I am using Node and NPM and Visual studio as IDE and npm http-server. Make sure to install the same. To manipulate the value of our file on html we are using jQuery.
Consider this is our html file
'<html>
'
'<head>
' <meta charset="UTF-8">
' <title>Siddhu Ethererum Example</title>
'
'</head>
'
'<body>
' <input type="text" id="amount">
'
' <p id="balanceValue"></p>
' <button id="deposite">Deposite</button>
' <button id="withdraw">Withdraw</button>
' <script src="https://cdn.jsdelivr.net/gh/ethereum/web3.js@1.0.0-beta.36/dist/web3.min.js"></script>
' <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" crossorigin="anonymous"></script>
' <script>
' var contract;
'
'
' $(document).ready(
' function () {
' web3 = new Web3(web3.currentProvider);
'
' var address = "Myaddress";
' var abi = [{
' "constant": false,
' "inputs": [{
' "name": "amount",
' "type": "int256"
' }],
' "name": "depositeMyBalance",
' "outputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "function"
' },
' {
' "constant": false,
' "inputs": [{
' "name": "amount",
' "type": "int256"
' }],
' "name": "withdrawMyBalance",
' "outputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "function"
' },
' {
' "inputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "constructor"
' },
' {
' "constant": true,
' "inputs": [],
' "name": "getBalanceValue",
' "outputs": [{
' "name": "",
' "type": "int256"
' }],
' "payable": false,
' "stateMutability": "view",
' "type": "function"
' }
' ];
' contract = new web3.eth.Contract(abi, address);
' contract.methods.getBalanceValue().call().then(
' function (bal) {
' console.log("bal"+bal);
' document.getElementById
' $("#balanceValue").html(bal);
' })
' })
' //Deposite
' $('#deposite').click(function()
' {
' var amount = 0;
' amount = parseInt($('#amount').val());
' console.log("amount"+amount);
'
' // web3.eth.getAccounts().then(e => console.log("---------------"+e));
' web3.eth.getAccounts().then(function(accounts)
' {
' var acc = accounts[0];
' console.log("acc"+ acc);
' return contract.methods.depositeMyBalance(amount).send({from: acc});
' }).then (function (tx)
' {
' console.log(tx);
' }).catch(function(tx)
' {
' console.log(tx);
' })
' })
' //Withdraw
' $('#withdraw').click(function()
' {
' var amount = 0;
' amount = parseInt($('#amount').val());
' console.log("amount"+amount);
'
' // web3.eth.getAccounts().then(e => console.log("---------------"+e));
' web3.eth.getAccounts().then(function(accounts)
' {
' var acc = accounts[0];
' console.log("acc"+ acc);
' return contract.methods.withdrawMyBalance(amount).send({from: acc});
' }).then (function (tx)
' {
' console.log(tx);
' }).catch(function(tx)
' {
' console.log(tx);
' })
' })
' </script>
'
'</body>
'
'</html>
This is our SOL files
pragma solidity ^0.4.24;
//Main class that will be used to perform operation on our block.
contract MyBank
{
//This is variable used for storing our balanceValue
int balanceValue;
//build constructor
constructor() public
{
//initialise the variable
balanceValue = 1;
}
//As getBalance is not manupulating the value it is used to show the data on the screen and use view returns(int)
function getBalanceValue() public view returns(int)
{
//return the current value of balanceValue
return balanceValue;
}
function withdrawMyBalance(int amount) public
{
balanceValue = balanceValue - amount;
}
function depositeMyBalance(int amount) public
{
balanceValue = balanceValue + amount;
}
}
Note :- For newer version when you try to get the Accounts details please check the MetaMask plugin of chorme. For safety reason you have been asked explicitly approve the access.
