Some of the follower has asked to explain Redux concept in more simple way as they found below explainination little bit hard to understand.
http://siddharathadhumale.blogspot.com/2020/04/redux-library-and-its-uses-in-java.html
Considering this i am trying to explain Redux with more simple form. I assume if you are not aware of the Redux concept please refer above url.
Redux is the third party extension that can be used as a predictable state container .
Following are the important aspect of the Redux
-> SAR – MA :-
‘- Store :- store the state of the Application as a central repository shared with all the applications.Create store , Getstate – to get the state of the application, susbcribe(listener) for subscrbing and unsubscribing the listener as the state change and dispatch(action) is used to make change in state in the store.
‘- Action :- indicate what need to be done with the state which is readonly. No Direct update is avaialble for the application to change the state in store.
‘- Reducer:- Actully carrier out the change in the state.
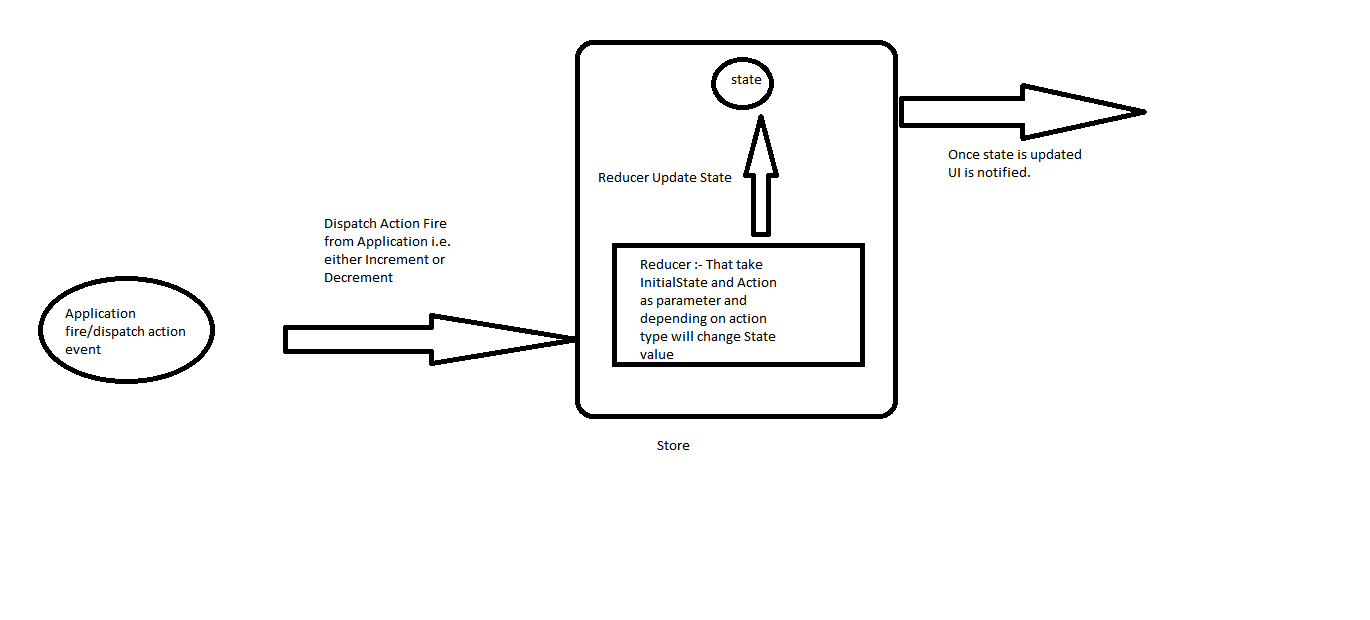
Lets take a simple example where in we have a varible named as counter whose value is store in the state. Through action we either increment or decrement the counter which in tern perform the increment or decrement of its state value in store. and fially we can get the updated inc/dec value of the counter stored as state in store.
Step :1 - Install redux from npm so that we can create store to accomodate state values. Use below command to install redux.
npm install redux --save
check in package.json file if the redux is added into dependencies. If you do not have package.json create it using command
npm init
Step 2:- First we need to create a store using below command
const { createStore } = require('redux')
const store = createStore()
Step 3:- Now store take Reducer as an argument so lets create a reducer
const myReducer = (state, action) => {
}
We can also define the initial state as shown below
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
}
Step 4:- Now lets assign this myReducer to our create store
const store = createStore(myReducer)
step 5:- As we did not have any UI applicaton that can fire the event to change the value of the counter inside store we can do it manually by dispatch methond as shown below in which we tell reducer that action is dispatched with its type as increment
store.dispatch({ type: 'increment' })
Step 5:- Now inside reducer we can check this action type and confirm what action need to be done with counter variable that is stored in global or store state
for increment we want to increase the value of counter with 1 and need to update that value in global state in store.
