Hibernate is ORM = Object Relation Mapping tool. This means in Hibernate we map our RDBMS Database table in Object i.e. JAVA object Class.
JAVA Object --> ORM/Hibernate --> RDBMS.
Supporting DB:- Oracle, MySQL, DB2, Sybase SQL Server, HSQL Database Engine etc
It is the provider for JPA= JAVA Persistance API.
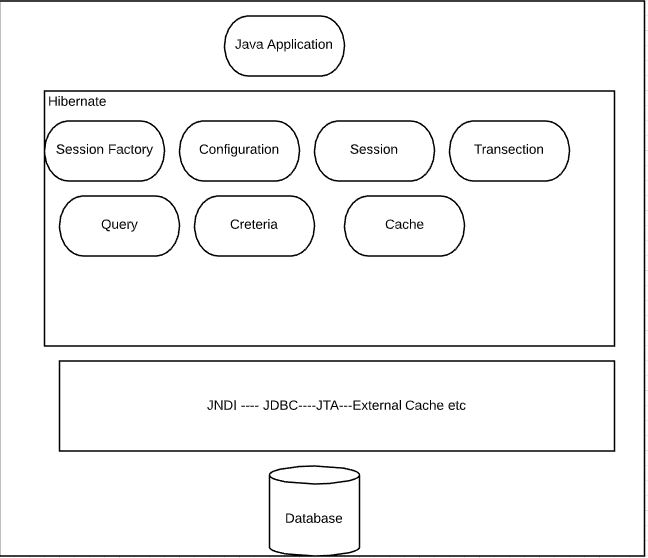
Hibernate can be used easily with JAVA application as a JPA Provider. We just need required jar to be added in the class path of the application. We can download the jar from the below given site
http://hibernate.org/orm/releases/
Once you download and unzip the latest version you will be able to see the below screen we generally required jar from required folder inside lib folder of the download
hibernate-release-5.4.10.Final\lib\required
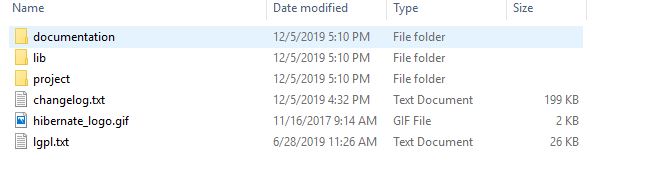
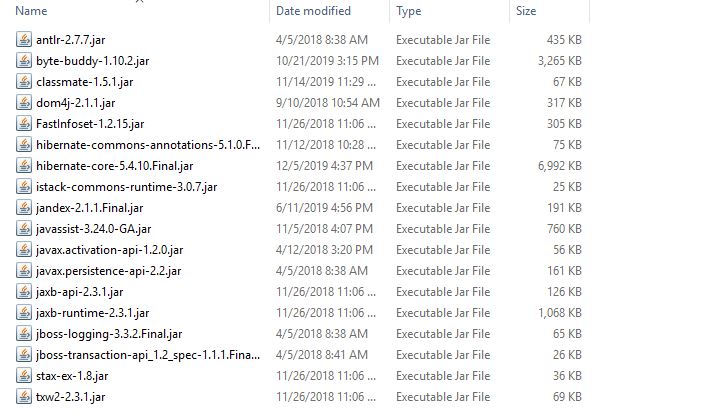
Now lets see the important aspect of Hibernate i.e. configuration, Seesion Factory , Session, Query language, Criteria Language etc.
1- Configuration:-
Hibernate can be configure in two ways 1- Using xml file 2- using property files. I prefer to use XML files. for configufation of the hibernate we gernerally use hibernate.cfg.xml. Few of the parameter that we need to keep in mind while configurations are
- hibernate.dialect :- use to generate appropriate SQL for chosend DB.
- hibernate.connection.driver_class- this is driver class used to connect DB.
- hibernate.connection.url - this is JDBC url to connect
- hibernate.connection.username and hibernate.connection.password - this is connection username and password
2- Session factory :- This is very important class for hibernate. It is created only once when the application is loaded and its function is to provide the access to session class instance used in the hibernate extensively. Depending on the number of *.cfg.xml files we create single respective session factory instance and destroy once the server is down of application is removed. This is thread safe object as it is created once.
