1- Lets say we have following XML files along with DTD added in it
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE root [
<!ELEMENT root (element*)>
<!ELEMENT element (name,surname)>
<!ELEMENT name (#PCDATA)>
<!ELEMENT surname (#PCDATA)>
]>
<root>
<element>
<name>Siddhartha</name>
<surname>Dhumale</surname>
</element>
</root>
2- Following is the java class that is used to check if the given xml adhere to valid xsd file or not
package com.siddhu;
import java.io.IOException;
// DOM
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
// SAX
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.XMLReader;
import javax.xml.parsers.ParserConfigurationException;
import org.xml.sax.ErrorHandler;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.InputSource;
public class ValidateXMLUsingDTD {
private ValidateXMLUsingDTD() {}
public static boolean validateWithDTDUsingDOM(String xmlFiles)
throws ParserConfigurationException, IOException
{
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setValidating(true);
factory.setNamespaceAware(true);
DocumentBuilder builder = factory.newDocumentBuilder();
builder.parse(new InputSource(xmlFiles));
System.out.print("XML files is valid using Dom Parser:"+xmlFiles+"---");
return true;
}
catch (ParserConfigurationException pce) {
throw pce;
}
catch (IOException io) {
throw io;
}
catch (SAXException se){
return false;
}
}
public static boolean validateWithDTDUsingSAX(String xmlFiles)
throws ParserConfigurationException, IOException
{
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setValidating(true);
factory.setNamespaceAware(true);
SAXParser parser = factory.newSAXParser();
XMLReader reader = parser.getXMLReader();
reader.parse(new InputSource( xmlFiles ));
System.out.print("XML files is valid using SAX Parser:"+xmlFiles+"---");
return true;
}
catch (ParserConfigurationException pce) {
throw pce;
}
catch (IOException io) {
throw io;
}
catch (SAXException se){
return false;
}
}
public static void main (String args[]) throws Exception{
