Django comes with its own concept of developing web application using Model of the Table as base.
Basic steps involved in creating a CRUD app in Django are as follows:-
1- Create an Django Web Application base folder structure. We are using Eclipse with PyDev. For more details refer to below site: https://shdhumale.wordpress.com/2017/03/03/simple-django-hello-world-programe-using-eclipse-python-3-5-and-django-1-10/
2- Create the Model class. This model class is responsible for creation of Table in our Database.
3- Create the View logic that we are planning to display to the end user
4- Declare and define the URLs which is going to map our view part. This url is resposible to divert the call to specific python class and html page depending on our relam or browser url.
5- Create the Templates where we put all our html file that we are planning to display to the end user.i.e. html file.
2- Create the Model class. This model class is responsible for creation of Table in our Database.
3- Create the View logic that we are planning to display to the end user
4- Declare and define the URLs which is going to map our view part. This url is resposible to divert the call to specific python class and html page depending on our relam or browser url.
5- Create the Templates where we put all our html file that we are planning to display to the end user.i.e. html file.
Step 1:- Creating an Django application. We can do this using CLI command i.e. ./manage.py startapp servers or use Eclipse process to create the Django Projects. Files marked in red colour will not be created by our eclipse plugin.
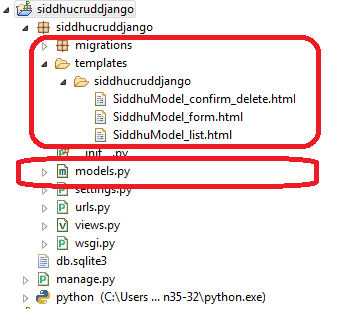
Step 2:- Add our newly created project entry in settings.py
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'siddhucruddjango',
]
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'siddhucruddjango',
]
Step 3:- Create Model class.
This class represent our Database Table. Create Model.py class inside our project.
from django.db import models
from django.core.urlresolvers import reverse
from django.core.urlresolvers import reverse
class SiddhuModel(models.Model):
name = models.CharField(max_length=200)
address = models.CharField(max_length=200)
emailid = models.CharField(max_length=20)
name = models.CharField(max_length=200)
address = models.CharField(max_length=200)
emailid = models.CharField(max_length=20)
def __unicode__(self):
return self.name
return self.name
def get_absolute_url(self):
return reverse('siddhu_update', kwargs={'pk': self.pk})
In Django Primary key is mandatory to have in table. If we did not define it frame work will automatically create an ID field for the same in table for us. In above python we had define a class SiddhuModel. When we execute this class using below command we will see migration folder created inside our project with files like __init__.py and 0001_initial.py.
return reverse('siddhu_update', kwargs={'pk': self.pk})
In Django Primary key is mandatory to have in table. If we did not define it frame work will automatically create an ID field for the same in table for us. In above python we had define a class SiddhuModel. When we execute this class using below command we will see migration folder created inside our project with files like __init__.py and 0001_initial.py.
./manage.py makemigrations
./manage.py migrate
./manage.py migrate
Try to analysis the code created inside 0001_initial.py.
operations = [
migrations.CreateModel(
name='SiddhuModel',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=200)),
('address', models.CharField(max_length=200)),
('emailid', models.CharField(max_length=20)),
],
),
Above code indicate when this files is executed it will create a table called siddhumodel with following four field. id, name, address and emailid.
migrations.CreateModel(
name='SiddhuModel',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=200)),
('address', models.CharField(max_length=200)),
('emailid', models.CharField(max_length=20)),
],
),
Above code indicate when this files is executed it will create a table called siddhumodel with following four field. id, name, address and emailid.
As we are using SQLite as database, I prefer to use SQLite Studio to see the database and its table.
You can download SQLite from https://sqlitestudio.pl/index.rvt.
our model.py will create follwing table in our database.
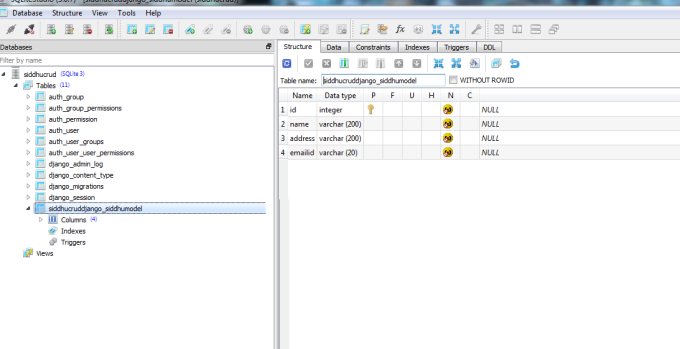
Step 3:- Modify our View logic for displaying the data to the end users.
