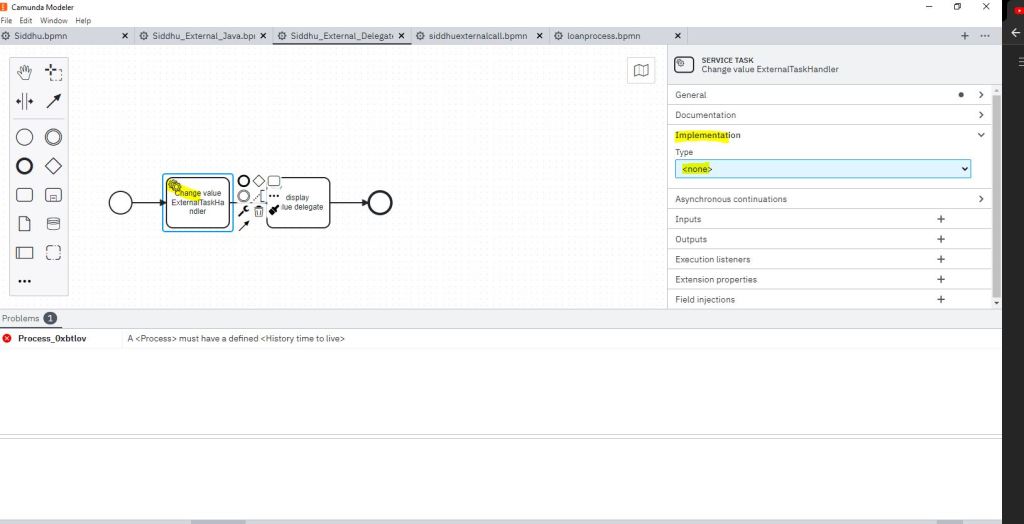



3- Write java class with ExternalTaskHandler that will take input parameter from bpmn files.
follow below steps for the same.

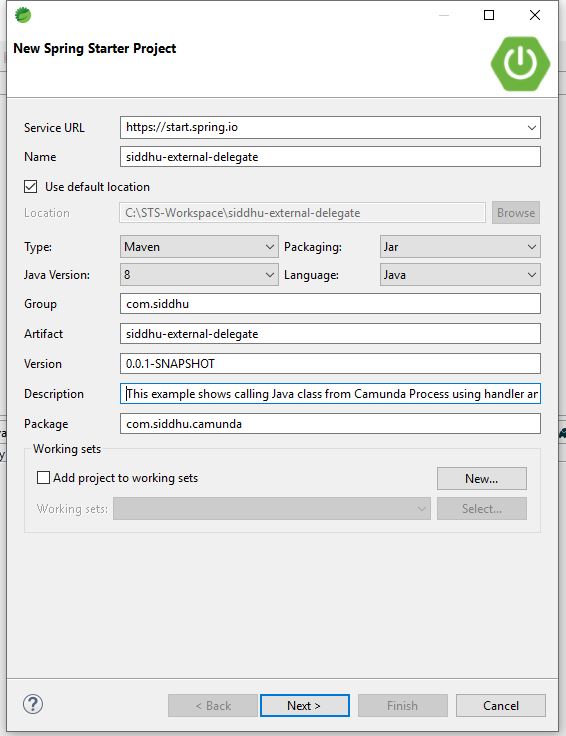
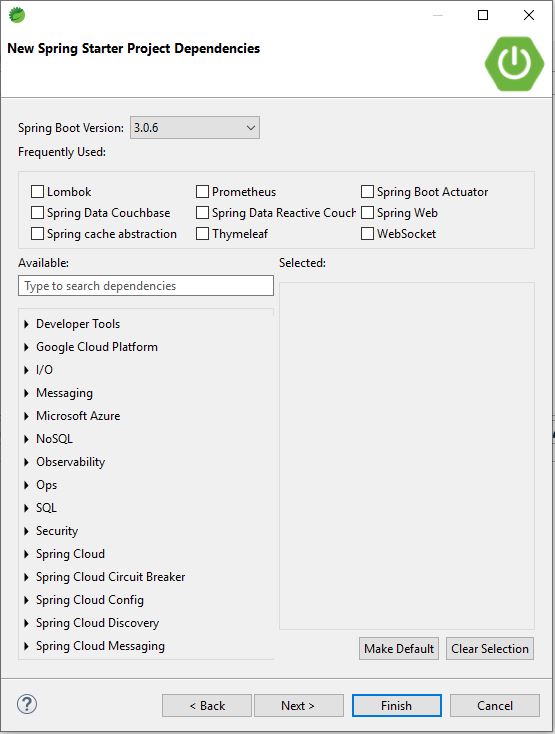

