Generally we use to Use JERSY in java to perform JSON REST call and then using traditional way we use to parse the output to get desired result.
Rest assured is best API available in its kind to do the same. It support all REST operation like GET/PUT/POST etc.
http://rest-assured.io/
Best use of rest assured is its well tested API to parse JSON response and get desired value.
Please find below given POC for the same
1- POM.xml
<!--project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<!--modelVersion>4.0.0<!--/modelVersion>
<!--groupId>com.rest.assured<!--/groupId>
<!--artifactId>com.rest.assured.test<!--/artifactId>
<!--version>0.0.1-SNAPSHOT<!--/version>
<!--packaging>jar<!--/packaging>
<!--name>com.rest.assured.test<!--/name>
<!--url>http://maven.apache.org<!--/url>
<!--properties>
<!--project.build.sourceEncoding>UTF-8<!--/project.build.sourceEncoding>
<!--/properties>
<!--dependencies>
<!--dependency>
<!--groupId>junit<!--/groupId>
<!--artifactId>junit<!--/artifactId>
<!--version>4.12<!--/version>
<!--scope>test<!--/scope>
<!--/dependency>
<!--dependency>
<!--groupId>io.rest-assured<!--/groupId>
<!--artifactId>rest-assured<!--/artifactId>
<!--version>3.0.1<!--/version>
<!--scope>test<!--/scope>
<!--/dependency>
<!--dependency>
<!--groupId>io.rest-assured<!--/groupId>
<!--artifactId>json-path<!--/artifactId>
<!--version>3.0.1<!--/version>
<!--/dependency>
<!--dependency>
<!--groupId>io.rest-assured<!--/groupId>
<!--artifactId>xml-path<!--/artifactId>
<!--version>3.0.1<!--/version>
<!--/dependency>
<!--dependency>
<!--groupId>io.rest-assured<!--/groupId>
<!--artifactId>json-schema-validator<!--/artifactId>
<!--version>3.0.1<!--/version>
<!--scope>test<!--/scope>
<!--/dependency>
<!--dependency>
<!--groupId>org.apache.commons<!--/groupId>
<!--artifactId>commons-lang3<!--/artifactId>
<!--version>3.4<!--/version>
<!--/dependency>
<!--dependency>
<!--groupId>org.codehaus.groovy<!--/groupId>
<!--artifactId>groovy-json<!--/artifactId>
<!--version>2.4.6<!--/version>
<!--/dependency>
<!--dependency>
<!--groupId>com.oracle<!--/groupId>
<!--artifactId>ojdbc6<!--/artifactId>
<!--version>11.2.0.2.0<!--/version>
<!--/dependency>
<!--/dependencies>
<!--/project>
2- TestRestAssured
import static io.restassured.RestAssured.given;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import io.restassured.response.ValidatableResponse;
import static io.restassured.RestAssured.given;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import io.restassured.response.ValidatableResponse;
import org.junit.Test;
public class TestRestAssured {
@Test
public void makeSureThatGoogleIsUp() {
//given().when().get("http://services.groupkt.com/country/get/iso2code/IN").then().statusCode(200);
/*
* //To check if result value in array is India
//given().when().get("http://services.groupkt.com/country/get/iso2code/IN").then().statusCode(200).body("RestResponse.result.name", equalTo("India"));
*/
public void makeSureThatGoogleIsUp() {
//given().when().get("http://services.groupkt.com/country/get/iso2code/IN").then().statusCode(200);
/*
* //To check if result value in array is India
//given().when().get("http://services.groupkt.com/country/get/iso2code/IN").then().statusCode(200).body("RestResponse.result.name", equalTo("India"));
*/
//ValidatableResponse objValidatableResponse = given().when().get("http://services.groupkt.com/country/get/iso2code/IN").then().statusCode(200);
/*
* //To check response contain perticular string
Response response = given().when().get("http://services.groupkt.com/country/get/iso2code/IN");
JsonPath jsonPath = new JsonPath(response.getBody().asString());
String user_id = jsonPath.getString("RestResponse.result.name");
*/
/*
* //To check if response is 200
Response response = given().when().get("http://services.groupkt.com/country/get/iso2code/IN");
Long statusCode = new Long(response.statusCode());
Long id = null;
if(statusCode.toString().equals("200")){
* //To check response contain perticular string
Response response = given().when().get("http://services.groupkt.com/country/get/iso2code/IN");
JsonPath jsonPath = new JsonPath(response.getBody().asString());
String user_id = jsonPath.getString("RestResponse.result.name");
*/
/*
* //To check if response is 200
Response response = given().when().get("http://services.groupkt.com/country/get/iso2code/IN");
Long statusCode = new Long(response.statusCode());
Long id = null;
if(statusCode.toString().equals("200")){
System.out.println("This is test");
//make a db call
}*/
//make a db call
}*/
}
}
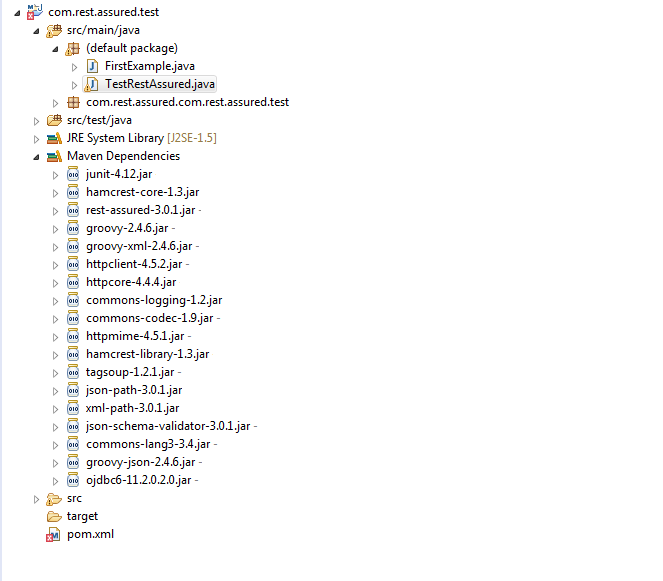
For Reference
http://testdetective.com/rest-assured-framework-overview/
https://semaphoreci.com/community/tutorials/testing-rest-endpoints-using-rest-assured
https://github.com/rest-assured/rest-assured/wiki/GettingStarted
https://github.com/rest-assured/rest-assured/wiki/GettingStarted#jsonpath
https://github.com/rest-assured/rest-assured/wiki/Usage
https://github.com/rest-assured/rest-assured/wiki/Downloads
http://james-willett.com/2015/06/extracting-a-json-response-with-rest-assured/
No comments:
Post a Comment