3- Session :- This is another important object which is used to create a physical DB connection. It works under the hood of Session factory class. Session is the base and main class of hibernate.It perform operation like getting connection , begining the transection executing the query and finally closing it. It is MANDATE to close the session object of kill it once the work is done. Session object is created and killed as per the which of the developer and should be always killed as this is not thread safe object. It has some inbuild function such as beginTransaction(), cancelQuery(), createQuery(String queryString), createSQLQuery(String queryString), delete() etc
4- Persistance class :- This is the java class that represent the Database table. We can say in can be in one of the state in applications. Genereally in persistance class in java we keep the name of the class as similar to DB Table name and its field as same as Table field name. It is just pojo with getter and setter method.
transient - A new instance of a persistent class not associated with a Session and hence has nothing to do with DB.
persistent - After assiociating with Session this object become persistent i.e. they now represent database
detached - after closing the Hibernate Session, the persistent instance will become a detached instance.
5- Mapping files :- This are terms as *.hbm.xml. We keep the name * as name of the DB table name. Lets see few of details of this files
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name = "SiddhuClass" table = "SIDDHUCLASS">
<meta attribute = "class-description">
Optional and this give information of what this class contains.
</meta>
<id name = "idClass" type = "int" column = "IDCOLUMN">
<generator class="native"/>
</id>
<property name = "firstNameClass" column = "FIRST_NAME_COLUMN" type = "string"/>
<property name = "LastNameClass" column = "LAST_NAME_COLUMN" type = "string"/>
</class>
</hibernate-mapping>
All Hibernate Mapping files start with hibernate-mapping tag. Class tag gives the mapping between the java object and DB table name here SIDDHUCLASS is DB table and SiddhuClass is java class. meta tag is optional and can be used to give to give information of what this class contains.id tag is used for primary key idClass is java idClass field and IDCOLUMN is DB tabel PK column name. type define the Hibernate data type. This is different from JAVA and DB table type.generator tag is used to tell that PK is auto generated using native methods. property tag gives the information of the other field.
Before going lets see few of the type i.e. Hibernate type that is used to map between JAVA dn DB data type.integer, integer, long, shot, float, character, sting etc.
Now lets see the working example
1- First create a table with name SIDDHUCLASS
create table SIDDHUCLASS (
IDCOLUMN INT NOT NULL,
FIRST_NAME_COLUMN VARCHAR(20) default NULL,
LAST_NAME_COLUMN VARCHAR(20) default NULL,
PRIMARY KEY (IDCOLUMN)
);
2- configuration files
we are usign oracle and hence using oracledriver, with dialect Oracle10gDialect. show_sql will show your query in log this is important to do debug.