Now update the pom.xml as
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.7.3</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>com.siddhu</groupId> <artifactId>siddhu-external-delegate</artifactId> <version>0.0.1-SNAPSHOT</version> <name>siddhu-external-delegate</name> <description>This example shows calling Java class from Camunda Process using handler and then sending value back to another java class using delegate via Service task </description> <properties> <java.version>1.8</java.version> <camunda.platform.runtime.version>7.18.0</camunda.platform.runtime.version> <spring.boot.version>2.7.3</spring.boot.version> <maven.compiler.plugin.version>3.8.1</maven.compiler.plugin.version> </properties> <dependencies> <dependency> <groupId>org.camunda.bpm.springboot</groupId> <artifactId>camunda-bpm-spring-boot-starter-external-task-client</artifactId> <version>${camunda.platform.runtime.version}</version> </dependency> <dependency> <groupId>com.sun.xml.bind</groupId> <artifactId>jaxb-impl</artifactId> <version>2.3.5</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>${spring.boot.version}</version> <executions> <execution> <id>repackage</id> <goals> <goal>repackage</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>${maven.compiler.plugin.version}</version> <configuration> <source>8</source> <target>8</target> </configuration> </plugin> </plugins> </build> </project> |
Check project is compiling and building properly using belwo screen after modification in pom.xml

