First install the camunda plarform using below link
1-First install JDK15 or above
2-Second install Camunda Paltform I am using 7 version
https://downloads.camunda.cloud/release/camunda-bpm/run/7.18/camunda-bpm-run-7.18.0.zip
3- Start up Camunda
Run start.bat (Windows)
4- Open http://localhost:8080/camunda-welcome/index.html and play with the Camunda webapps Cockpit and Tasklist.
5- Install Camunda modeler and exeute application icon.
https://camunda.com/download/modeler/
https://downloads.camunda.cloud/release/camunda-modeler/5.9.0/camunda-modeler-5.9.0-win-x64.zip
Now lets try to call java class from camunda task/process. i.e. calling Java Class from a BPMN 2.0 Service Task.
The above concept can be done by many ways as given below:-

we will see here two ways
1- External
2- Delegate
Lets try the first
1- External
We will create a simple process diagram and create a new service task.


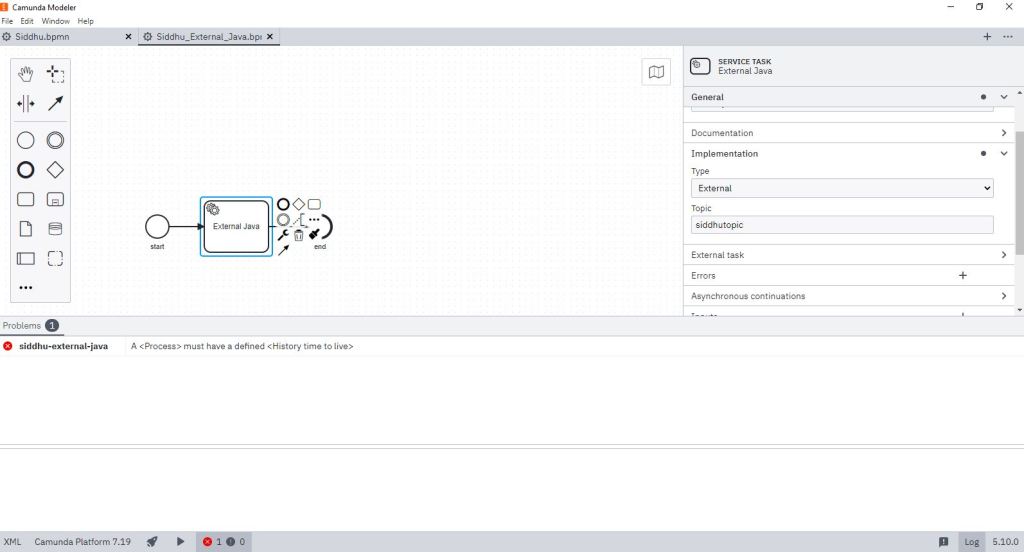
now start the process and check if it is displayed on the screen
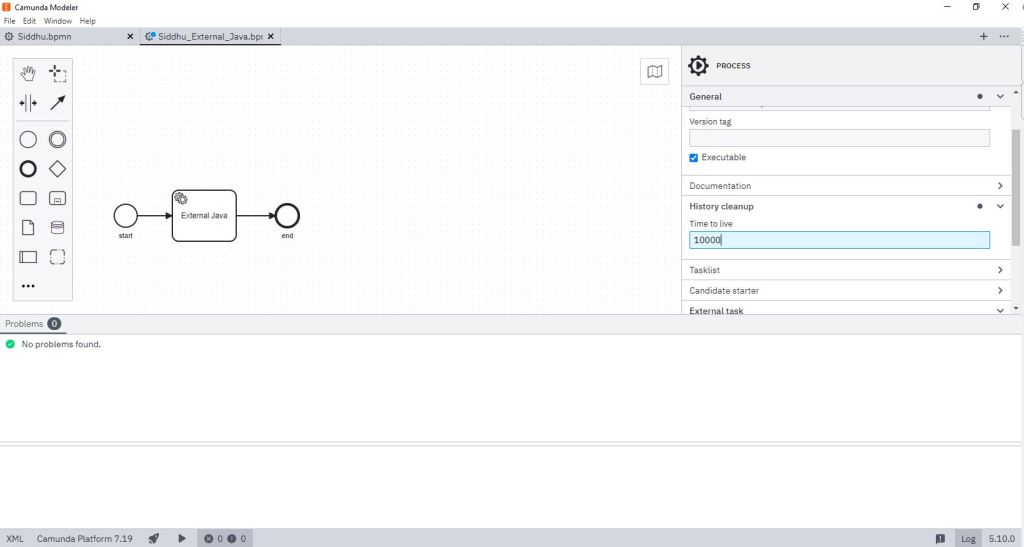




