For this example we will take our base project which has already integrated our PWA with notification created in blog
Source code :- https://github.com/shdhumale/complete-pwa-using-workbox-notification-fallout
Above example is running with concept such as
1- Precaching [Precaching Static Files Caching]:-
2- Offline page to show or 404 page not found:
3- Runtime caching
4- Strategies and also implementing different strategies.
5- Notification
In this example we are going to look into Sync behaviour for PWA i.e. it will address following concerns
1- If the use goes offline and fill the data and submit. This submitted data will be hold by IndexDB of the browser and when the user come online it will be inserted into the persistant system like DB.
2- If the data is upated at the DB end then it will be refelected once the user come online on the screen to the user.
Here we are going to use the Firebase DB. It has many advantage
1- We can easily integrate it with our application
2- Support Sync operation efficiently. We it handle indexdb of browser automatically i.e. we did need to write the code for the same.
3- It has the capacity to load our prepared appicatoin on the Firebase cloud server with https certification
4- We can easily test the application live behaviour from the mobile device as it is not live on the internet.
So first lets create our DB in Firstore. For that login to Firebase and follow below gien step.
Note:- Firestore is NOSQL DB i.e. it deals with collection, document etc.
1- Login to Firebase
2- Click on Go to Console
After this Firebae wil;l create the project for you.
Now go to project overview and click on this icon
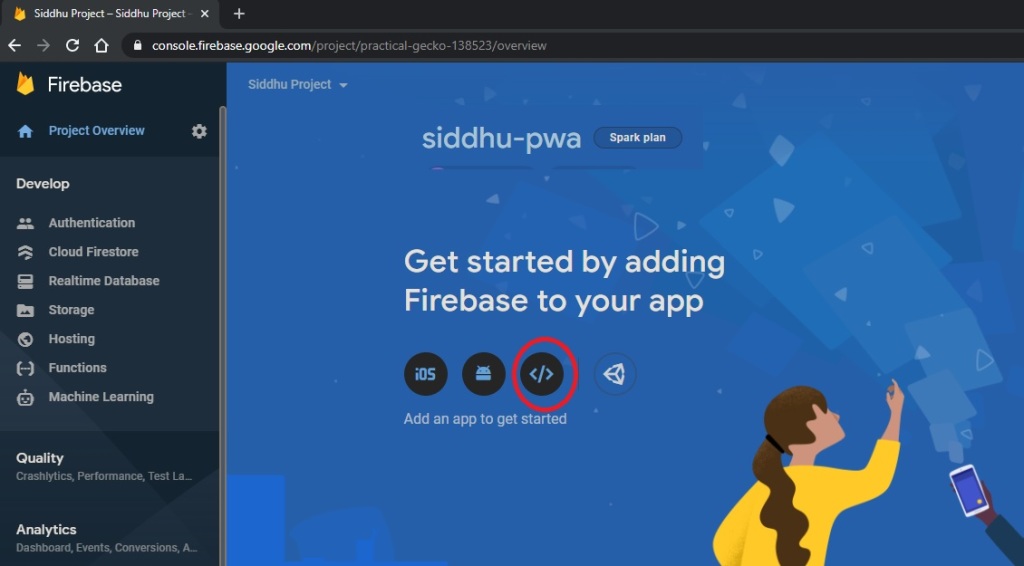
Copy the whole code this code will be used for connection to firestore.

Add this code inour index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | <!-- The core Firebase JS SDK is always required and must be listed first --> <script src="https://www.gstatic.com/firebasejs/7.24.0/firebase-app.js"></script> <script src="https://www.gstatic.com/firebasejs/7.24.0/firebase-firestore.js"></script> <!-- TODO: Add SDKs for Firebase products that you want to use <script src="https://www.gstatic.com/firebasejs/7.24.0/firebase-analytics.js"></script> <script> // Your web app's Firebase configuration // For Firebase JS SDK v7.20.0 and later, measurementId is optional var firebaseConfig = { apiKey: "AIzaSyAU_ScbpPpEkiHPZbzZj31_5Hi8NYHPkVs", authDomain: "siddhu-pwa.firebaseapp.com", databaseURL: "https://siddhu-pwa.firebaseio.com", projectId: "siddhu-pwa", storageBucket: "siddhu-pwa.appspot.com", messagingSenderId: "38363144416", appId: "1:38363144416:web:3a54a37ac815762dddf085", measurementId: "G-D6LRB9KK6Y" }; // Initialize Firebase firebase.initializeApp(firebaseConfig); firebase.analytics(); const db = firebase.firestore(); </script> |
Also create a db.js in js folder and add following line in it
// real-time listener
db.collection('users').onSnapshot(snapshot => {
console.log("-----------------------", snapshot.docChanges());
snapshot.docChanges().forEach(change => {
console.log(change);
console.log(change, change.doc.data(), change.doc.id);
if (change.type === 'added') {
userAdded(change.doc.data(), change.doc.id);
}
});
});
And in ui.js add this method// render User data
const userAdded = (data, id) => {
const html = ` ${data.userName}
${data.userAddress}
delete_outline
`;
users.innerHTML += html;
};
now perform this two command
npm run build
npm run start
we will be able to see the firebase data on the screen.

Now to show the offline DB data add these line in the uil.js
Now We can also see the data in offline as we are now storing the data in indexdb as shown below.

And this is done by this simple belwo line of code in our db.js
// enable offline data
db.enablePersistence()
.catch(function (err) {
if (err.code == 'failed-precondition') {
// probably multible tabs open at once
console.log('persistance failed');
} else if (err.code == 'unimplemented') {
// lack of browser support for the feature
console.log('persistance not available');
}
});
Now as soon as we go offline and add new record in the DB and hten again come online and do the refresh onthe screen we will be abel to see the new record on the screen.
Now lets perform add operation from the screen to the firebase.
Add following line in your db.js
// add new user
const form = document.querySelector('form');
form.addEventListener('submit', evt => {
evt.preventDefault();
1 2 3 4 5 6 7 8 9 10 | const user = { userName: form.title.value, userAddress: form.address.value }; db.collection('users').add(user) .catch(err => console.log(err)); form.title.value = ''; form.address.value = ''; |
});
Now again execute below command
npm run build
npm run start
Now lets create the data offline and we can check no data is created in DB but it is is created in IndexDB
Now lets go online and we will see the newly created data is also created in Firebasd DB.

Now lets perform the last delete operation
Add following line in db.js
// real-time listener
db.collection('users').onSnapshot(snapshot => {
console.log("-----------------------", snapshot.docChanges());
snapshot.docChanges().forEach(change => {
console.log(change);
console.log(change, change.doc.data(), change.doc.id);
if (change.type === 'added') {
userAdded(change.doc.data(), change.doc.id);
}
if (change.type === 'removed') {
// remove the document data from the web page
userRemoved(change.doc.id);
}
});
});
// remove a user
const recipeContainer = document.querySelector('.users');
recipeContainer.addEventListener('click', evt => {
if(evt.target.tagName === 'I'){
const id = evt.target.getAttribute('data-id');
//console.log(id);
db.collection('users').doc(id).delete();
}
})
And add this line in ui.js ..Even though if you do not add below line in ui.js it will remove the item from the list once you refreshed the data.
// remove user
const userRemoved
= (id) => {
const user = document.querySelector(.user[data-id=${id}]);
user.remove();
};
Now again execute below command
npm run build
npm run start
Download source:-
https://github.com/shdhumale/pwawithlivedatafirebase.git
No comments:
Post a Comment