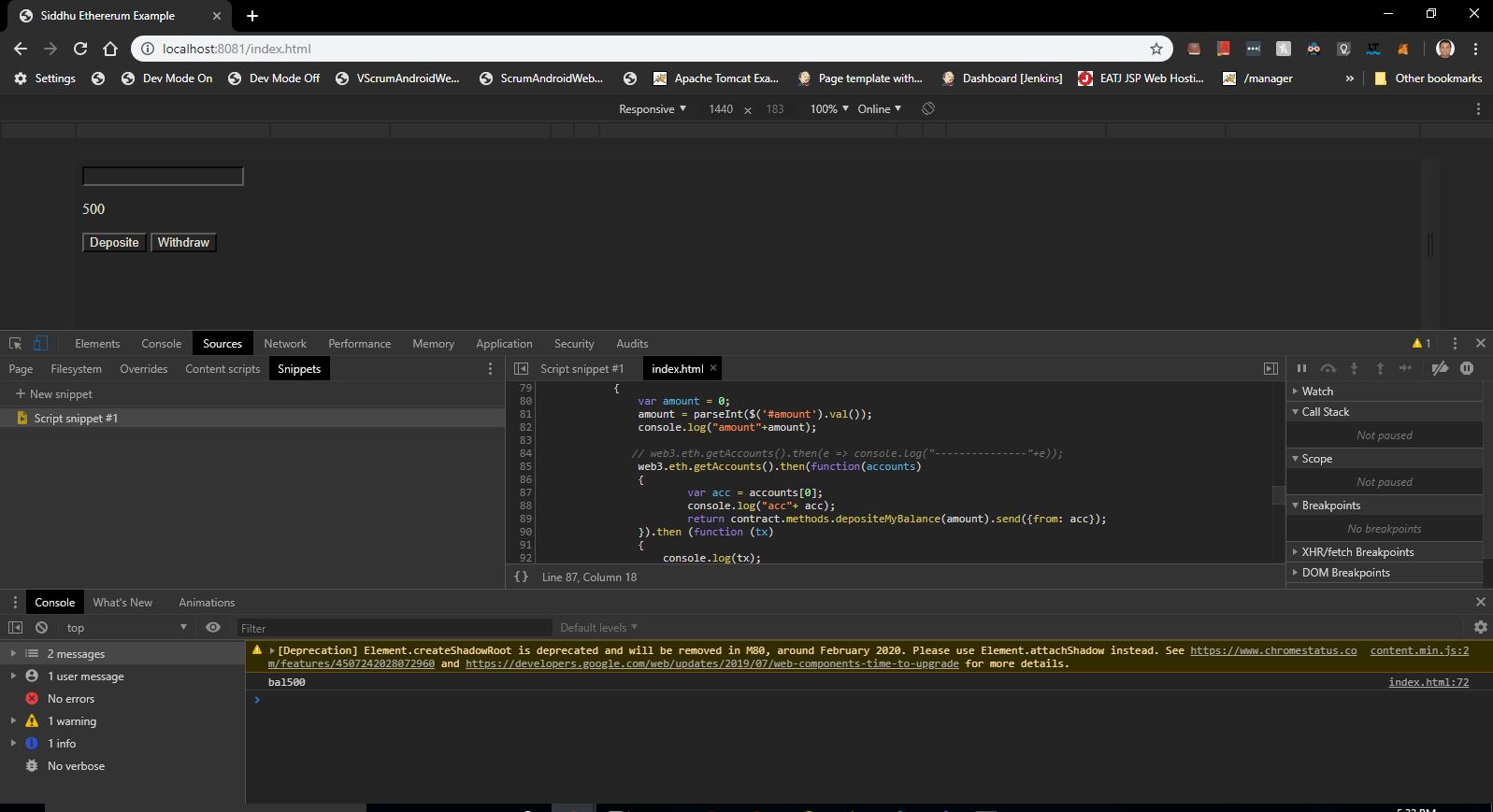
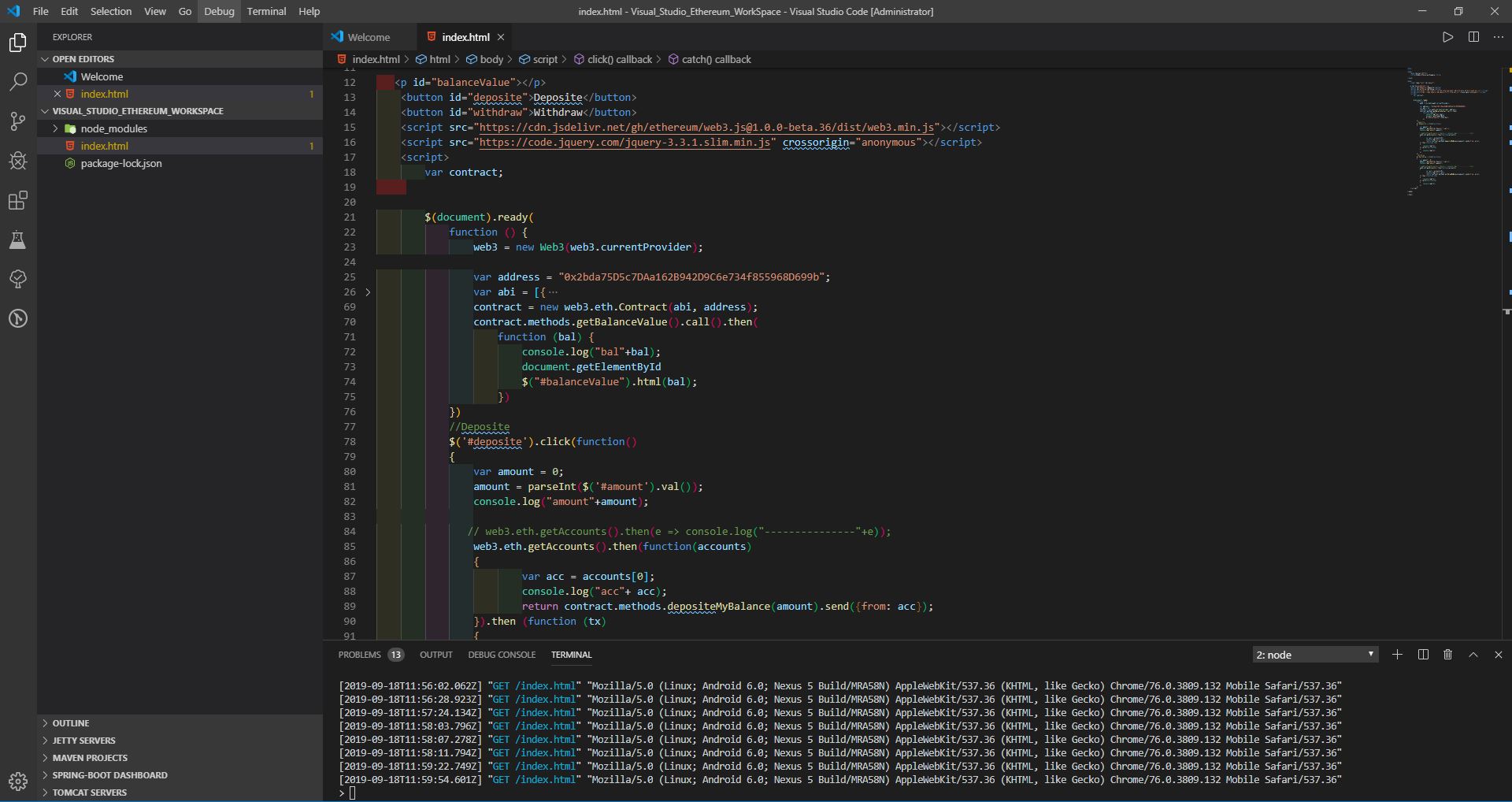
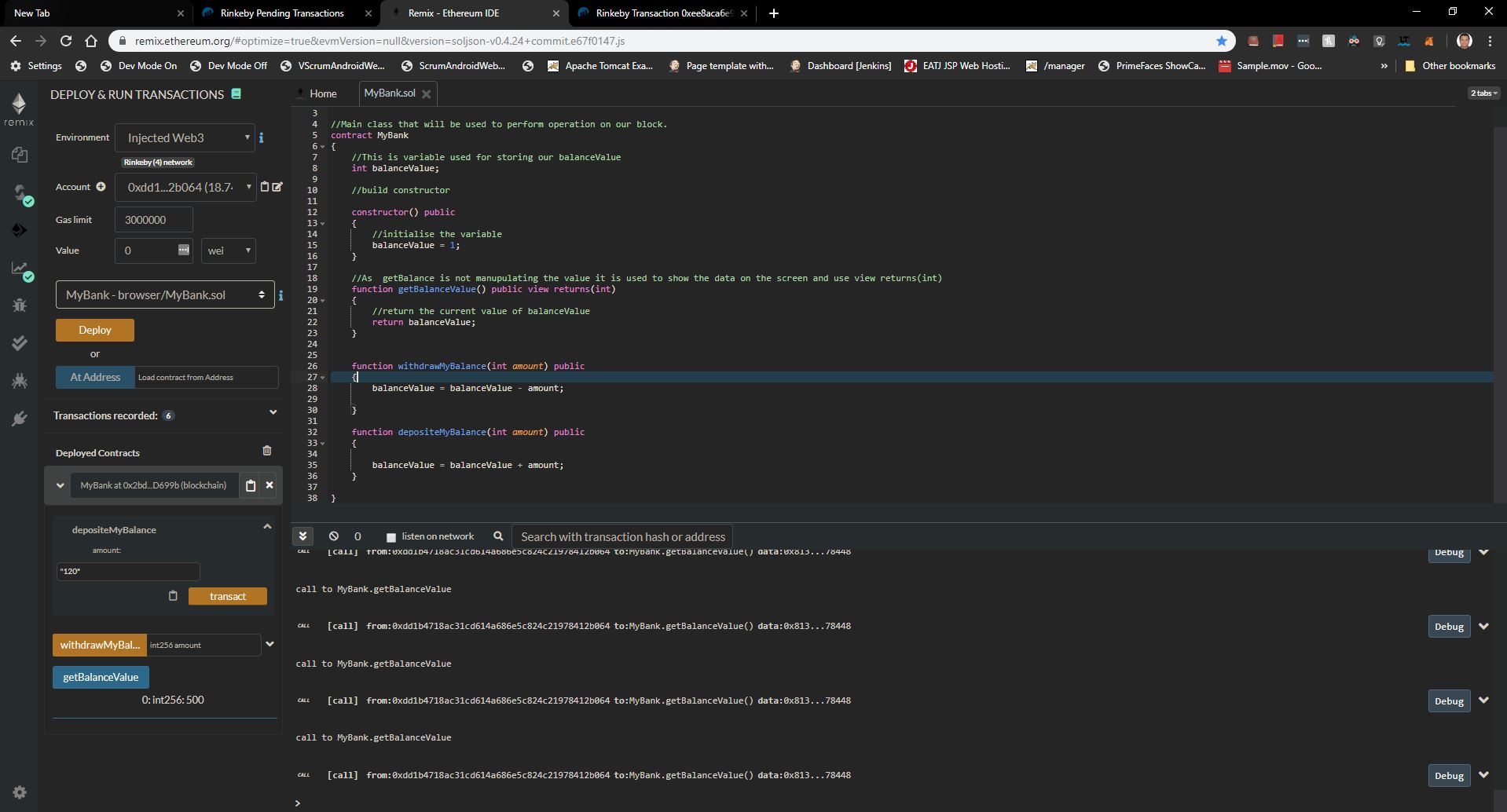
Consider this is our html file
'<html>
'
'<head>
' <meta charset="UTF-8">
' <title>Siddhu Ethererum Example</title>
'
'</head>
'
'<body>
' <input type="text" id="amount">
'
' <p id="balanceValue"></p>
' <button id="deposite">Deposite</button>
' <button id="withdraw">Withdraw</button>
' <script src="https://cdn.jsdelivr.net/gh/ethereum/web3.js@1.0.0-beta.36/dist/web3.min.js"></script>
' <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" crossorigin="anonymous"></script>
' <script>
' var contract;
'
'
' $(document).ready(
' function () {
' web3 = new Web3(web3.currentProvider);
'
' var address = "Myaddress";
' var abi = [{
' "constant": false,
' "inputs": [{
' "name": "amount",
' "type": "int256"
' }],
' "name": "depositeMyBalance",