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-configuration SYSTEM
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">oracle.jdbc.driver.OracleDriver</property>
<property name="hibernate.connection.url">jdbc:oracle:thin:@localhost:1521:xe</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">root</property>
<property name="hibernate.dialect">org.hibernate.dialect.Oracle10gDialect</property>
<property name="show_sql">true</property>
<mapping resource="SiddhuClass.hbm.xml"></mapping>
</session-factory>
</hibernate-configuration>
3- hbm file
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name = "com.siddhu.SiddhuClass" table = "SIDDHUCLASS">
<meta attribute = "class-description">
Optional and this give information of what this class contains.
</meta>
<id name = "idClass" type = "int" column = "IDCOLUMN">
</id>
<property name = "firstNameClass" column = "first_name_Column" type = "string"/>
<property name = "LastNameClass" column = "last_name_column" type = "string"/>
</class>
</hibernate-mapping>
4- Lets create pojo persistance class as shown below
package com.siddhu;
public class SiddhuClass {
private int idClass;
private String firstNameClass;
private String LastNameClass;
public SiddhuClass() {}
public SiddhuClass(int id,String fname, String lname) {
this.idClass = id;
this.firstNameClass = fname;
this.LastNameClass = lname;
}
public int getIdClass() {
return idClass;
}
public void setIdClass(int idClass) {
this.idClass = idClass;
}
public String getFirstNameClass() {
return firstNameClass;
}
public void setFirstNameClass(String firstNameClass) {
this.firstNameClass = firstNameClass;
}
public String getLastNameClass() {
return LastNameClass;
}
public void setLastNameClass(String lastNameClass) {
LastNameClass = lastNameClass;
}
}
5- Main OperateSiddhuClass class to show all the CRUD operation
package com.siddhu;
import java.util.List;
import java.util.Date;
import java.util.Iterator;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class OperateSiddhuClass {
private static SessionFactory factory;
public static void main(String[] args) {
try {
factory = new Configuration().configure().buildSessionFactory();
} catch (Exception ex) {
throw new ExceptionInInitializerError(ex);
}
OperateSiddhuClass objOperateSiddhuClass = new OperateSiddhuClass();
// Add records in database
Integer siddhuID1 = objOperateSiddhuClass.createSiddhu(1,"Siddhu1", "Dhumale1");
Integer siddhuID2 = objOperateSiddhuClass.createSiddhu(2,"Siddhu2", "Dhumale2");
// List records
objOperateSiddhuClass.showSiddhuValues();
//Update records
objOperateSiddhuClass.updateSiddhu(siddhuID1, "sername change");
//Delete operation
objOperateSiddhuClass.deleteSiddhu(siddhuID2);
}
//To create Data in Table
public Integer createSiddhu(int id, String firstName, String lastName){
Session session = factory.openSession();
Transaction tx = null;
Integer siddhuID = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = new SiddhuClass(id, firstName, lastName);
siddhuID = (Integer) session.save(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
return siddhuID;
}
//To read data from table