Create a views.py file inside our project and place following code. Note:- We are using Class-based views.
from django.http import HttpResponse
from django.views.generic import TemplateView,ListView
from django.views.generic.edit import CreateView, UpdateView, DeleteView
from django.core.urlresolvers import reverse_lazy
from django.views.generic import TemplateView,ListView
from django.views.generic.edit import CreateView, UpdateView, DeleteView
from django.core.urlresolvers import reverse_lazy
from siddhucruddjango.models import SiddhuModel
class SiddhuList(ListView):
model = SiddhuModel
model = SiddhuModel
class SiddhuInsert(CreateView):
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
fields = ['name', 'address', 'emailid']
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
fields = ['name', 'address', 'emailid']
class SiddhuUpdate(UpdateView):
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
fields = ['name', 'address', 'emailid']
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
fields = ['name', 'address', 'emailid']
class SiddhuDelete(DeleteView):
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
model = SiddhuModel
success_url = reverse_lazy('siddhu_list')
Step 4:- Modify URLS for navigations.
Change urls.py with below code.
from django.conf.urls import url
from django.contrib import admin
from siddhucruddjango import views
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^$', views.SiddhuList.as_view(), name='siddhu_list'),
url(r'^insert$', views.SiddhuInsert.as_view(), name='siddhu_insert'),
url(r'^update/(?P
url(r'^delete/(?P
]
Step 5:- Develop UI HTML Part
Create a template folder inside our project. Create another folder with siddhucruddjango inside template and keep all our ui part files inside it.
and open the url the screen http://127.0.0.1:8000/

Click on Click to Create New Data link and follwing screen will be displayed
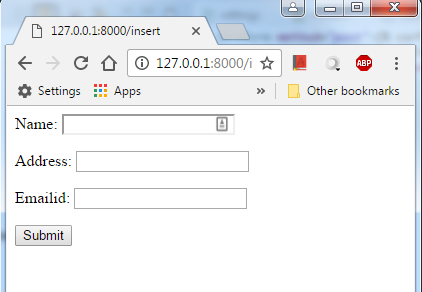
Fill the data and click on submit and check data is enter in our sqlite db.

Click on delete option and you will get following screen submit the button and check data is deleted from screen and databae.
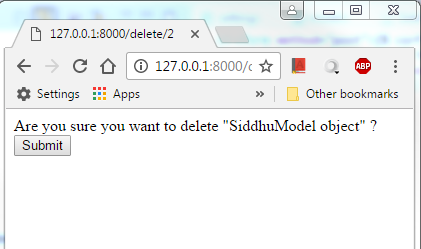
1- urls.py
from django.conf.urls import url
from django.contrib import admin
from siddhucruddjango import views
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^$', views.SiddhuList, name='siddhu_list'),
url(r'^insert$', views.SiddhuInsert, name='siddhu_insert'),
url(r'^update/(?P
url(r'^delete/(?P
]
2- views.py
from django.shortcuts import render, redirect, get_object_or_404
from django.forms import ModelForm
from siddhucruddjango.models import SiddhuModel
class ServerForm(ModelForm):
class Meta:
model = SiddhuModel
fields = ['name', 'address', 'emailid']
def SiddhuList(request, template_name='siddhucruddjango/SiddhuModel_list.html'):
servers = SiddhuModel.objects.all()
data = {}
data['object_list'] = servers
return render(request, template_name, data)
def SiddhuInsert(request, template_name='siddhucruddjango/SiddhuModel_form.html'):
form = ServerForm(request.POST or None)
if form.is_valid():
form.save()
return redirect('siddhu_list')
return render(request, template_name, {'form':form})
def SiddhuUpdate(request, pk, template_name='siddhucruddjango/SiddhuModel_form.html'):
server = get_object_or_404(SiddhuModel, pk=pk)
form = ServerForm(request.POST or None, instance=server)
if form.is_valid():
form.save()
return redirect('siddhu_list')
return render(request, template_name, {'form':form})
def SiddhuDelete(request, pk, template_name='siddhucruddjango/SiddhuModel_confirm_delete.html'):
server = get_object_or_404(SiddhuModel, pk=pk)
if request.method == 'POST':
server.delete()
return redirect('siddhu_list')
return render(request, template_name, {'object':server})
No comments:
Post a Comment