' "outputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "function"
' },
' {
' "constant": false,
' "inputs": [{
' "name": "amount",
' "type": "int256"
' }],
' "name": "withdrawMyBalance",
' "outputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "function"
' },
' {
' "inputs": [],
' "payable": false,
' "stateMutability": "nonpayable",
' "type": "constructor"
' },
' {
' "constant": true,
' "inputs": [],
' "name": "getBalanceValue",
' "outputs": [{
' "name": "",
' "type": "int256"
' }],
' "payable": false,
' "stateMutability": "view",
' "type": "function"
' }
' ];
' contract = new web3.eth.Contract(abi, address);
' contract.methods.getBalanceValue().call().then(
' function (bal) {
' console.log("bal"+bal);
' document.getElementById
' $("#balanceValue").html(bal);
' })
' })
' //Deposite
' $('#deposite').click(function()
' {
' var amount = 0;
' amount = parseInt($('#amount').val());
' console.log("amount"+amount);
'
' // web3.eth.getAccounts().then(e => console.log("---------------"+e));
' web3.eth.getAccounts().then(function(accounts)
' {
' var acc = accounts[0];
' console.log("acc"+ acc);
' return contract.methods.depositeMyBalance(amount).send({from: acc});
' }).then (function (tx)
' {
' console.log(tx);
' }).catch(function(tx)
' {
' console.log(tx);
' })
' })
' //Withdraw
' $('#withdraw').click(function()
' {
' var amount = 0;
' amount = parseInt($('#amount').val());
' console.log("amount"+amount);
'
' // web3.eth.getAccounts().then(e => console.log("---------------"+e));
' web3.eth.getAccounts().then(function(accounts)
' {
' var acc = accounts[0];
' console.log("acc"+ acc);
' return contract.methods.withdrawMyBalance(amount).send({from: acc});
' }).then (function (tx)
' {
' console.log(tx);
' }).catch(function(tx)
' {
' console.log(tx);
' })
' })
' </script>
'
'</body>
'
'</html>
This is our SOL files
pragma solidity ^0.4.24;
//Main class that will be used to perform operation on our block.
contract MyBank
{
//This is variable used for storing our balanceValue
int balanceValue;
//build constructor
constructor() public
{
//initialise the variable
balanceValue = 1;
}
//As getBalance is not manupulating the value it is used to show the data on the screen and use view returns(int)
function getBalanceValue() public view returns(int)
{
//return the current value of balanceValue
return balanceValue;
}
function withdrawMyBalance(int amount) public
{
balanceValue = balanceValue - amount;
}
function depositeMyBalance(int amount) public
{
balanceValue = balanceValue + amount;
}
}
Note :- For newer version when you try to get the Accounts details please check the MetaMask plugin of chorme. For safety reason you have been asked explicitly approve the access.