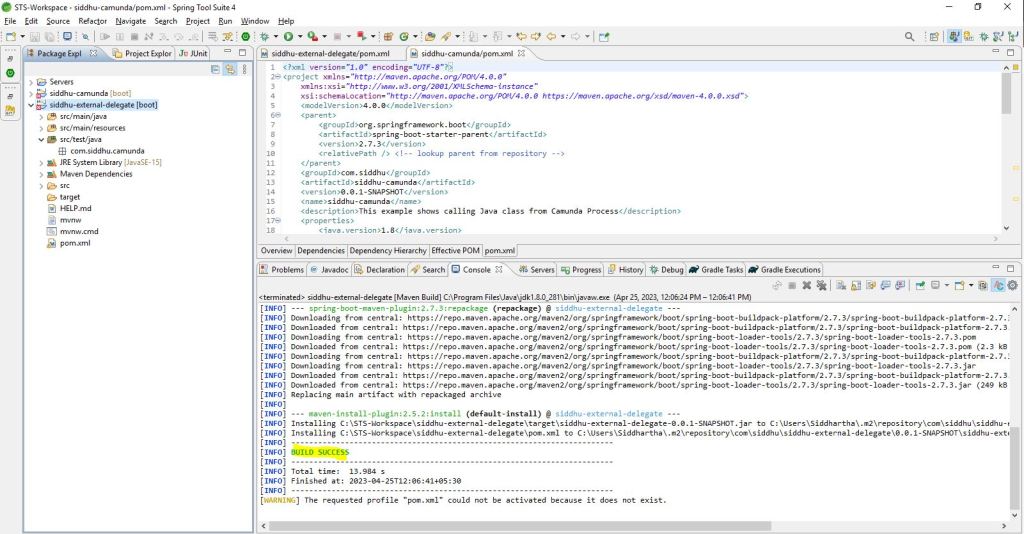
But first change the application.yaml files.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | camunda.bpm.client: base-url: http://localhost:8080/engine-rest # the URL pointing to the Camunda Platform Runtime REST API lock-duration: 10000 # defines how many milliseconds the External Tasks are locked until they can be fetched again subscriptions: changevalue: # This is topic name variable-names: name,age # only fetch these variables process-definition-key: siddhuexternaldelegate #this is the id that we give for process ID camunda.bpm: admin-user: id: demo password: demo firstName: Demo filter: create: All tasks |
Now lets create our java class that will be called from our camunda process
1 |
Now lets run the application and check we are able to get the name and age that we set in start of bpmn files
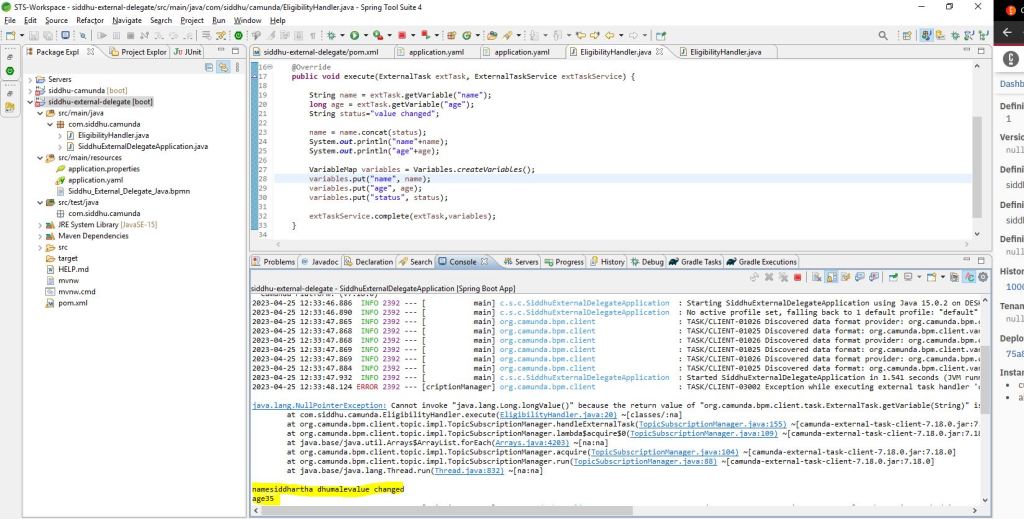
Now lets change this value in our class and send it to our another service task.
Please follow below process religiously

