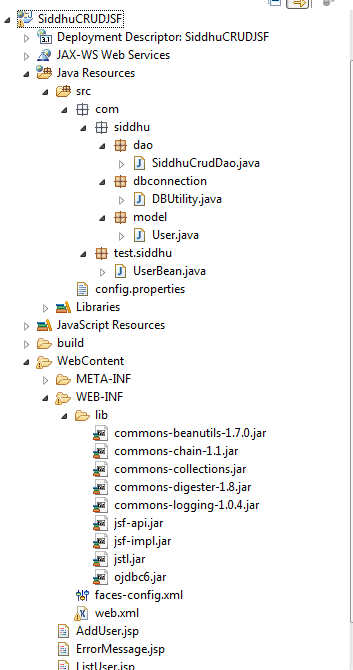
1:- SiddhuCrudDao
package com.siddhu.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.List;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.List;
import com.siddhu.dbconnection.DBUtility;
import com.siddhu.model.User;
public class SiddhuCrudDao {
import com.siddhu.model.User;
public class SiddhuCrudDao {
private Connection connection;
private int result;
private int result;
public SiddhuCrudDao() {
connection = DBUtility.getConnection();
}
connection = DBUtility.getConnection();
}
public int addUser(User user) {
try {
try {
PreparedStatement preparedStatement = connection
.prepareStatement("insert into \"SIDDHU\".\"USER\"(userid,firstname,lastname,email) values (?,?, ?, ? )");
preparedStatement.setInt(1, user.getUserid());
preparedStatement.setString(2, user.getFirstName());
preparedStatement.setString(3, user.getLastName());
preparedStatement.setString(4, user.getEmail());
result= preparedStatement.executeUpdate();
.prepareStatement("insert into \"SIDDHU\".\"USER\"(userid,firstname,lastname,email) values (?,?, ?, ? )");
preparedStatement.setInt(1, user.getUserid());
preparedStatement.setString(2, user.getFirstName());
preparedStatement.setString(3, user.getLastName());
preparedStatement.setString(4, user.getEmail());
result= preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
return result;
}
e.printStackTrace();
}
return result;
}
public int deleteUser(int userId) {
try {
PreparedStatement preparedStatement = connection
.prepareStatement("delete from USER where userid=?");
preparedStatement.setInt(1, userId);
result= preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
return result;
}
try {
PreparedStatement preparedStatement = connection
.prepareStatement("delete from USER where userid=?");
preparedStatement.setInt(1, userId);
result= preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
return result;
}
public int updateUser(User user) throws ParseException {
try {
PreparedStatement preparedStatement = connection
.prepareStatement("update USER set lastname=?,email=?" +
"where userid=?");
preparedStatement.setString(1, user.getLastName());
preparedStatement.setString(2, user.getEmail());
preparedStatement.setInt(3, user.getUserid());
result= preparedStatement.executeUpdate();
try {
PreparedStatement preparedStatement = connection
.prepareStatement("update USER set lastname=?,email=?" +
"where userid=?");
preparedStatement.setString(1, user.getLastName());
preparedStatement.setString(2, user.getEmail());
preparedStatement.setInt(3, user.getUserid());
result= preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
return result;
}
e.printStackTrace();
}
return result;
}
public List getAllUsers() {
List users = new ArrayList();
try {
Statement statement = connection.createStatement();
ResultSet rs = statement.executeQuery("select * from USER");
while (rs.next()) {
User user = new User();
user.setUserid(rs.getInt("userid"));
user.setFirstName(rs.getString("firstname"));
user.setLastName(rs.getString("lastname"));
user.setEmail(rs.getString("email"));
users.add(user);
}
} catch (SQLException e) {
e.printStackTrace();
}
List
try {
Statement statement = connection.createStatement();
ResultSet rs = statement.executeQuery("select * from USER");
while (rs.next()) {
User user = new User();
user.setUserid(rs.getInt("userid"));
user.setFirstName(rs.getString("firstname"));
user.setLastName(rs.getString("lastname"));
user.setEmail(rs.getString("email"));
users.add(user);
}
} catch (SQLException e) {
e.printStackTrace();
}
return users;
}
}
public User getUserById(int userId) {
User user = new User();
try {
PreparedStatement preparedStatement = connection.
prepareStatement("select * from USER where userid=?");
preparedStatement.setInt(1, userId);
ResultSet rs = preparedStatement.executeQuery();
User user = new User();
try {
PreparedStatement preparedStatement = connection.
