1) Install STS from the below given site. Chose your OS dependend IDE version.
https://spring.io/tools/sts/all
2) Create a simple Maven JAVA project with following details
org.springframework.social
spring-social-facebook
2.0.3.RELEASE
3) Modify your pom.xml as given below
<!--?xml version="1.0" encoding="UTF-8"?>
<!--project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<!--modelVersion>4.0.0<!--/modelVersion>
<!--project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<!--modelVersion>4.0.0<!--/modelVersion>
<!--groupId>org.springframework<!--/groupId>
<!--artifactId>gs-accessing-facebook<!--/artifactId>
<!--version>0.1.0<!--/version>
<!--artifactId>gs-accessing-facebook<!--/artifactId>
<!--version>0.1.0<!--/version>
<!--parent>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-starter-parent<!--/artifactId>
<!--version>1.4.2.RELEASE<!--/version>
<!--/parent>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-starter-parent<!--/artifactId>
<!--version>1.4.2.RELEASE<!--/version>
<!--/parent>
<!--dependencies>
<!--dependency>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-starter-thymeleaf<!--/artifactId>
<!--/dependency>
<!--dependency>
<!--groupId>org.springframework.social<!--/groupId>
<!--artifactId>spring-social-facebook<!--/artifactId>
<!--/dependency>
<!--/dependencies>
<!--dependency>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-starter-thymeleaf<!--/artifactId>
<!--/dependency>
<!--dependency>
<!--groupId>org.springframework.social<!--/groupId>
<!--artifactId>spring-social-facebook<!--/artifactId>
<!--/dependency>
<!--/dependencies>
<!--properties>
<!--java.version>1.8<!--/java.version>
<!--/properties>
<!--java.version>1.8<!--/java.version>
<!--/properties>
<!--build>
<!--plugins>
<!--plugin>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-maven-plugin<!--/artifactId>
<!--/plugin>
<!--/plugins>
<!--/build>
<!--plugins>
<!--plugin>
<!--groupId>org.springframework.boot<!--/groupId>
<!--artifactId>spring-boot-maven-plugin<!--/artifactId>
<!--/plugin>
<!--/plugins>
<!--/build>
<!--repositories>
<!--repository>
<!--id>spring-snapshots<!--/id>
<!--name>Spring Snapshots<!--/name>
<!--url>https://repo.spring.io/libs-milestone<!--/url>
<!--snapshots>
<!--enabled>false<!--/enabled>
<!--/snapshots>
<!--/repository>
<!--/repositories>
<!--pluginRepositories>
<!--pluginRepository>
<!--id>spring-snapshots<!--/id>
<!--name>Spring Snapshots<!--/name>
<!--url>https://repo.spring.io/libs-milestone<!--/url>
<!--snapshots>
<!--enabled>false<!--/enabled>
<!--/snapshots>
<!--/pluginRepository>
<!--/pluginRepositories>
<!--repository>
<!--id>spring-snapshots<!--/id>
<!--name>Spring Snapshots<!--/name>
<!--url>https://repo.spring.io/libs-milestone<!--/url>
<!--snapshots>
<!--enabled>false<!--/enabled>
<!--/snapshots>
<!--/repository>
<!--/repositories>
<!--pluginRepositories>
<!--pluginRepository>
<!--id>spring-snapshots<!--/id>
<!--name>Spring Snapshots<!--/name>
<!--url>https://repo.spring.io/libs-milestone<!--/url>
<!--snapshots>
<!--enabled>false<!--/enabled>
<!--/snapshots>
<!--/pluginRepository>
<!--/pluginRepositories>
<!--/project>
4) Follow the instruction givn in the below site
https://spring.io/guides/gs/accessing-facebook/
4) Follow the instruction givn in the below site
https://spring.io/guides/gs/accessing-facebook/
Follow step that is provided for Maven build. If you are using Gradle follow the same.
5) Register or create your Facebook application as given below
https://spring.io/guides/gs/register-facebook-app/
https://spring.io/guides/gs/register-facebook-app/



from here we will get two things consumer key and consumer secret. This is used to call the Facebook API.