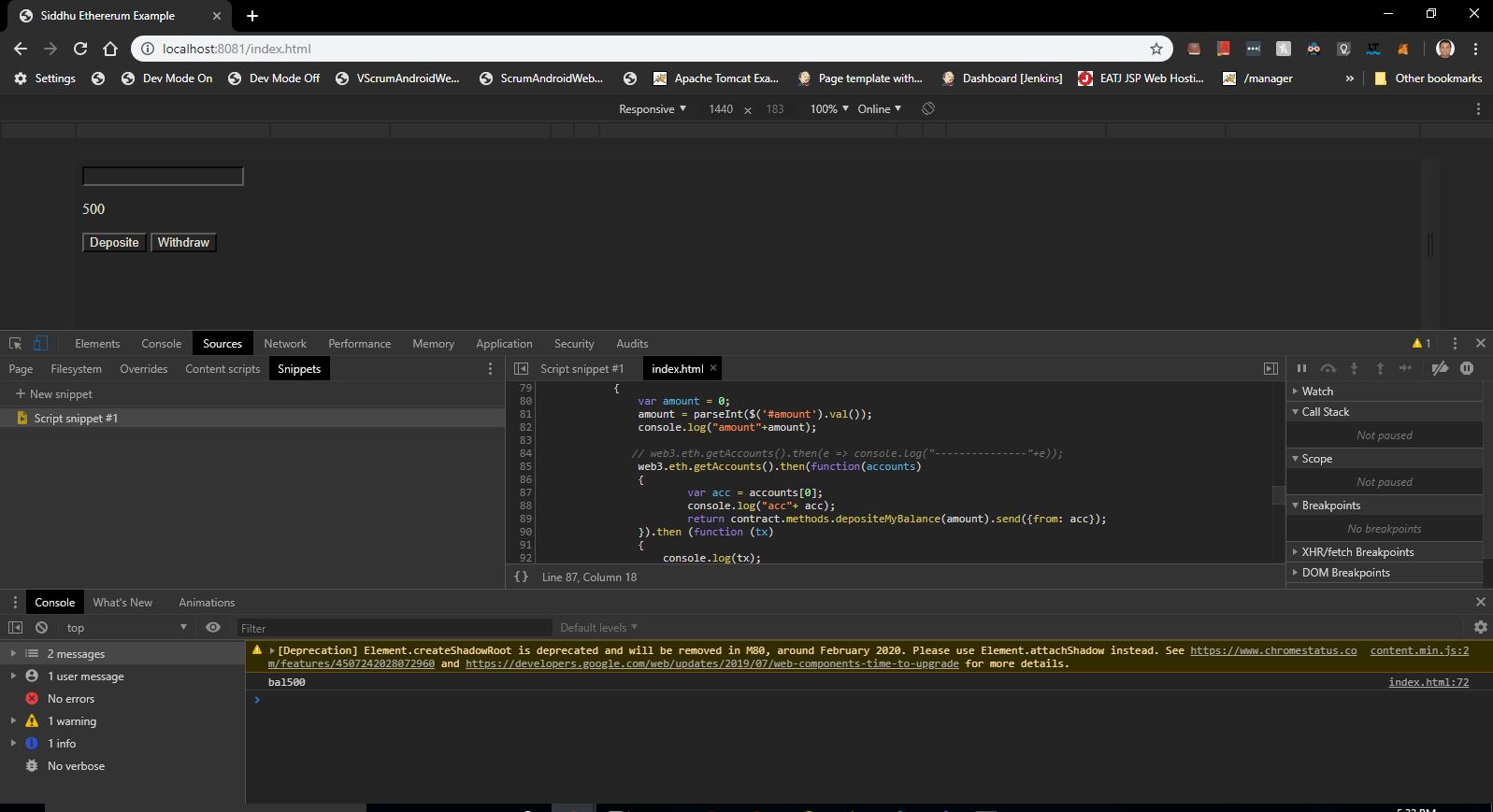