public void showSiddhuValues( ){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
List siddhuValues = session.createQuery("FROM SiddhuClass").list();
for (Iterator iterator = siddhuValues.iterator(); iterator.hasNext();){
SiddhuClass siddhu = (SiddhuClass) iterator.next();
System.out.print("First Name: " + siddhu.getFirstNameClass() + " Last Name: " + siddhu.getLastNameClass());
}
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
//To update
public void updateSiddhu(Integer siddhuId, String newLastName){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = (SiddhuClass)session.get(SiddhuClass.class, siddhuId);
siddhu.setLastNameClass(newLastName);
session.update(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
//To Delete
public void deleteSiddhu(Integer siddhuId){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = (SiddhuClass)session.get(SiddhuClass.class, siddhuId);
session.delete(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
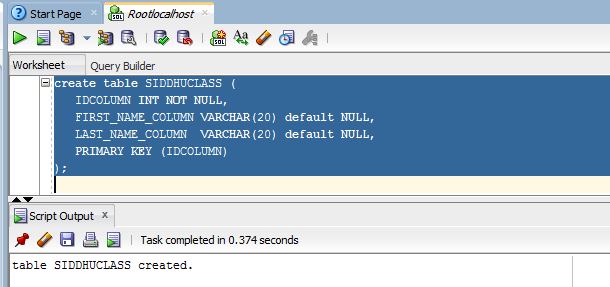
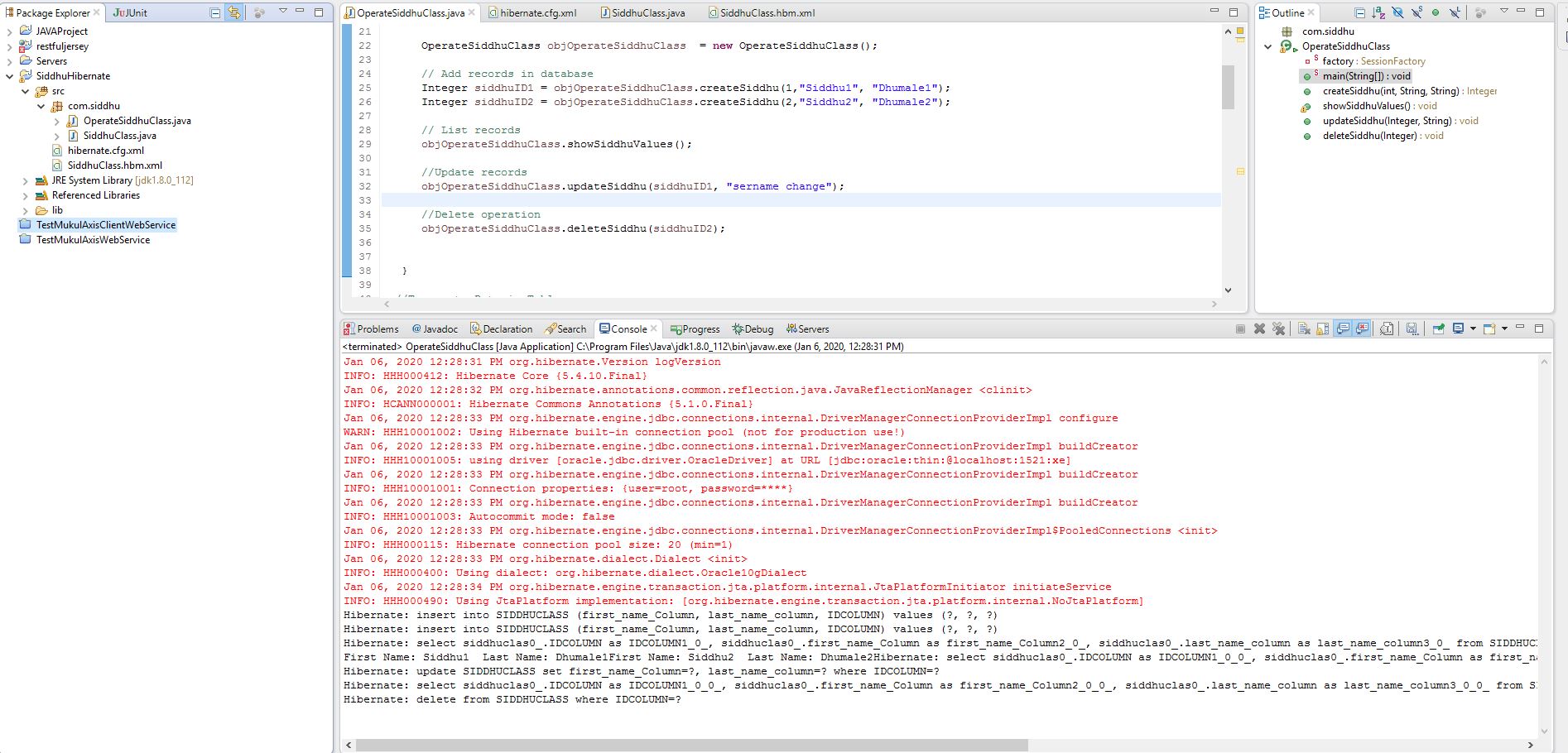
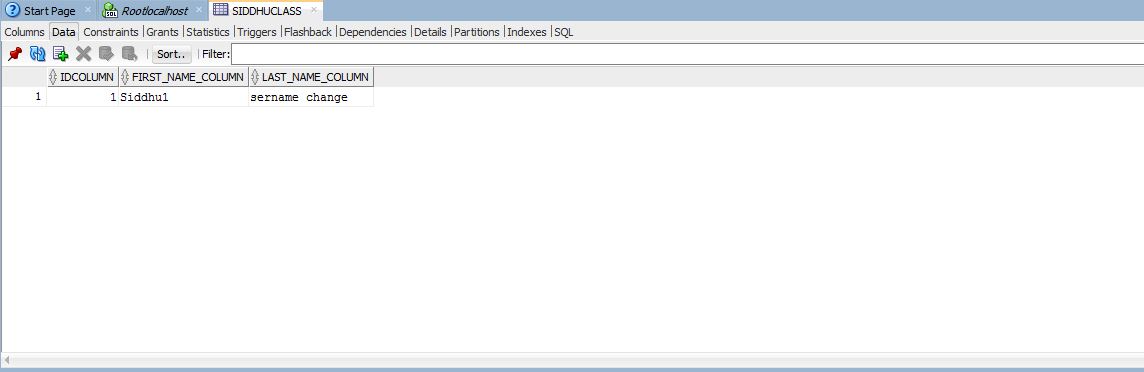
Same above example we can also do using Annotation instead of hbm file. but personaly i prefer to use hbm files for more clarity.
Additional also refer to the HQL- Hibernate query languge aspect of the hibernate. In this we use to query java object rather than DB table and hence irrespective of any DB changes this will always work because we are acting on JAVA object and has nothing to do with DB table. In HQL we had select, where, order , group by , named parameter, delete etc.
Also have some eye on Criteria query where in we use class such as Restrictions and Projections class to operate on the JAVA class object . Restrictions give us api like greater than gt, smaller then st, like, isnull, isemplty etc ...Projections give us inbuild function like max, count, min , sum, rowcount etc
Hibernate also allowed to use the native query i.e. direct db query to be executed from the java class using public SQLQuery createSQLQuery(String sqlString) throws HibernateException.
Caching :- this is important part of the hibernate. There are three type of cache
1- First level:- This is by default cache provided by the Hibernate. Developer has nothing to do with this cache and it is used by hibernate for performance of the application.
2- Second level:- This is cache which developer can play. Many third party cache are available such as EHCache, JBoss cache etc. Refer to teh online example for the same. PLEASE USE THIS CACHE IF IT EXTREME USEFUL ELSE IT WILL DEGRADE PREFORMACE.
3- Query cache:- this is used when we are sure our DB table is not changed frequently. in this cache we store the data of the query and time stamp of the DB table modificaiton in HArd disk. When ever request come for the same query we give the date to application from Cache if the Time stamp is not modified else we make a new call.
Hibernate also support batch processing as it is requird if we are working with huge record. If we dont take the batch concept in practise performing CRUD with more than 1 lac of record will make your application and DB down very soon.