System.out.println(ValidateXMLUsingDTD.validateWithDTDUsingDOM("C:\\Latest_JAVA_eclipse_WorkSpace\\TestXMLFilesUsingDTD\\src\\com\\siddhu\\siddhu.xml"));
System.out.println(ValidateXMLUsingDTD.validateWithDTDUsingSAX("C:\\Latest_JAVA_eclipse_WorkSpace\\TestXMLFilesUsingDTD\\src\\com\\siddhu\\siddhu.xml"));
}
}
For valid xml we will get below output
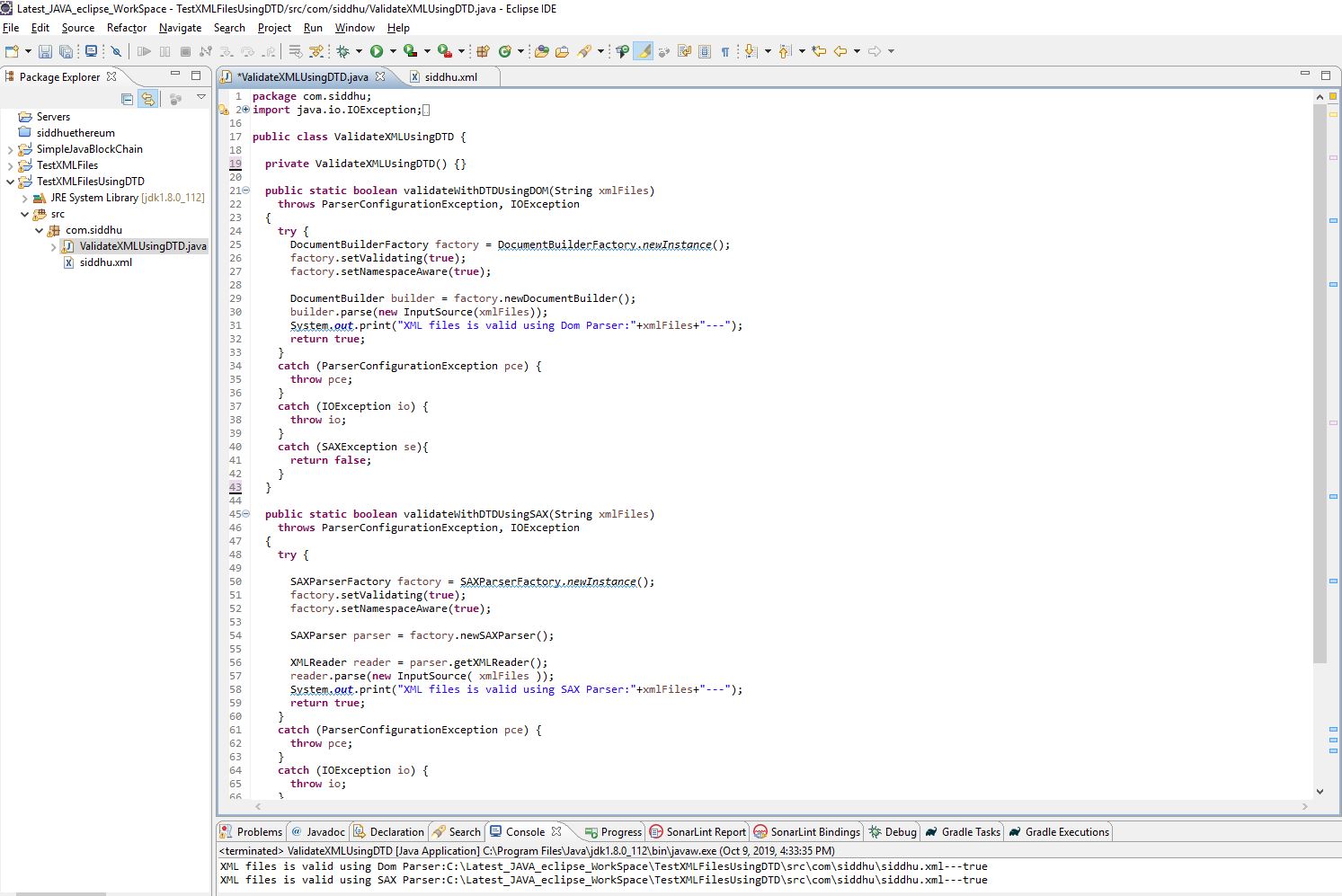
For in valid xml we will get below output
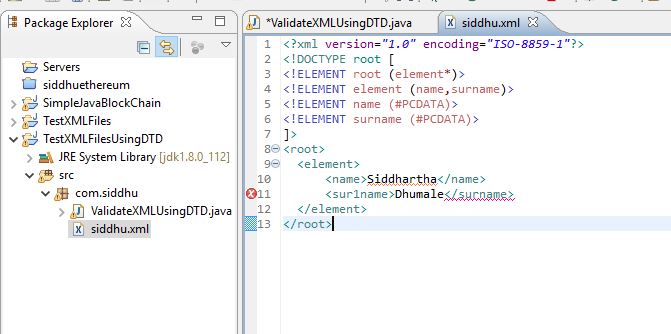
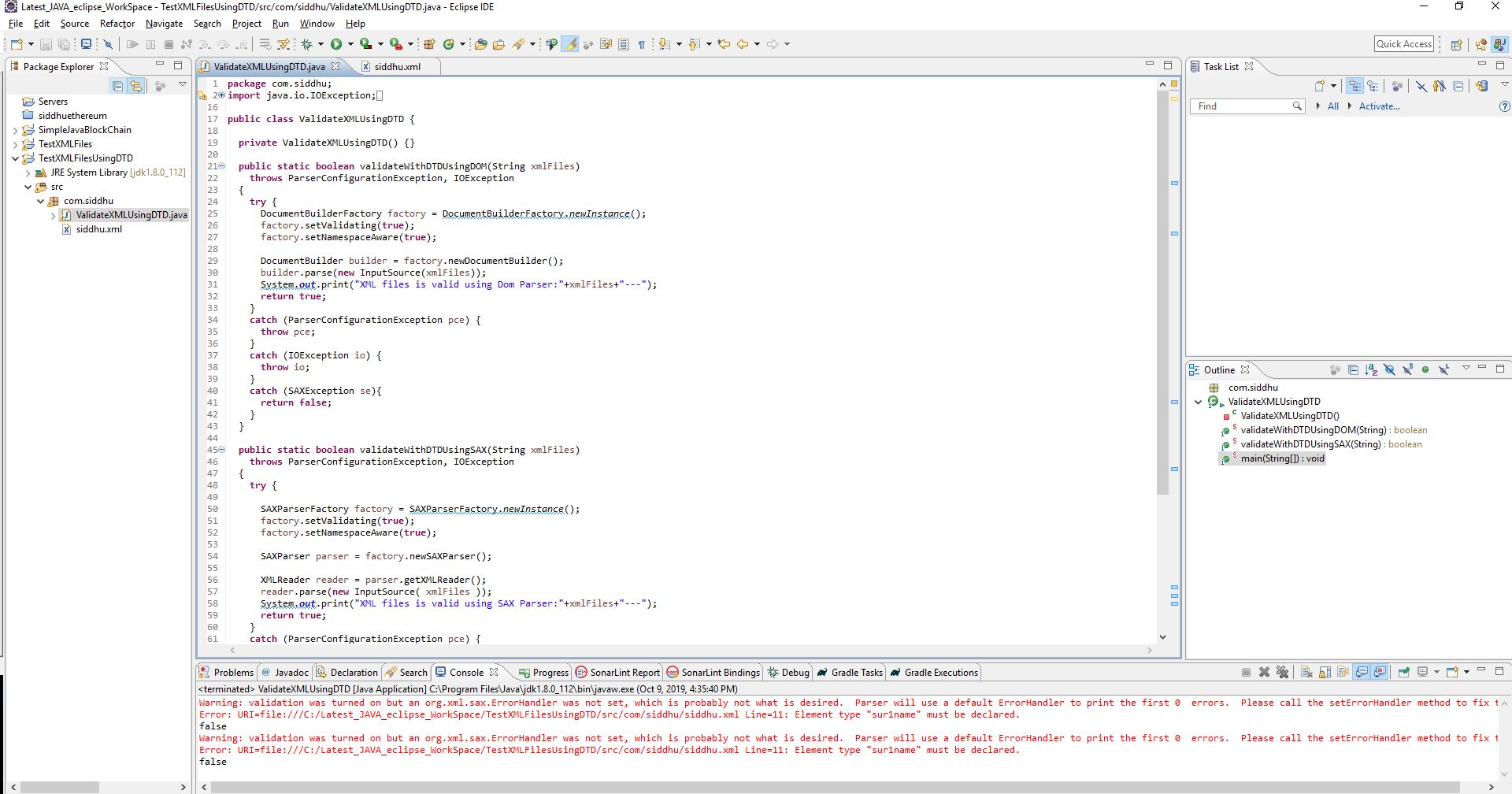
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE root [
<!ELEMENT root (element*)>
<!ELEMENT element (name,surname)>
<!ELEMENT name (#PCDATA)>
<!ELEMENT surname (#PCDATA)>
]>
<root>
<element>
<name>Siddhartha</name>
<surname>Dhumale</surname>
</element>
</root>
2- Following is the java class that is used to check if the given xml adhere to valid xsd file or not
package com.siddhu;
import java.io.IOException;
// DOM
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