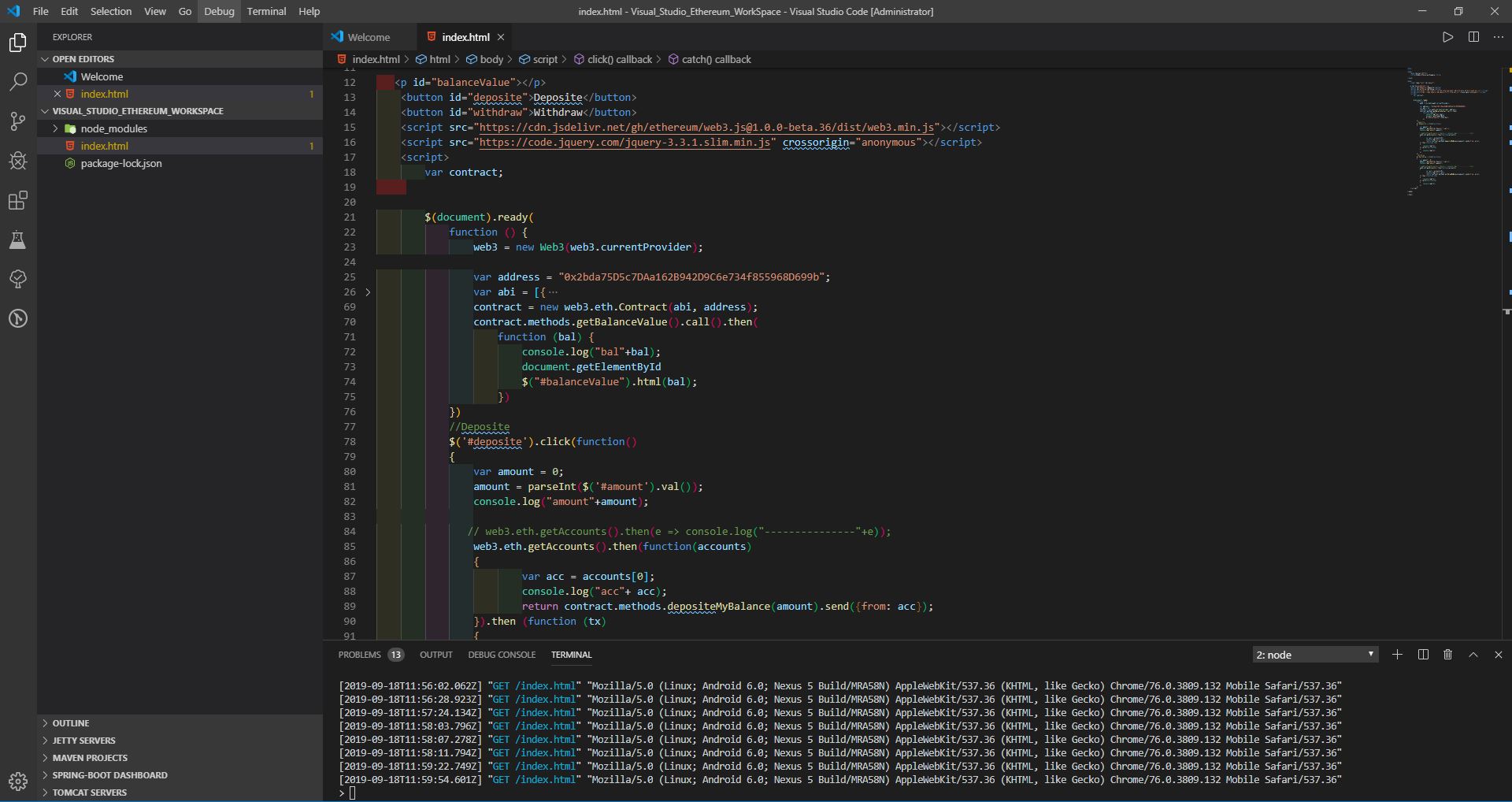