JAVA Object --> ORM/Hibernate --> RDBMS.
Supporting DB:- Oracle, MySQL, DB2, Sybase SQL Server, HSQL Database Engine etc
It is the provider for JPA= JAVA Persistance API.
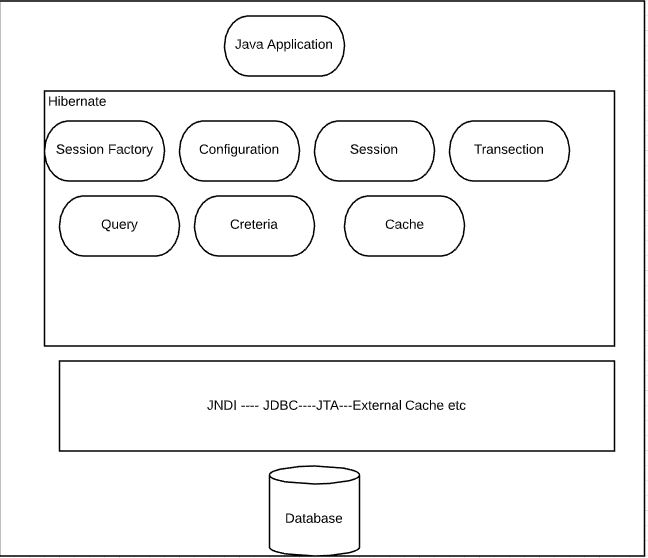
Hibernate can be used easily with JAVA application as a JPA Provider. We just need required jar to be added in the class path of the application. We can download the jar from the below given site
http://hibernate.org/orm/releases/
Once you download and unzip the latest version you will be able to see the below screen we generally required jar from required folder inside lib folder of the download
hibernate-release-5.4.10.Final\lib\required
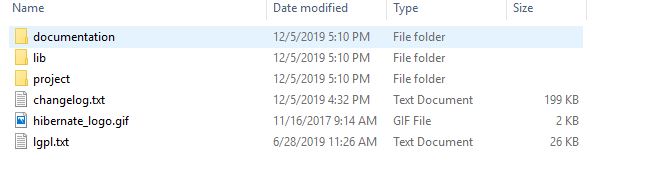
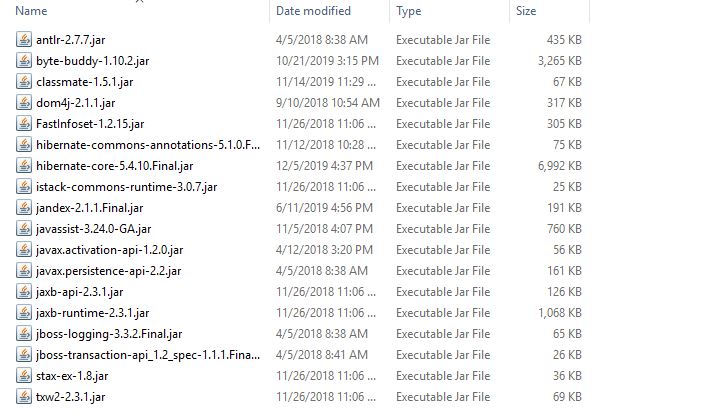
Now lets see the important aspect of Hibernate i.e. configuration, Seesion Factory , Session, Query language, Criteria Language etc.
1- Configuration:-
Hibernate can be configure in two ways 1- Using xml file 2- using property files. I prefer to use XML files. for configufation of the hibernate we gernerally use hibernate.cfg.xml. Few of the parameter that we need to keep in mind while configurations are
- hibernate.dialect :- use to generate appropriate SQL for chosend DB.
- hibernate.connection.driver_class- this is driver class used to connect DB.
- hibernate.connection.url - this is JDBC url to connect
- hibernate.connection.username and hibernate.connection.password - this is connection username and password
2- Session factory :- This is very important class for hibernate. It is created only once when the application is loaded and its function is to provide the access to session class instance used in the hibernate extensively. Depending on the number of *.cfg.xml files we create single respective session factory instance and destroy once the server is down of application is removed. This is thread safe object as it is created once.