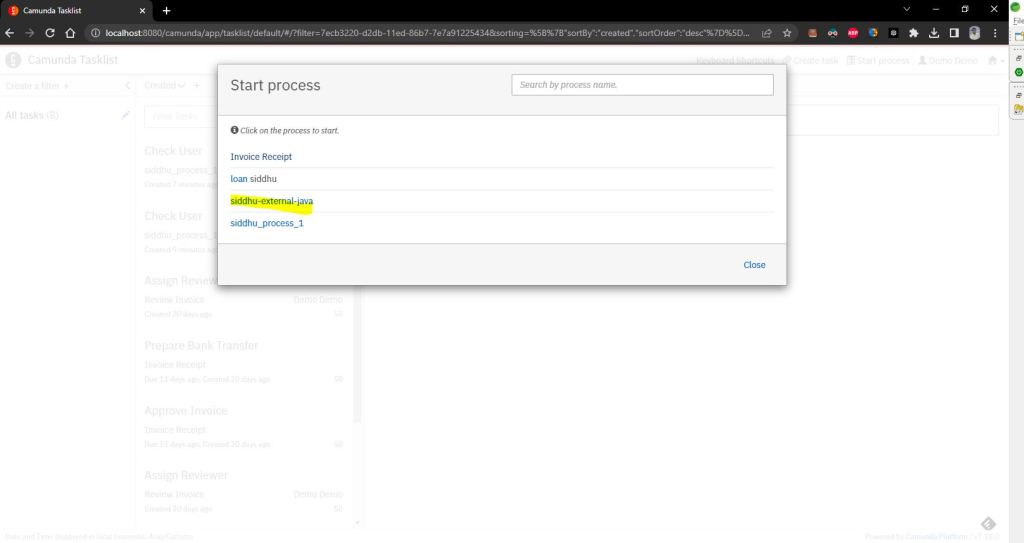


Now lets create a simple spring boot maven project as shown below which will contain our java class which will be called from the camunda service task. We will send few data as name and age from the ui that will be captured in our java class.