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
return newState
}
Step 5:- Now finally execute the below programe and you will see how we can do the increment and decrement of the counter value which is store as global state in store of redux.
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - 1
}
return newState
}
const store = createStore(myReducer)
console.log(store.getState())
store.dispatch({ type: 'increment' })
console.log(store.getState())
store.dispatch({ type: 'decrement' })
console.log(store.getState())
Out put
PS C:\Visual_Source_Code_WorkSpace_ReactJSNew\simplereduxexample> node .\simpleredux.js
{ counter: 1 }
{ counter: 2 }
{ counter: 1 }
now the question arise how i would be know automatically that value of global state i.e. counter inside store is changed. We dont want to manually track everytime checking global state change. For this we have beautiful concept of subscriber
store.subscribe(() => {
console.log("Nofied automatically that state is changed:- " + JSON.stringify(store.getState()))
})
and then we can remove all the console log.
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - 1
}
return newState
}
const store = createStore(myReducer)
store.subscribe(() => {
console.log("Nofied automatically that state is changed:- " + JSON.stringify(store.getState()))
})
//console.log(store.getState())
store.dispatch({ type: 'increment' })
//console.log(store.getState())
store.dispatch({ type: 'decrement' })
//console.log(store.getState())
now lets say we also want to pass a value that will indicate how much we want to do increment and decreament and this value is called payload
i.e.
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + action.val
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - action.val
}
return newState
}
store.dispatch({ type: 'increment', val:10})
Final code will be
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + action.val
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - action.val
}
return newState
}
const store = createStore(myReducer)
store.subscribe(() => {
console.log("Notified automatically that state is changed:- " + JSON.stringify(store.getState()))
})
//console.log(store.getState())
store.dispatch({ type: 'increment', val: 10 })
//console.log(store.getState())
store.dispatch({ type: 'decrement', val: 10 })
//console.log(store.getState())
OutPut :-
PS C:\Visual_Source_Code_WorkSpace_ReactJSNew\simplereduxexample> node .\simpleredux.js
Notified automatically that state is changed:- {"counter":10}
Notified automatically that state is changed:- {"counter":1}
http://siddharathadhumale.blogspot.com/2020/04/redux-library-and-its-uses-in-java.html
Considering this i am trying to explain Redux with more simple form. I assume if you are not aware of the Redux concept please refer above url.
Redux is the third party extension that can be used as a predictable state container .
Following are the important aspect of the Redux
-> SAR – MA :-
‘- Store :- store the state of the Application as a central repository shared with all the applications.Create store , Getstate – to get the state of the application, susbcribe(listener) for subscrbing and unsubscribing the listener as the state change and dispatch(action) is used to make change in state in the store.
‘- Action :- indicate what need to be done with the state which is readonly. No Direct update is avaialble for the application to change the state in store.
‘- Reducer:- Actully carrier out the change in the state.
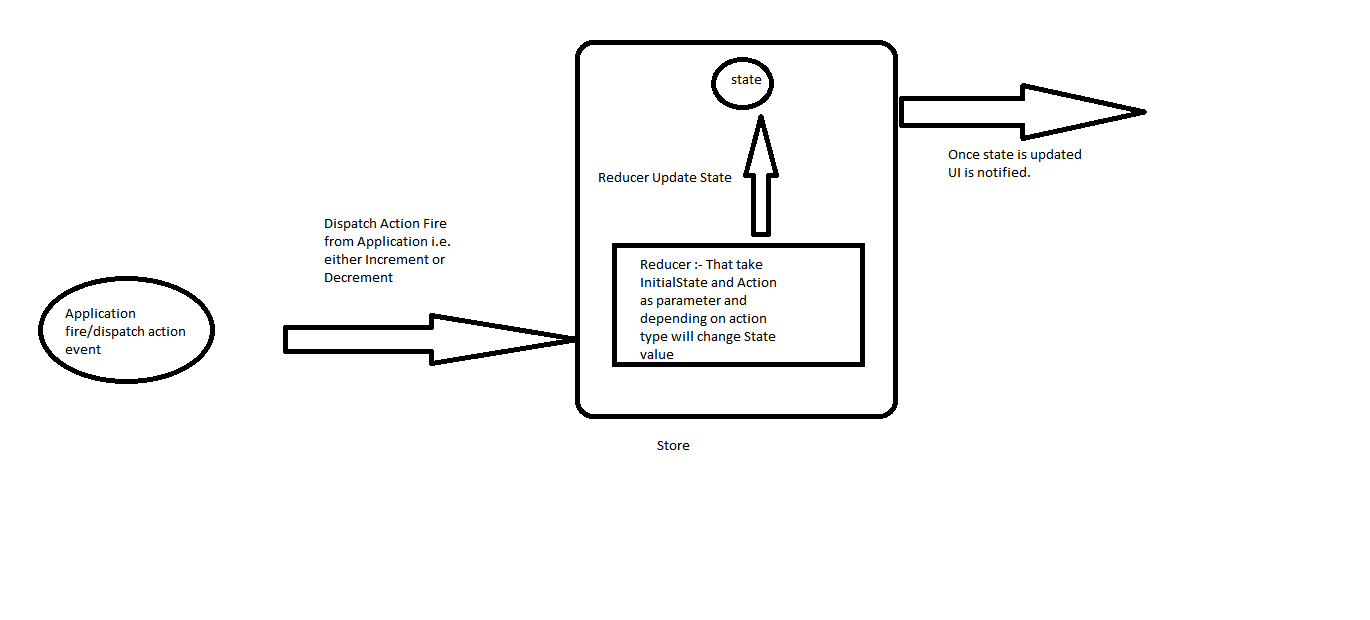
Lets take a simple example where in we have a varible named as counter whose value is store in the state. Through action we either increment or decrement the counter which in tern perform the increment or decrement of its state value in store. and fially we can get the updated inc/dec value of the counter stored as state in store.