3- Session :- This is another important object which is used to create a physical DB connection. It works under the hood of Session factory class. Session is the base and main class of hibernate.It perform operation like getting connection , begining the transection executing the query and finally closing it. It is MANDATE to close the session object of kill it once the work is done. Session object is created and killed as per the which of the developer and should be always killed as this is not thread safe object. It has some inbuild function such as beginTransaction(), cancelQuery(), createQuery(String queryString), createSQLQuery(String queryString), delete() etc
4- Persistance class :- This is the java class that represent the Database table. We can say in can be in one of the state in applications. Genereally in persistance class in java we keep the name of the class as similar to DB Table name and its field as same as Table field name. It is just pojo with getter and setter method.
transient - A new instance of a persistent class not associated with a Session and hence has nothing to do with DB.
persistent - After assiociating with Session this object become persistent i.e. they now represent database
detached - after closing the Hibernate Session, the persistent instance will become a detached instance.
5- Mapping files :- This are terms as *.hbm.xml. We keep the name * as name of the DB table name. Lets see few of details of this files
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name = "SiddhuClass" table = "SIDDHUCLASS">
<meta attribute = "class-description">
Optional and this give information of what this class contains.
</meta>
<id name = "idClass" type = "int" column = "IDCOLUMN">
<generator class="native"/>
</id>
<property name = "firstNameClass" column = "FIRST_NAME_COLUMN" type = "string"/>
<property name = "LastNameClass" column = "LAST_NAME_COLUMN" type = "string"/>
</class>
</hibernate-mapping>
All Hibernate Mapping files start with hibernate-mapping tag. Class tag gives the mapping between the java object and DB table name here SIDDHUCLASS is DB table and SiddhuClass is java class. meta tag is optional and can be used to give to give information of what this class contains.id tag is used for primary key idClass is java idClass field and IDCOLUMN is DB tabel PK column name. type define the Hibernate data type. This is different from JAVA and DB table type.generator tag is used to tell that PK is auto generated using native methods. property tag gives the information of the other field.
Before going lets see few of the type i.e. Hibernate type that is used to map between JAVA dn DB data type.integer, integer, long, shot, float, character, sting etc.
Now lets see the working example
1- First create a table with name SIDDHUCLASS
create table SIDDHUCLASS (
IDCOLUMN INT NOT NULL,
FIRST_NAME_COLUMN VARCHAR(20) default NULL,
LAST_NAME_COLUMN VARCHAR(20) default NULL,
PRIMARY KEY (IDCOLUMN)
);
2- configuration files
we are usign oracle and hence using oracledriver, with dialect Oracle10gDialect. show_sql will show your query in log this is important to do debug.
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-configuration SYSTEM
