Let say we have following Service.ts file
import { Injectable } from '@angular/core';
import { Http, Response, RequestOptions, Headers } from '@angular/http';
@Injectable()
export class MyServiceService {
apiRoot: string = "http://httpbin.org";
constructor(private http: Http) {
}
getData()
{
let url = `${this.apiRoot}/get`;
return this.http.get(url);
}
}
In above service we are using Http to have the spec.ts file for this use below code
/* tslint:disable:no-unused-variable */
import { TestBed, async, inject } from '@angular/core/testing';
import { MyServiceService } from './my-service.service';
import { HttpModule } from '@angular/http';
describe('Service: MyService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
// HttpModule is imported because we are using this module in service to make http call.
imports : [ HttpModule ],
// We need to provide which service we are going to test or used in testing
providers: [MyServiceService]
});
});
it('should ...', inject([MyServiceService], (service: MyServiceService) => {
//create the instance of service method as shown below
let a = service.getData();
console.log('==================='+ a);
expect(service).toBeTruthy();
}));
});
Now lets say we want to test the AppComponent class for the same service method
import { Component } from '@angular/core';
import { MyServiceService } from './my-service.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
myArrayData=[];
constructor(private myServiceService: MyServiceService) {
}
doGET() {
console.log("GET");
this.myServiceService.getData().subscribe(
(data: any) => {
//data = JSON.parse(data['body']);
console.log("data",data);
this.myArrayData = data;
console.log("<<<<<<<<<<<>>>>>>>>>>>>>>>>>"+this.myArrayData);
},
err => console.log(err), // error
() => console.log('getData Complete') // complete
);
}
doPOST() {
console.log("POST");
}
doPUT() {
console.log("PUT");
}
doDELETE() {
console.log("DELETE");
}
doGETAsPromise() {
console.log("GET AS PROMISE");
}
doGETAsPromiseError() {
console.log("GET AS PROMISE ERROR");
}
doGETAsObservableError() {
console.log("GET AS OBSERVABLE ERROR");
}
doGETWithHeaders() {
console.log("GET WITH HEADERS");
}
}
Our spec.ts file will be
import { AppComponent } from './app.component';
import { MyServiceService } from './my-service.service';
import { HttpModule } from '@angular/http';
import { TestBed, async, inject } from '@angular/core/testing';
describe('AppComponent : appComponent', () => {
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [
AppComponent
],
//we need to import HttpModule as we are using this module in service.ts file
imports : [ HttpModule ],
//we need to provide two ts file orignial name for which we are going to write the test
providers: [MyServiceService, AppComponent]
}).compileComponents();
}));
it('should ...', inject([MyServiceService , AppComponent], (service: MyServiceService, appComponent : AppComponent) => {
//Creating the object to call the method.
let a = appComponent.doGET();
console.log('>>>>>>>>>>>>>>>>>>>'+ a);
expect(appComponent).toBeTruthy();
}));
});

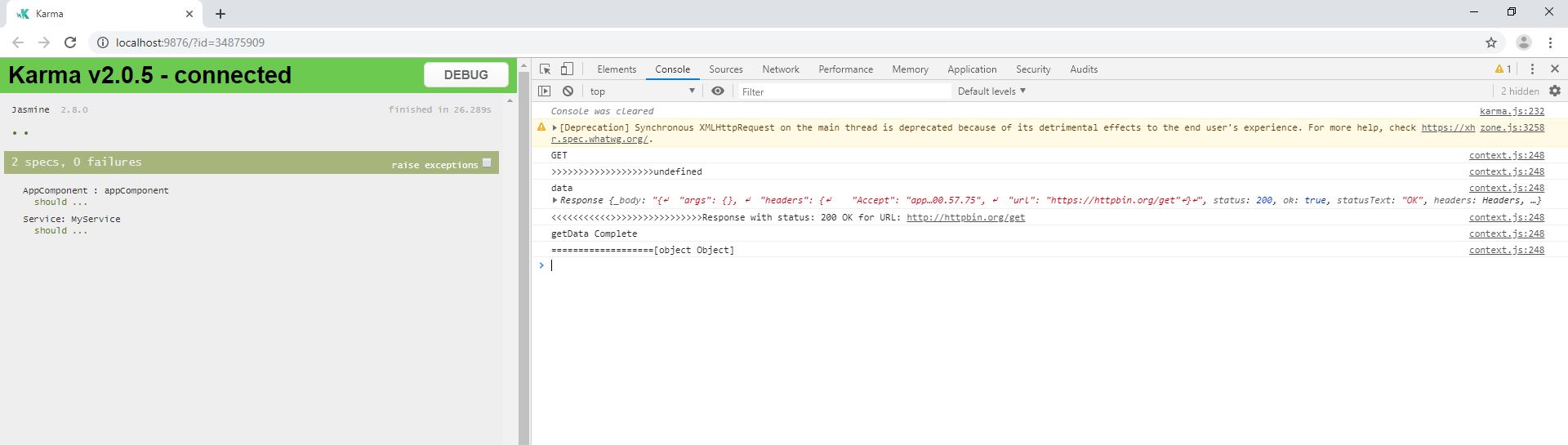
import { Injectable } from '@angular/core';
import { Http, Response, RequestOptions, Headers } from '@angular/http';
@Injectable()
export class MyServiceService {
apiRoot: string = "http://httpbin.org";
constructor(private http: Http) {
}
getData()
{
let url = `${this.apiRoot}/get`;
return this.http.get(url);
}
}
In above service we are using Http to have the spec.ts file for this use below code
/* tslint:disable:no-unused-variable */
import { TestBed, async, inject } from '@angular/core/testing';
import { MyServiceService } from './my-service.service';
import { HttpModule } from '@angular/http';
describe('Service: MyService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
// HttpModule is imported because we are using this module in service to make http call.
