This example show you how to call REST JSON response with AngularJS2
In this example we are using Eclipse IDE and REST JSON service from
http://services.groupkt.com/country/get/all
http://services.groupkt.com/country/get/iso2code/IN
http://services.groupkt.com/country/get/all
http://services.groupkt.com/country/get/iso2code/IN
Step 1:- Create an angularJS2 project in eclipse
right click --> New --> Other-->AngularJs2 project--> Give the name of the project
Above step will create and base project with node-module folder inside our project.
Step 2:- Lets create an service which is used to make an REST Call i.e. siddhu-rest-service.service.ts
In code we are using the concept of observable and map so import following below line inside the code.
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/map';
we are expecting the result in the form of JSON
getAllCountry(){
return this._http.get(this.getAllUrl)
.map(response => response.json());
}
Step 3:- Add newly created service inside at app.modules.ts so that it can be available to whole project
return this._http.get(this.getAllUrl)
.map(response => response.json());
}
Step 3:- Add newly created service inside at app.modules.ts so that it can be available to whole project
import { SiddhuRestServiceService } from './siddhu-rest-service.service';
providers: [SiddhuRestServiceService],
providers: [SiddhuRestServiceService],
Step 4:- Modfiy html file to show the JSON response on the screen
<!--button (click)="getAllCountry()">Call Country Code Web Service<!--/button>
<!--label>The result after Post<!--/label> {{getJSONResponse}}
<!--div>
<!--label>Country Code: <!--/label> <!--input [(ngModel)]="countrycode"
placeholder="countrycode" />
<!--/div>
<!--button (click)="getIsoofCountry(countrycode)">Call Country Code Web Service<!--/button>
<!--div>
<!--label>REST Response of http://services.groupkt.com/country/get/iso2code: <!--/label>
{{ getCountryCodeJSONResponse }}
<!--/div>
<!--label>The result after Post<!--/label> {{getJSONResponse}}
<!--div>
<!--label>Country Code: <!--/label> <!--input [(ngModel)]="countrycode"
placeholder="countrycode" />
<!--/div>
<!--button (click)="getIsoofCountry(countrycode)">Call Country Code Web Service<!--/button>
<!--div>
<!--label>REST Response of http://services.groupkt.com/country/get/iso2code: <!--/label>
{{ getCountryCodeJSONResponse }}
<!--/div>
<!--/div>
2:- siddhu-rest-service.service.ts
import { Injectable } from '@angular/core';
import { Headers, Http } from '@angular/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/toPromise';
import { Headers, Http } from '@angular/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/toPromise';
@Injectable()
export class SiddhuRestServiceService {
private headers = new Headers({'Content-Type': 'application/json'});
private getAllUrl = 'http://services.groupkt.com/country/get/all'; // URL to web api
private getISOCode = 'http://services.groupkt.com/country/get/iso2code';
constructor(private _http: Http) {
}
// getAllCountry(): Promise<{}> {
getAllCountry(){
/* console.log(this._http.get(this.getAllUrl)
.toPromise()
.then(response => response.json())
.catch(this.handleError));*/
// .map(response => response.json()));
/* return this._http.get(this.getAllUrl)
.toPromise()
.then(response => response.json().data)
.catch(this.handleError);*/
return this._http.get(this.getAllUrl)
.map(response => response.json());
}
// getIsoofCountry(id: string): Promise<{}> {
getIsoofCountry(id: string) {
const url = `${this.getISOCode}/${id}`;
/* console.log(this._http.get(url)
.toPromise()
.then(response => response.json())
.catch(this.handleError));*/
/*return this._http.get(url)
.toPromise()
.then(response => response.json().data)
.catch(this.handleError);*/
return this._http.get(url)
.map(response => response.json());
}
private handleError(error: any) {
console.error(error);
return Observable.throw(error.json().error || 'Server error');
}
}
3:- app.component.ts
import { Component } from '@angular/core';
import { SiddhuRestServiceService } from './siddhu-rest-service.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
getJSONResponse:string;
getCountryCodeJSONResponse:string;
getCountryCodeJSONResponse:string;
constructor(private siddhurestservice: SiddhuRestServiceService) { }
title = 'Calling REST Web service using AngularJS2';
getAllCountry() {
this.siddhurestservice
.getAllCountry().subscribe(
data => this.getJSONResponse = JSON.stringify(data), // put the data returned from the server in our variable
error => console.log("Error HTTP GET Service"), // in case of failure show this message
() => console.log("Job Done Get !")//run this code in all cases
);
}
this.siddhurestservice
.getAllCountry().subscribe(
data => this.getJSONResponse = JSON.stringify(data), // put the data returned from the server in our variable
error => console.log("Error HTTP GET Service"), // in case of failure show this message
() => console.log("Job Done Get !")//run this code in all cases
);
}
getIsoofCountry(id : string) {
console.log(id);
this.siddhurestservice
.getIsoofCountry(id).subscribe(
data => this.getCountryCodeJSONResponse = JSON.stringify(data), // put the data returned from the server in our variable
error => console.log("Error HTTP GET Service"), // in case of failure show this message
() => console.log("Job Done Get !")//run this code in all cases
);
}
console.log(id);
this.siddhurestservice
.getIsoofCountry(id).subscribe(
data => this.getCountryCodeJSONResponse = JSON.stringify(data), // put the data returned from the server in our variable
error => console.log("Error HTTP GET Service"), // in case of failure show this message
() => console.log("Job Done Get !")//run this code in all cases
);
}
}
4:- app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpModule } from '@angular/http';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpModule } from '@angular/http';
import { AppComponent } from './app.component';
import { SiddhuRestServiceService } from './siddhu-rest-service.service';
import { SiddhuRestServiceService } from './siddhu-rest-service.service';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule,
HttpModule
],
providers: [SiddhuRestServiceService],
bootstrap: [AppComponent]
})
export class AppModule { }
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule,
HttpModule
],
providers: [SiddhuRestServiceService],
bootstrap: [AppComponent]
})
export class AppModule { }
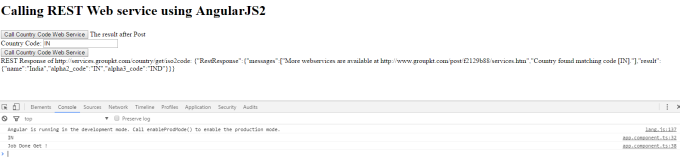

No comments:
Post a Comment