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">oracle.jdbc.driver.OracleDriver</property>
<property name="hibernate.connection.url">jdbc:oracle:thin:@localhost:1521:xe</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">root</property>
<property name="hibernate.dialect">org.hibernate.dialect.Oracle10gDialect</property>
<property name="show_sql">true</property>
<mapping resource="SiddhuClass.hbm.xml"></mapping>
</session-factory>
</hibernate-configuration>
3- hbm file
<?xml version = "1.0" encoding = "utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name = "com.siddhu.SiddhuClass" table = "SIDDHUCLASS">
<meta attribute = "class-description">
Optional and this give information of what this class contains.
</meta>
<id name = "idClass" type = "int" column = "IDCOLUMN">
</id>
<property name = "firstNameClass" column = "first_name_Column" type = "string"/>
<property name = "LastNameClass" column = "last_name_column" type = "string"/>
</class>
</hibernate-mapping>
4- Lets create pojo persistance class as shown below
package com.siddhu;
public class SiddhuClass {
private int idClass;
private String firstNameClass;
private String LastNameClass;
public SiddhuClass() {}
public SiddhuClass(int id,String fname, String lname) {
this.idClass = id;
this.firstNameClass = fname;
this.LastNameClass = lname;
}
public int getIdClass() {
return idClass;
}
public void setIdClass(int idClass) {
this.idClass = idClass;
}
public String getFirstNameClass() {
return firstNameClass;
}
public void setFirstNameClass(String firstNameClass) {
this.firstNameClass = firstNameClass;
}
public String getLastNameClass() {
return LastNameClass;
}
public void setLastNameClass(String lastNameClass) {
LastNameClass = lastNameClass;
}
}
5- Main OperateSiddhuClass class to show all the CRUD operation
package com.siddhu;
import java.util.List;
import java.util.Date;
import java.util.Iterator;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class OperateSiddhuClass {
private static SessionFactory factory;
public static void main(String[] args) {
try {
factory = new Configuration().configure().buildSessionFactory();
} catch (Exception ex) {
throw new ExceptionInInitializerError(ex);
}
OperateSiddhuClass objOperateSiddhuClass = new OperateSiddhuClass();
// Add records in database
Integer siddhuID1 = objOperateSiddhuClass.createSiddhu(1,"Siddhu1", "Dhumale1");
Integer siddhuID2 = objOperateSiddhuClass.createSiddhu(2,"Siddhu2", "Dhumale2");
// List records
objOperateSiddhuClass.showSiddhuValues();
//Update records
objOperateSiddhuClass.updateSiddhu(siddhuID1, "sername change");
//Delete operation
objOperateSiddhuClass.deleteSiddhu(siddhuID2);
}
//To create Data in Table
public Integer createSiddhu(int id, String firstName, String lastName){
Session session = factory.openSession();
Transaction tx = null;
Integer siddhuID = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = new SiddhuClass(id, firstName, lastName);
siddhuID = (Integer) session.save(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
return siddhuID;
}
//To read data from table
public void showSiddhuValues( ){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