imports : [ HttpModule ],
// We need to provide which service we are going to test or used in testing
providers: [MyServiceService]
});
});
it('should ...', inject([MyServiceService], (service: MyServiceService) => {
//create the instance of service method as shown below
let a = service.getData();
console.log('==================='+ a);
expect(service).toBeTruthy();
}));
});
Now lets say we want to test the AppComponent class for the same service method
import { Component } from '@angular/core';
import { MyServiceService } from './my-service.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
myArrayData=[];
constructor(private myServiceService: MyServiceService) {
}
doGET() {
console.log("GET");
this.myServiceService.getData().subscribe(
(data: any) => {
//data = JSON.parse(data['body']);
console.log("data",data);
this.myArrayData = data;
console.log("<<<<<<<<<<<>>>>>>>>>>>>>>>>>"+this.myArrayData);
},
err => console.log(err), // error
() => console.log('getData Complete') // complete
);
}
doPOST() {
console.log("POST");
}
doPUT() {
console.log("PUT");
}
doDELETE() {
console.log("DELETE");
}
doGETAsPromise() {
console.log("GET AS PROMISE");
}
doGETAsPromiseError() {
console.log("GET AS PROMISE ERROR");
}
doGETAsObservableError() {
console.log("GET AS OBSERVABLE ERROR");
}
doGETWithHeaders() {
console.log("GET WITH HEADERS");
}
}
Our spec.ts file will be
import { AppComponent } from './app.component';
import { MyServiceService } from './my-service.service';
import { HttpModule } from '@angular/http';
import { TestBed, async, inject } from '@angular/core/testing';
describe('AppComponent : appComponent', () => {
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [
AppComponent
],
//we need to import HttpModule as we are using this module in service.ts file
imports : [ HttpModule ],
//we need to provide two ts file orignial name for which we are going to write the test
providers: [MyServiceService, AppComponent]
}).compileComponents();
}));
it('should ...', inject([MyServiceService , AppComponent], (service: MyServiceService, appComponent : AppComponent) => {
//Creating the object to call the method.
let a = appComponent.doGET();
console.log('>>>>>>>>>>>>>>>>>>>'+ a);
expect(appComponent).toBeTruthy();
}));
});

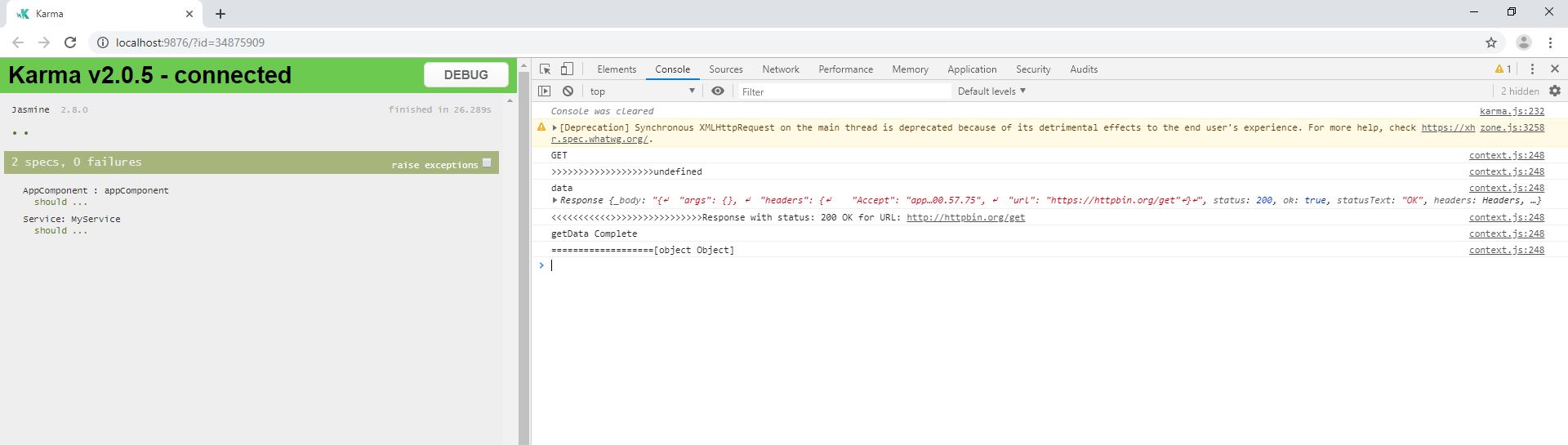
No comments:
Post a Comment