6) Make sure your application project folder structure look like the same. You can all download the zip from this site
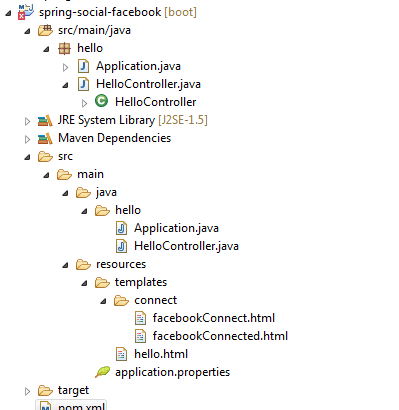
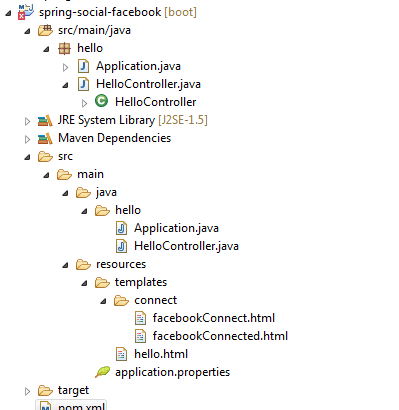
7) Build and run your Maven project using following configuration
9) Once server is started you will be able to see following screen

10) Click on Connect facebook and if you get below screen

follow the steps given in
http://stackoverflow.com/questions/37063685/facebook-oauth-the-domain-of-this-url-isnt-included-in-the-apps-domain

11) Click on Click here and we will see


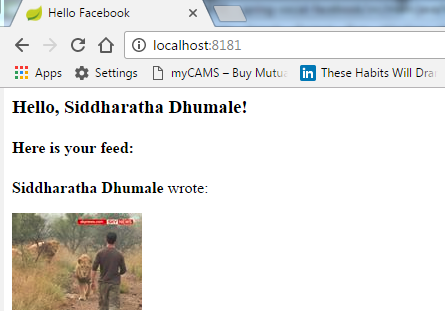
package hello;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
SpringApplication.run(Application.class, args);
}
}
package hello;
import org.springframework.social.connect.ConnectionRepository;
import org.springframework.social.facebook.api.Facebook;
import org.springframework.social.facebook.api.PagedList;
import org.springframework.social.facebook.api.Post;
import org.springframework.social.facebook.api.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.social.facebook.api.Facebook;
import org.springframework.social.facebook.api.PagedList;
import org.springframework.social.facebook.api.Post;
import org.springframework.social.facebook.api.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/")
public class HelloController {
@RequestMapping("/")
public class HelloController {
private Facebook facebook;
private ConnectionRepository connectionRepository;
private ConnectionRepository connectionRepository;
public HelloController(Facebook facebook, ConnectionRepository connectionRepository) {
this.facebook = facebook;
this.connectionRepository = connectionRepository;
}
this.facebook = facebook;
this.connectionRepository = connectionRepository;
}
@GetMapping
public String helloFacebook(Model model) {
if (connectionRepository.findPrimaryConnection(Facebook.class) == null) {
return "redirect:/connect/facebook";
}
public String helloFacebook(Model model) {
if (connectionRepository.findPrimaryConnection(Facebook.class) == null) {
return "redirect:/connect/facebook";
}
/* model.addAttribute("facebookProfile", facebook.userOperations().getUserProfile());
PagedList
model.addAttribute("feed", feed);
return "hello";*/
String [] fields = { "id","name","birthday","email","location","hometown","gender","first_name","last_name"};
User user = facebook.fetchObject("me", User.class, fields);
String name=user.getName();
String birthday=user.getBirthday();
String email=user.getEmail();
String gender=user.getGender();
String firstname=user.getFirstName();
String lastname=user.getLastName();
model.addAttribute("name",name );
model.addAttribute("birthday",birthday );
model.addAttribute("email",email );
model.addAttribute("gender",gender);
model.addAttribute("firstname",firstname);
model.addAttribute("lastname",lastname);
model.addAttribute("facebookProfile", facebook.fetchObject("me", User.class, fields));
PagedList
model.addAttribute("feed", feed);
return "hello";
}
}
spring.social.facebook.app-id=XXXXXX
spring.social.facebook.app-secret=XXXXX
spring.social.facebook.app-secret=XXXXX
No comments:
Post a Comment