// SAX
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.XMLReader;
import javax.xml.parsers.ParserConfigurationException;
import org.xml.sax.ErrorHandler;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.InputSource;
public class ValidateXMLUsingDTD {
private ValidateXMLUsingDTD() {}
public static boolean validateWithDTDUsingDOM(String xmlFiles)
throws ParserConfigurationException, IOException
{
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setValidating(true);
factory.setNamespaceAware(true);
DocumentBuilder builder = factory.newDocumentBuilder();
builder.parse(new InputSource(xmlFiles));
System.out.print("XML files is valid using Dom Parser:"+xmlFiles+"---");
return true;
}
catch (ParserConfigurationException pce) {
throw pce;
}
catch (IOException io) {
throw io;
}
catch (SAXException se){
return false;
}
}
public static boolean validateWithDTDUsingSAX(String xmlFiles)
throws ParserConfigurationException, IOException
{
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setValidating(true);
factory.setNamespaceAware(true);
SAXParser parser = factory.newSAXParser();
XMLReader reader = parser.getXMLReader();
reader.parse(new InputSource( xmlFiles ));
System.out.print("XML files is valid using SAX Parser:"+xmlFiles+"---");
return true;
}
catch (ParserConfigurationException pce) {
throw pce;
}
catch (IOException io) {
throw io;
}
catch (SAXException se){
return false;
}
}
public static void main (String args[]) throws Exception{
System.out.println(ValidateXMLUsingDTD.validateWithDTDUsingDOM("C:\\Latest_JAVA_eclipse_WorkSpace\\TestXMLFilesUsingDTD\\src\\com\\siddhu\\siddhu.xml"));
System.out.println(ValidateXMLUsingDTD.validateWithDTDUsingSAX("C:\\Latest_JAVA_eclipse_WorkSpace\\TestXMLFilesUsingDTD\\src\\com\\siddhu\\siddhu.xml"));
}
}
For valid xml we will get below output
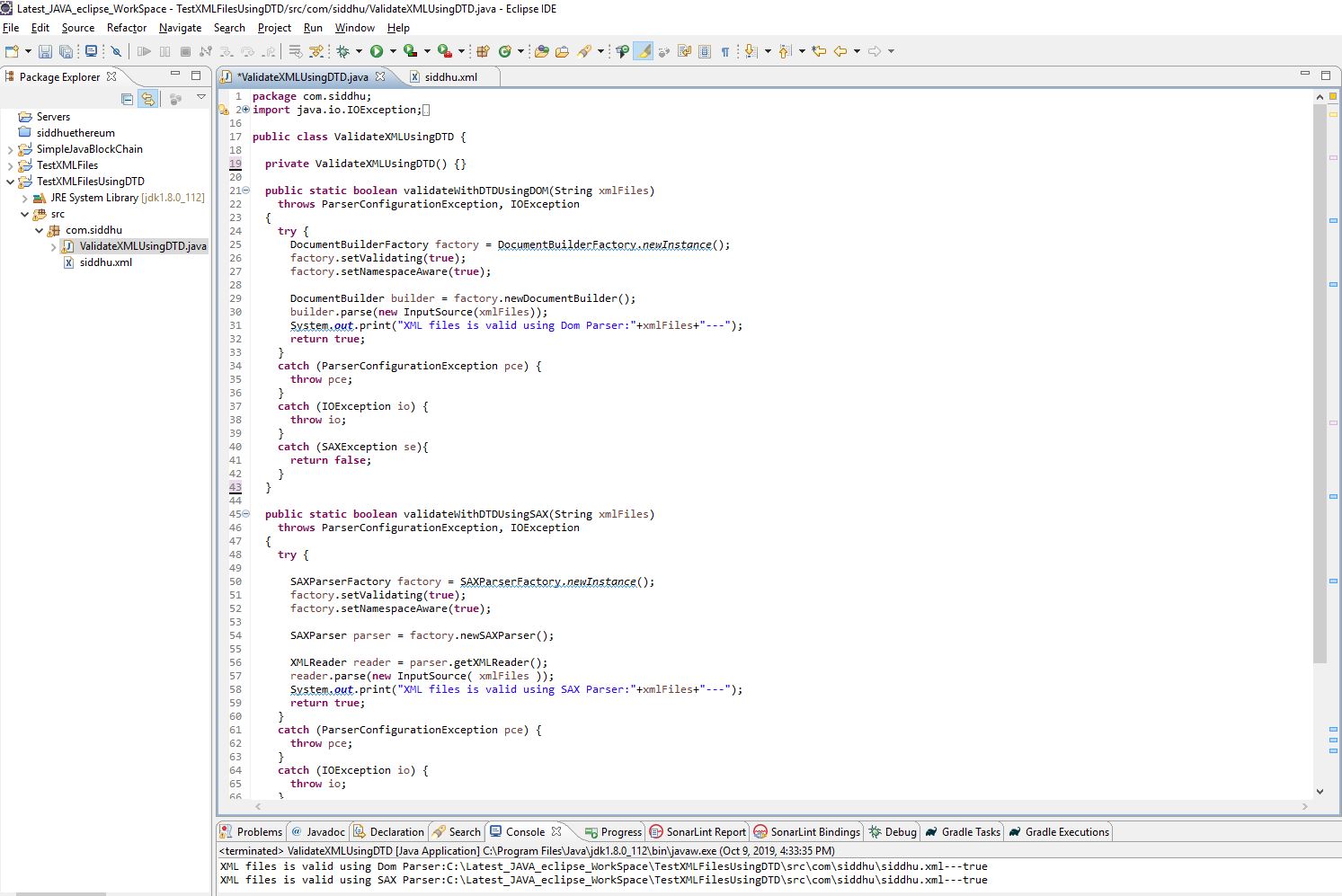
For in valid xml we will get below output
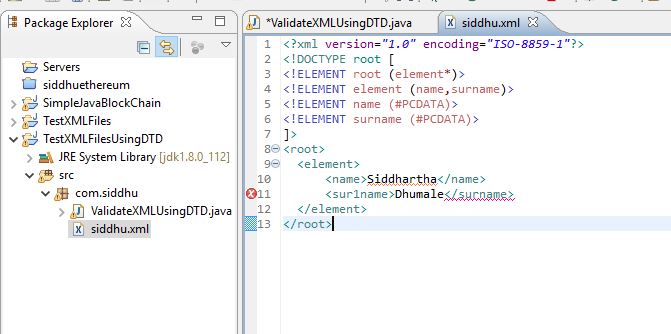
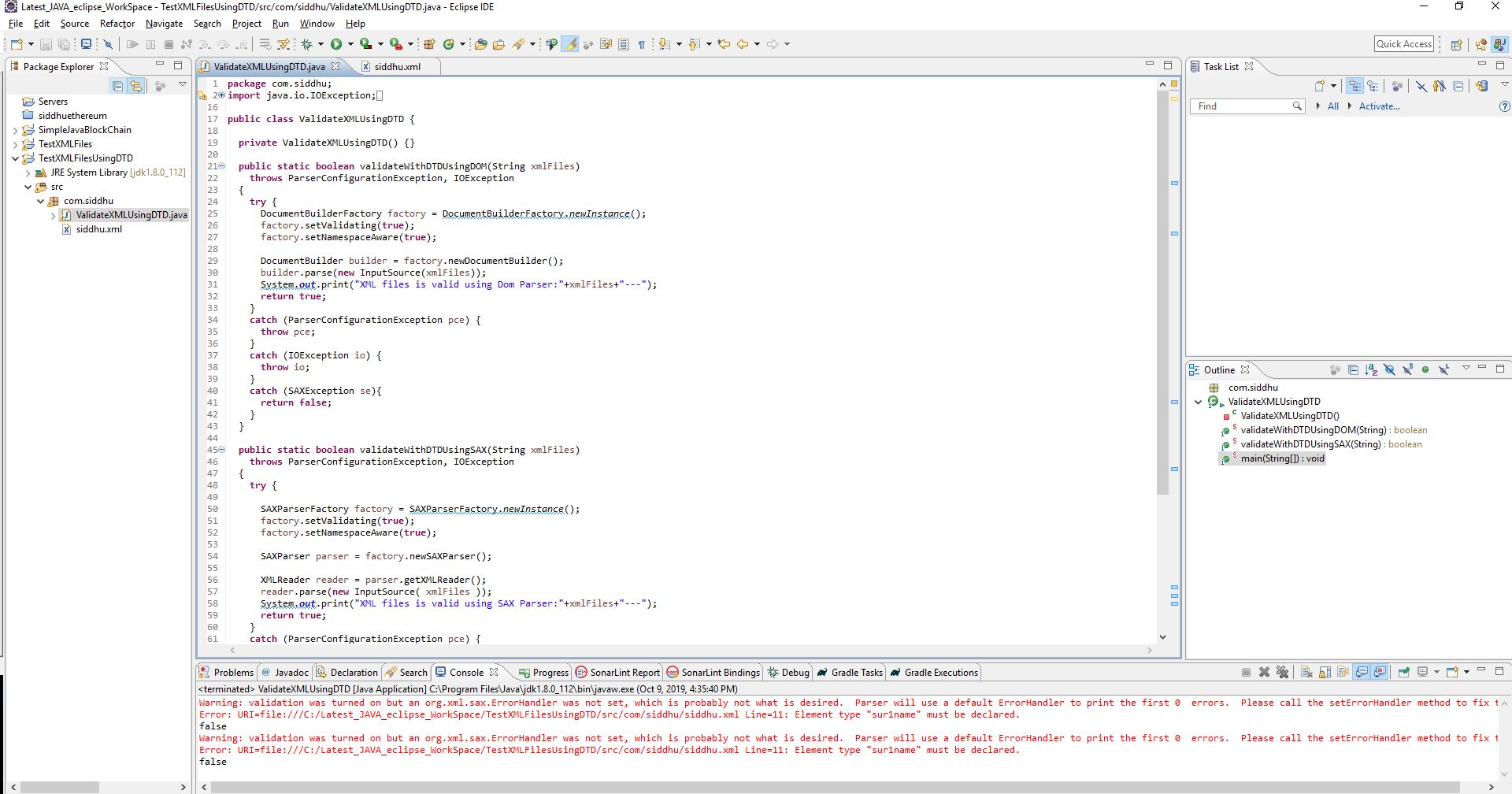