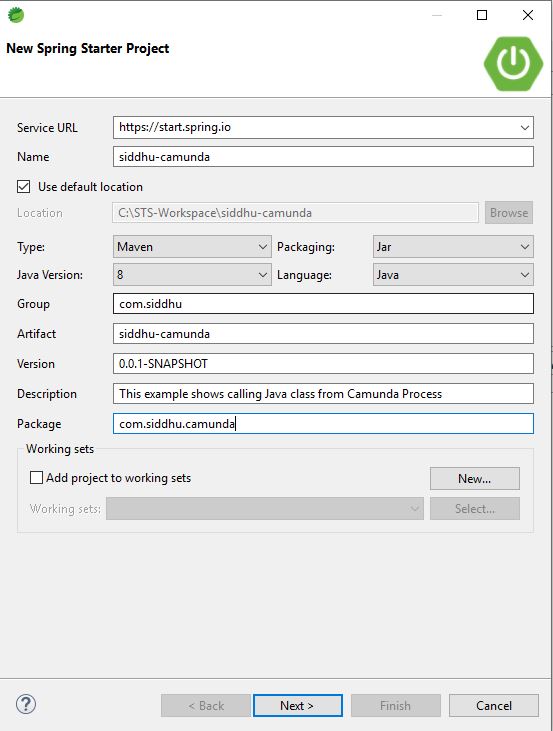

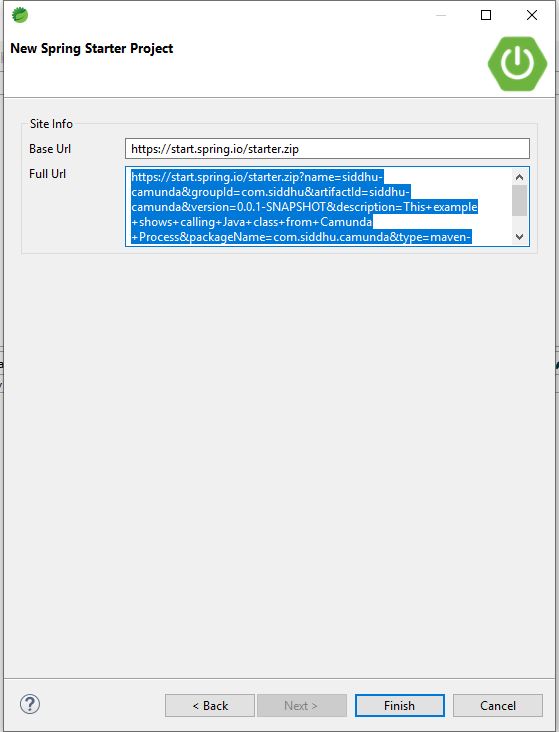
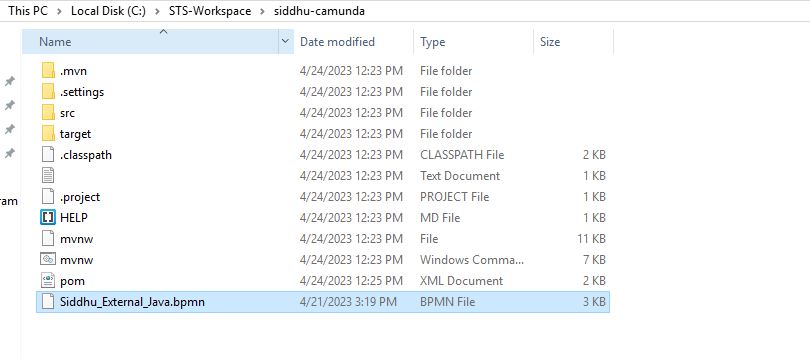
Now add following dependencies on the pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 | <dependency> <groupId>org.camunda.bpm.springboot</groupId> <artifactId>camunda-bpm-spring-boot-starter-external-task-client</artifactId> <version>${camunda.platform.runtime.version}</version> </dependency> <dependency> <groupId>com.sun.xml.bind</groupId> <artifactId>jaxb-impl</artifactId> <version>2.3.5</version> </dependency> |
our complete pom.xml is
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.7.3</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>com.siddhu</groupId> <artifactId>siddhu-camunda</artifactId> <version>0.0.1-SNAPSHOT</version> <name>siddhu-camunda</name> <description>This example shows calling Java class from Camunda Process</description> <properties> <java.version>1.8</java.version> <camunda.platform.runtime.version>7.18.0</camunda.platform.runtime.version> <spring.boot.version>2.7.3</spring.boot.version> <maven.compiler.plugin.version>3.8.1</maven.compiler.plugin.version> </properties> <dependencies> <dependency> <groupId>org.camunda.bpm.springboot</groupId> <artifactId>camunda-bpm-spring-boot-starter-external-task-client</artifactId> <version>${camunda.platform.runtime.version}</version> </dependency> <dependency> <groupId>com.sun.xml.bind</groupId> <artifactId>jaxb-impl</artifactId> <version>2.3.5</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>${spring.boot.version}</version> <executions> <execution> <id>repackage</id> <goals> <goal>repackage</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>${maven.compiler.plugin.version}</version> <configuration> <source>8</source> <target>8</target> </configuration> </plugin> </plugins> </build> </project> |
now lets add following yaml in our project application.yaml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | camunda.bpm.client: base-url: http://localhost:8080/engine-rest # the URL pointing to the Camunda Platform Runtime REST API lock-duration: 10000 # defines how many milliseconds the External Tasks are locked until they can be fetched again subscriptions: siddhutopic: variable-names: name,age # only fetch these variables process-definition-key: siddhu-external-java #this is the id that we give for process ID camunda.bpm: admin-user: id: demo password: demo firstName: Demo filter: create: All tasks |
Now lets keep our Siddhu_External_Java.bpmn in our springboot application.

Now lets write our java class that will be called from our camunda process
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | package com.siddhu.camunda; import org.camunda.bpm.client.spring.annotation.ExternalTaskSubscription; import org.camunda.bpm.client.task.ExternalTask; import org.camunda.bpm.client.task.ExternalTaskHandler; import org.camunda.bpm.client.task.ExternalTaskService; import org.camunda.bpm.engine.variable.VariableMap; import org.camunda.bpm.engine.variable.Variables; import org.springframework.stereotype.Component; @Component @ExternalTaskSubscription("siddhutopic") public class EligibilityHandler implements ExternalTaskHandler { @Override public void execute(ExternalTask extTask, ExternalTaskService extTaskService) { String name = extTask.getVariable("name"); long age = extTask.getVariable("age"); System.out.println("name"+name); System.out.println("age"+age); } } Now compile our maven build using clean install |

Now lets run the application and check if we are able to call the our java class from camunda process with defined values in process.

So the process is as given below
1- First create a bpmn file using moduler
2- Start the standalone camunda server
3- upload your created bpmn files from moduler to camiunda server. As we have choose “Generate Task form” this will ask the variable we has asked the user to enter.
4- finally check the task is created in camunda server
5- run the spring boot application and you will be able to call the java class from the process and also get the data in it.
you can download the code from here. Note bpmn file is place in resource folder of the project.
https://github.com/shdhumale/siddhu-camunda.git
No comments:
Post a Comment