Step :1 - Install redux from npm so that we can create store to accomodate state values. Use below command to install redux.
npm install redux --save
check in package.json file if the redux is added into dependencies. If you do not have package.json create it using command
npm init
Step 2:- First we need to create a store using below command
const { createStore } = require('redux')
const store = createStore()
Step 3:- Now store take Reducer as an argument so lets create a reducer
const myReducer = (state, action) => {
}
We can also define the initial state as shown below
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
}
Step 4:- Now lets assign this myReducer to our create store
const store = createStore(myReducer)
step 5:- As we did not have any UI applicaton that can fire the event to change the value of the counter inside store we can do it manually by dispatch methond as shown below in which we tell reducer that action is dispatched with its type as increment
store.dispatch({ type: 'increment' })
Step 5:- Now inside reducer we can check this action type and confirm what action need to be done with counter variable that is stored in global or store state
for increment we want to increase the value of counter with 1 and need to update that value in global state in store.
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
return newState
}
Step 5:- Now finally execute the below programe and you will see how we can do the increment and decrement of the counter value which is store as global state in store of redux.
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - 1
}
return newState
}
const store = createStore(myReducer)
console.log(store.getState())
store.dispatch({ type: 'increment' })
console.log(store.getState())
store.dispatch({ type: 'decrement' })
console.log(store.getState())
Out put
PS C:\Visual_Source_Code_WorkSpace_ReactJSNew\simplereduxexample> node .\simpleredux.js
{ counter: 1 }
{ counter: 2 }
{ counter: 1 }
now the question arise how i would be know automatically that value of global state i.e. counter inside store is changed. We dont want to manually track everytime checking global state change. For this we have beautiful concept of subscriber
store.subscribe(() => {
console.log("Nofied automatically that state is changed:- " + JSON.stringify(store.getState()))
})
and then we can remove all the console log.
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + 1
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - 1
}
return newState
}
const store = createStore(myReducer)
store.subscribe(() => {
console.log("Nofied automatically that state is changed:- " + JSON.stringify(store.getState()))
})
//console.log(store.getState())
store.dispatch({ type: 'increment' })
//console.log(store.getState())
store.dispatch({ type: 'decrement' })
//console.log(store.getState())
now lets say we also want to pass a value that will indicate how much we want to do increment and decreament and this value is called payload
i.e.
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + action.val
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - action.val
}
return newState
}
store.dispatch({ type: 'increment', val:10})
Final code will be
const { createStore } = require('redux')
const initialState = {
counter: 1
}
const myReducer = (state = initialState, action) => {
const newState = { ...state }
if (action.type === 'increment') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter + action.val
}
if (action.type === 'decrement') {
//as in react we do not directly modify the state without setState here we will do it by
//creating copy of the state using spread operators
newState.counter = newState.counter - action.val
}
return newState
}
const store = createStore(myReducer)
store.subscribe(() => {
console.log("Notified automatically that state is changed:- " + JSON.stringify(store.getState()))
})
//console.log(store.getState())
store.dispatch({ type: 'increment', val: 10 })
//console.log(store.getState())
store.dispatch({ type: 'decrement', val: 10 })
//console.log(store.getState())
OutPut :-
PS C:\Visual_Source_Code_WorkSpace_ReactJSNew\simplereduxexample> node .\simpleredux.js
Notified automatically that state is changed:- {"counter":10}
Notified automatically that state is changed:- {"counter":1}
No comments:
Post a Comment