List siddhuValues = session.createQuery("FROM SiddhuClass").list();
for (Iterator iterator = siddhuValues.iterator(); iterator.hasNext();){
SiddhuClass siddhu = (SiddhuClass) iterator.next();
System.out.print("First Name: " + siddhu.getFirstNameClass() + " Last Name: " + siddhu.getLastNameClass());
}
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
//To update
public void updateSiddhu(Integer siddhuId, String newLastName){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = (SiddhuClass)session.get(SiddhuClass.class, siddhuId);
siddhu.setLastNameClass(newLastName);
session.update(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
//To Delete
public void deleteSiddhu(Integer siddhuId){
Session session = factory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
SiddhuClass siddhu = (SiddhuClass)session.get(SiddhuClass.class, siddhuId);
session.delete(siddhu);
tx.commit();
} catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
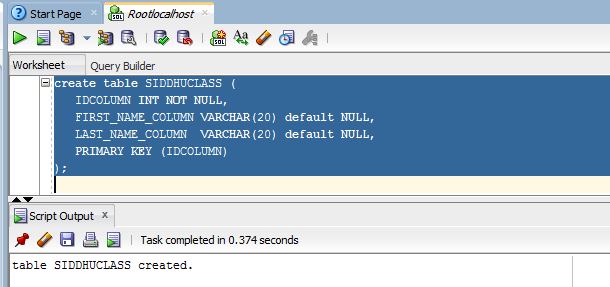
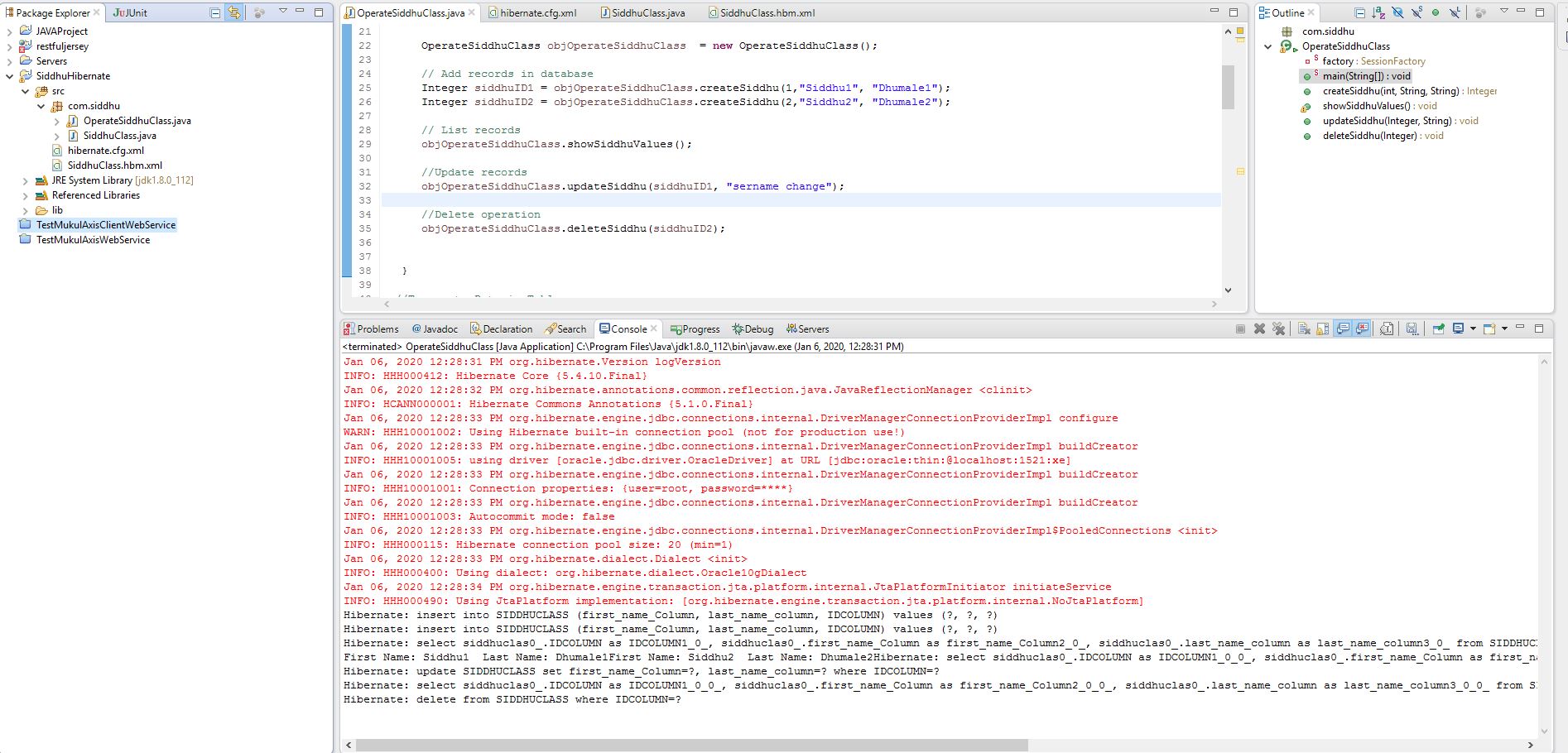
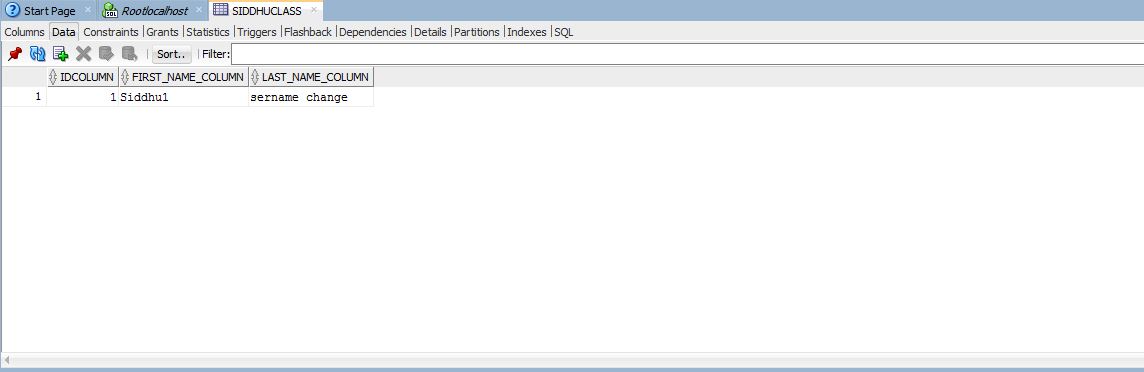
Same above example we can also do using Annotation instead of hbm file. but personaly i prefer to use hbm files for more clarity.
Additional also refer to the HQL- Hibernate query languge aspect of the hibernate. In this we use to query java object rather than DB table and hence irrespective of any DB changes this will always work because we are acting on JAVA object and has nothing to do with DB table. In HQL we had select, where, order , group by , named parameter, delete etc.
Also have some eye on Criteria query where in we use class such as Restrictions and Projections class to operate on the JAVA class object . Restrictions give us api like greater than gt, smaller then st, like, isnull, isemplty etc ...Projections give us inbuild function like max, count, min , sum, rowcount etc
Hibernate also allowed to use the native query i.e. direct db query to be executed from the java class using public SQLQuery createSQLQuery(String sqlString) throws HibernateException.
Caching :- this is important part of the hibernate. There are three type of cache
1- First level:- This is by default cache provided by the Hibernate. Developer has nothing to do with this cache and it is used by hibernate for performance of the application.
2- Second level:- This is cache which developer can play. Many third party cache are available such as EHCache, JBoss cache etc. Refer to teh online example for the same. PLEASE USE THIS CACHE IF IT EXTREME USEFUL ELSE IT WILL DEGRADE PREFORMACE.
3- Query cache:- this is used when we are sure our DB table is not changed frequently. in this cache we store the data of the query and time stamp of the DB table modificaiton in HArd disk. When ever request come for the same query we give the date to application from Cache if the Time stamp is not modified else we make a new call.
Hibernate also support batch processing as it is requird if we are working with huge record. If we dont take the batch concept in practise performing CRUD with more than 1 lac of record will make your application and DB down very soon.
No comments:
Post a Comment