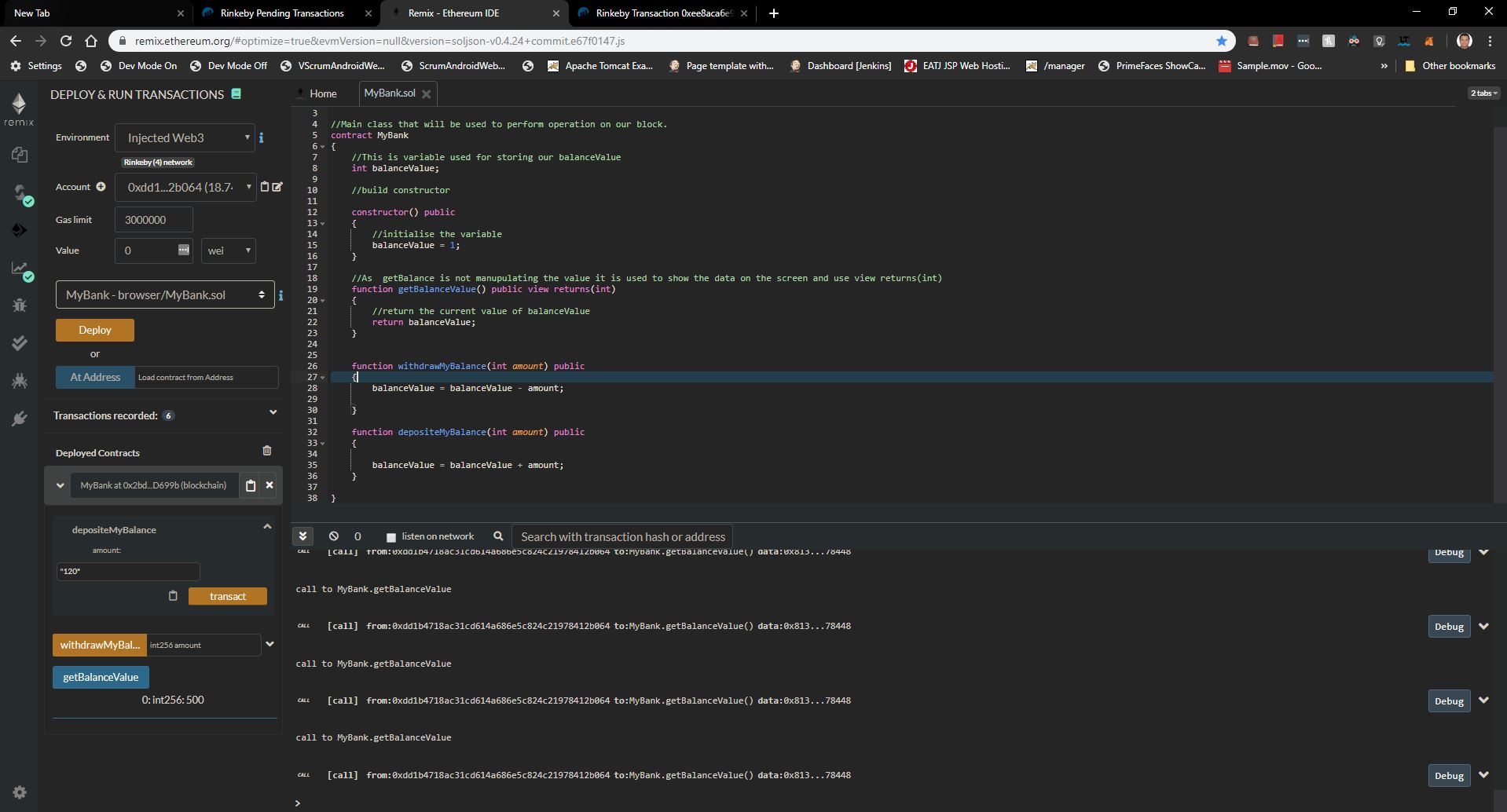
No comments:
Post a Comment