prepareStatement("select * from USER where userid=?");
preparedStatement.setInt(1, userId);
ResultSet rs = preparedStatement.executeQuery();
if (rs.next()) {
user.setUserid(rs.getInt("userid"));
user.setFirstName(rs.getString("firstname"));
user.setLastName(rs.getString("lastname"));
user.setUserid(rs.getInt("userid"));
user.setFirstName(rs.getString("firstname"));
user.setLastName(rs.getString("lastname"));
user.setEmail(rs.getString("email"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return user;
}
}
} catch (SQLException e) {
e.printStackTrace();
}
return user;
}
public static void main(String args[])
{
SiddhuCrudDao objSiddhuCrudDao = new SiddhuCrudDao();
User user=new User();
user.setUserid(2);
user.setFirstName("Siddharatha");
user.setLastName("Dhumale");
user.setEmail("shdhumale@gmail.com");
objSiddhuCrudDao.addUser(user);
}
{
SiddhuCrudDao objSiddhuCrudDao = new SiddhuCrudDao();
User user=new User();
user.setUserid(2);
user.setFirstName("Siddharatha");
user.setLastName("Dhumale");
user.setEmail("shdhumale@gmail.com");
objSiddhuCrudDao.addUser(user);
}
}
2:- DBUtility
package com.siddhu.dbconnection;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
public class DBUtility {
private static Connection connection = null;
private static Connection connection = null;
public static Connection getConnection() {
if (connection != null)
return connection;
else {
try {
Properties prop = new Properties();
//InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("/config.properties");
//InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("config.properties");
InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("config.properties");
prop.load(inputStream);
String driver = prop.getProperty("driver");
String url = prop.getProperty("url");
String user = prop.getProperty("user");
String password = prop.getProperty("password");
Class.forName(driver);
connection = DriverManager.getConnection(url, user, password);
if (connection != null)
return connection;
else {
try {
Properties prop = new Properties();
//InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("/config.properties");
//InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("config.properties");
InputStream inputStream = DBUtility.class.getClassLoader().getResourceAsStream("config.properties");
prop.load(inputStream);
String driver = prop.getProperty("driver");
String url = prop.getProperty("url");
String user = prop.getProperty("user");
String password = prop.getProperty("password");
Class.forName(driver);
connection = DriverManager.getConnection(url, user, password);
/*// Store the database URL in a string
String serverName = "127.0.0.1";
String portNumber = "1521";
String sid = "XE";
String dbUrl = "jdbc:oracle:thin:@" + serverName + ":" + portNumber
+ ":" + sid;
String serverName = "127.0.0.1";
String portNumber = "1521";
String sid = "XE";
String dbUrl = "jdbc:oracle:thin:@" + serverName + ":" + portNumber
+ ":" + sid;
Class.forName("oracle.jdbc.driver.OracleDriver");
// set the url, username and password for the database
connection = DriverManager.getConnection(dbUrl, "siddhu", "siddhu"); */
// set the url, username and password for the database
connection = DriverManager.getConnection(dbUrl, "siddhu", "siddhu"); */
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return connection;
}
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return connection;
}
}
}
3:- User
package com.siddhu.model;
public class User {
public class User {
private int userid;
private String firstName;
private String lastName;
private String email;
private String firstName;
private String lastName;
private String email;
public int getUserid() {
return userid;
}
public void setUserid(int userid) {
this.userid = userid;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
return userid;
}
public void setUserid(int userid) {
this.userid = userid;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "User [userid=" + userid + ", firstName=" + firstName
+ ", lastName=" + lastName + ", email="
+ email + "]";
}
}
4:- UserBean
package com.test.siddhu;
import com.siddhu.dao.SiddhuCrudDao;
import com.siddhu.model.User;
import com.siddhu.model.User;
public class UserBean {
private int userID;
private String firstName;
private String lastName;
private String email;
private String firstName;
private String lastName;
private String email;
private SiddhuCrudDao dao;
//This is Action method to add user
public String addUser() {
dao=new SiddhuCrudDao();
User user=new User();
user.setUserid(this.getUserID());
user.setFirstName(this.getFirstName());
user.setLastName(this.getLastName());
user.setEmail(this.getEmail());
//dao.addUser(user);
if (dao.addUser(user)>0)
return "success";
else
return "failure";
public String addUser() {
dao=new SiddhuCrudDao();
User user=new User();
user.setUserid(this.getUserID());
user.setFirstName(this.getFirstName());
user.setLastName(this.getLastName());
user.setEmail(this.getEmail());
//dao.addUser(user);
if (dao.addUser(user)>0)
return "success";
else
return "failure";
}
public int getUserID() {
return userID;
}
return userID;
}
public void setUserID(int userID) {
this.userID = userID;
}
this.userID = userID;
}
public String getFirstName() {
return firstName;
}
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
this.lastName = lastName;
}
public String getEmail() {
return email;
}
return email;
}
public void setEmail(String email) {
this.email = email;
}
this.email = email;
}
public static void main(String args[])
{
UserBean objUserBean = new UserBean();
//User user=new User();
objUserBean.setUserID(2);
objUserBean.setFirstName("Siddharatha");
objUserBean.setLastName("Dhumale");
objUserBean.setEmail("shdhumale@gmail.com");
objUserBean.addUser();
}
}
5:- faces-config.xml
6:- web.xml
7:- AddUser.jsp
8:- ErrorMessage.jsp
9:- ListUser.jsp