This blog will explain to you how use the google recaptcha in angular project
First we need to create a site key and secrete key for that visit to below site
https://www.google.com/recaptcha/admin/create
Fill the data as per site url. As I am using local host i used 127.0.0.1 as localhost is not supported as name in the url by google for captcha.
Once the site key and secret key is created, store them properly as we are going to use that in our angular project.
Let's now create a simple Angular project using below command
ng new angular-google-captacha
Lets install ng-recaptcha that we can use in our created project
C:\vscode-angular-workspace\angular-google-captcha>npm i ng-recaptcha –save
and now change below files as follows:-
1- app.module.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { RecaptchaModule } from 'ng-recaptcha'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, RecaptchaModule, ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
2- app.component.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | import { Component } from '@angular/core'; @Component({ selector: 'app-root', //templateUrl: './app.component.html', template: `<re-captcha (resolved)="resolved($event)" siteKey="YOUR_SITE_KEY"></re-captcha>`, styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'angular-google-captcha'; resolved(captchaResponse: string) { console.log(`Resolved captcha with response: ${captchaResponse}`); } } |
Note in above you have mention “YOUR_SITE_KEY” this is the site key that is created above by you.
Now let's hit the url
http://127.0.0.1:4200/
You will be able to see the below screen
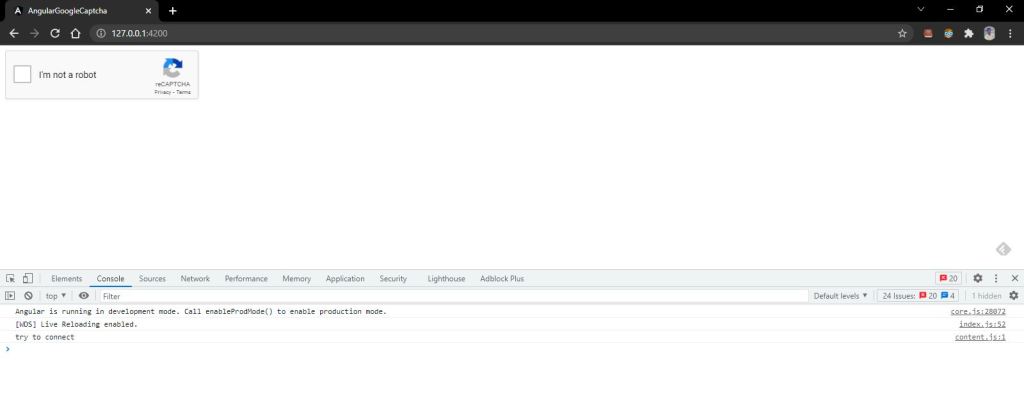
Click on “I am not robot” and you will see we get success response. It you end the wrong site key the you will get null as response.
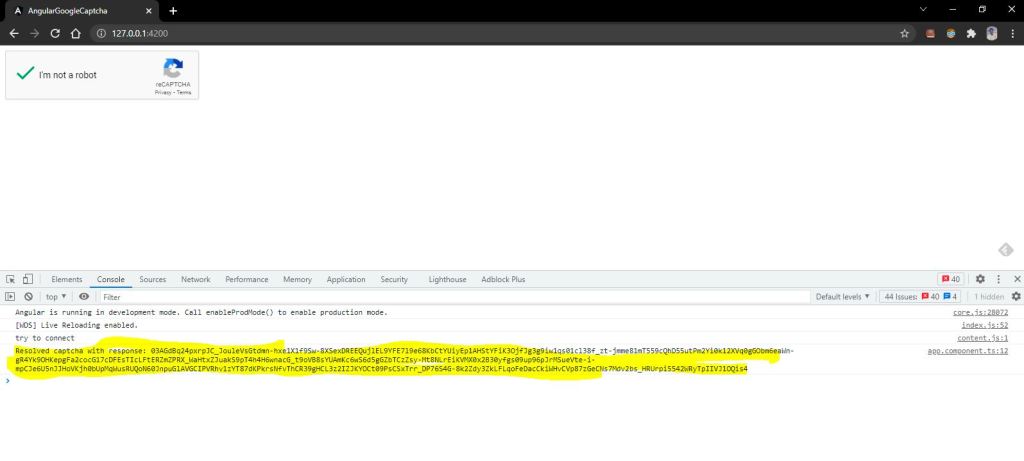
Download link :-
https://github.com/shdhumale/angular-google-captcha.git
Note:-
For more details, visit to below site
No comments:
Post a Comment