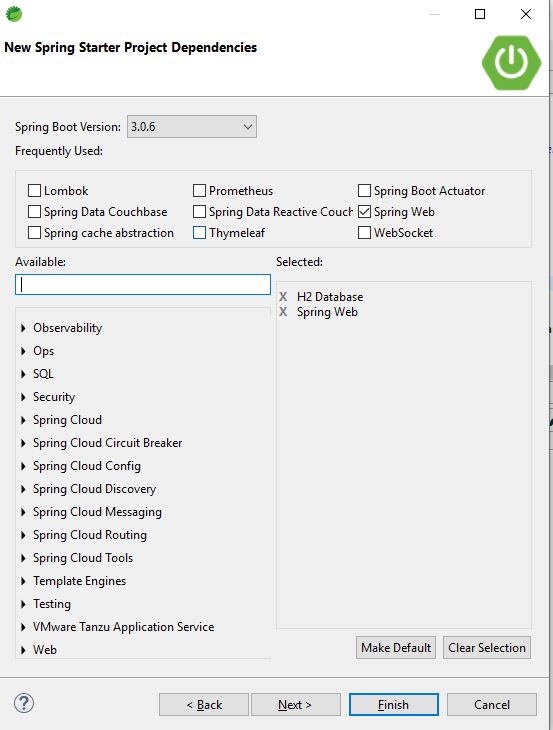

Now lets modify our pom.xml as given below to inject dependencies
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.6.1</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>com.siddhu</groupId> <artifactId>siddhu-delegate-task</artifactId> <version>0.0.1-SNAPSHOT</version> <name>siddhu-delegate-task</name> <description>This example take value send from java ExternalTaskHandler from one of the service task to respective this task</description> <properties> <java.version>8</java.version> <camunda.spring-boot.version>7.16.0</camunda.spring-boot.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.camunda.bpm.springboot</groupId> <artifactId>camunda-bpm-spring-boot-starter-webapp</artifactId> <version>${camunda.spring-boot.version}</version> </dependency> <dependency> <groupId>org.camunda.bpm.springboot</groupId> <artifactId>camunda-bpm-spring-boot-starter-rest</artifactId> <version>${camunda.spring-boot.version}</version> </dependency> <dependency> <groupId>com.sun.xml.bind</groupId> <artifactId>jaxb-impl</artifactId> <version>2.3.5</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>5.2.8.RELEASE</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project> |
Now make following files
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | 1- SiddhuDelegateTaskApplication package com.siddhu.camunda; import org.camunda.bpm.spring.boot.starter.annotation.EnableProcessApplication; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication @EnableProcessApplication public class SiddhuDelegateTaskApplication { public static void main(String[] args) { SpringApplication.run(SiddhuDelegateTaskApplication.class, args); } } 2-ServletInitializer package com.siddhu.camunda; import org.springframework.boot.builder.SpringApplicationBuilder; import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; public class ServletInitializer extends SpringBootServletInitializer { @Override protected SpringApplicationBuilder configure(SpringApplicationBuilder application) { return application.sources(SiddhuDelegateTaskApplication.class); } } 3- DelegateTask package com.siddhu.camunda; import javax.inject.Named; import org.camunda.bpm.engine.delegate.DelegateExecution; import org.camunda.bpm.engine.delegate.JavaDelegate; @Named public class DelegateTask implements JavaDelegate{ @Override public void execute(DelegateExecution execution) throws Exception { // TODO Auto-generated method stub String name=(String) execution.getVariable("name"); String status=(String) execution.getVariable("status"); System.out.println("name"+name); System.out.println("status"+status); } } 4- application.yaml camunda.bpm: admin-user: id: demo password: demo firstName: Demo filter: create: All tasks |
Now first stop the standalone server and Now lets start our this application and check if we are getting this modified value inside our java class from next service task


now start our siddhu-external-delegate project

Now lets upload our bpnm file using moduler



Now lets start our deployed process


Check the values in our java programe
now you might be thinking was is difference between ExternalTaskHandler and delegate
let me try to expalin the same as we have use these both concept here.

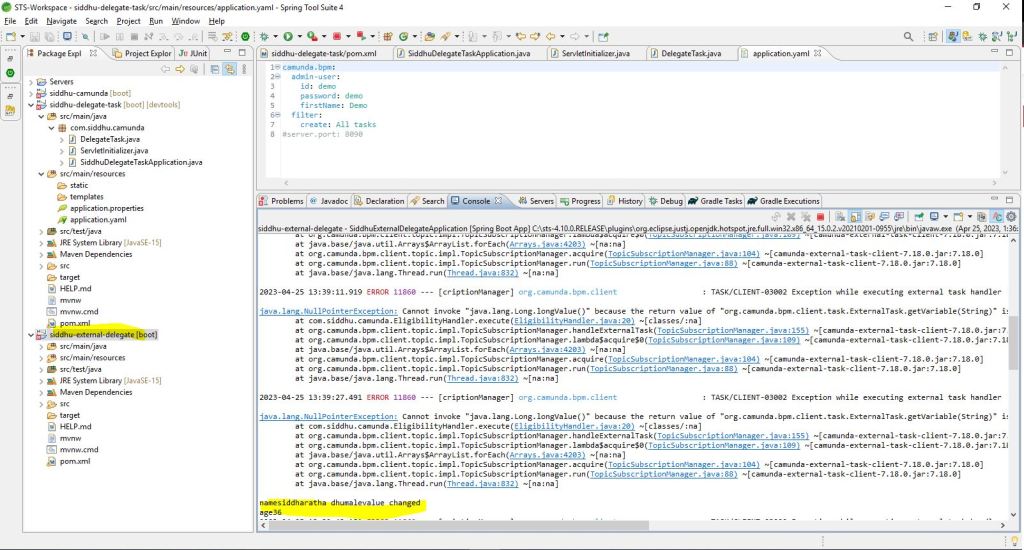
code download:-