Express comes inbuild with expressgenerator that helps us to set the defualt frame work for creating Nodejs application using ExpressJS frame work.
https://expressjs.com/en/starter/generator.html
Lets try to use it
First create package.json using
npm init
then install express using
npm install express --save
check in package.json if express is install
install nodemon for auto refresh
npm install -g nodemon
then install express-generator using below command
npx express-generator
or
npm install -g express-generator
I prefer to use second command as it help me to further take view engine as my need i.e. npm install -g express-generator
Check package.json is updated with the express-generator.
Note:- If you want to create project with express you can do it in two ways
1- Directly executing command
npx express-generator
This will create a project with its own Package.json and view as JADE by default
2- Use below this command to install the express generator
npm install -g express-generator
then use below command to create a project name as myapp and view as .pug or any of the template you want as an view engine.
express --view=pug myapp
3- If you have your project folder and you dont want to create a new folder and want PUG as view use this command
express --view=pug
Note:- Now as I already had my folder ready i will use this command express --view=pug
By defualt The generated app has the following directory structure:
.
+-- app.js
+-- bin
¦ +-- www
+-- package.json
+-- public
¦ +-- images
¦ +-- javascripts
¦ +-- stylesheets
¦ +-- style.css
+-- routes
¦ +-- index.js
¦ +-- users.js
+-- views
+-- error.pug
+-- index.pug
+-- layout.pug
7 directories, 9 files
you can run the application using
set DEBUG=myapp:* & npm start
After executing express --view=pug
PS C:\Visual_Source_Code_WorkSpace_NodeJS\expressgeneratorexample> express --view=pug
destination is not empty, continue? [y/N] y
create : public\
create : public\javascripts\
create : public\images\
create : public\stylesheets\
create : public\stylesheets\style.css
create : routes\
create : routes\index.js
create : routes\users.js
create : views\
create : views\error.pug
create : views\index.pug
create : views\layout.pug
create : app.js
create : package.json
create : bin\
create : bin\www
install dependencies:
> npm install
run the app:
> SET DEBUG=expressgeneratorexample:* & npm start
Now as shown above first execute this command to install all dependencies
> npm install
and then run the app using npm start or go one step above
SET DEBUG=expressgeneratorexample:*; npm start
and check brower http://localhost:3000/ you will be able to see first defualt expressgenerator screen
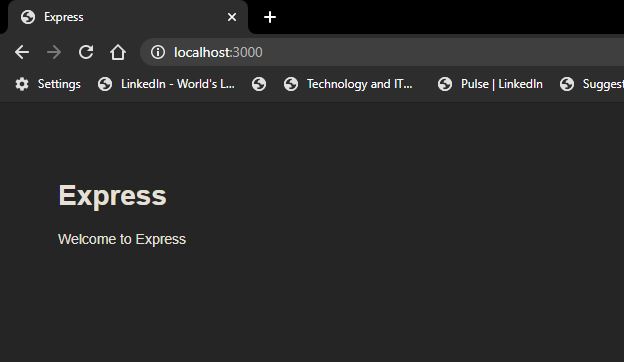
We can also use other Template Engine Basic Introduction
Express frame work give us many UI template to display the runtime data on the screen web/mobile using nodejs. Few of them are pug, jade etc. You can find list of the template on this location supported by expressjs.
https://expressjs.com/en/resources/template-engines.html
Lets discuss one more template engine We will discuss few of them
1- ejs
To use this first we need to install pug view using command
npm install ejs --save
once it is installed use below line to inform express to indicate which engine need to be used for rendring page on ui.
app.set('view engine', 'ejs')
'<html>
This is EJS file example
'<head>'</head>
'<body>
'<p>'<%=title %>'</p>
'</body>
'</html>
Now lets run the applications and check the url
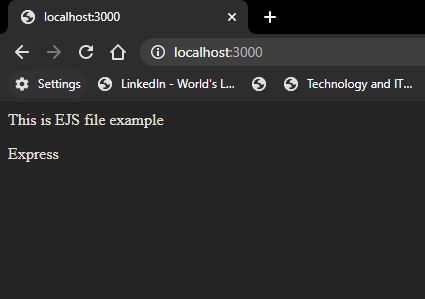
Download:- https://github.com/shdhumale/expressgeneratorexample
https://expressjs.com/en/starter/generator.html
Lets try to use it
First create package.json using
npm init
then install express using
npm install express --save
check in package.json if express is install
install nodemon for auto refresh
npm install -g nodemon
then install express-generator using below command
npx express-generator
or
npm install -g express-generator
I prefer to use second command as it help me to further take view engine as my need i.e. npm install -g express-generator
Check package.json is updated with the express-generator.
Note:- If you want to create project with express you can do it in two ways
1- Directly executing command
npx express-generator
This will create a project with its own Package.json and view as JADE by default
2- Use below this command to install the express generator
npm install -g express-generator
then use below command to create a project name as myapp and view as .pug or any of the template you want as an view engine.
express --view=pug myapp
3- If you have your project folder and you dont want to create a new folder and want PUG as view use this command
express --view=pug
Note:- Now as I already had my folder ready i will use this command express --view=pug
By defualt The generated app has the following directory structure:
.
+-- app.js
+-- bin
¦ +-- www
+-- package.json
+-- public
¦ +-- images
¦ +-- javascripts
¦ +-- stylesheets
¦ +-- style.css
+-- routes
¦ +-- index.js
¦ +-- users.js
+-- views
+-- error.pug
+-- index.pug
+-- layout.pug
7 directories, 9 files
you can run the application using
set DEBUG=myapp:* & npm start
After executing express --view=pug
PS C:\Visual_Source_Code_WorkSpace_NodeJS\expressgeneratorexample> express --view=pug
destination is not empty, continue? [y/N] y
create : public\
create : public\javascripts\
create : public\images\
create : public\stylesheets\
create : public\stylesheets\style.css
create : routes\
create : routes\index.js
create : routes\users.js
create : views\
create : views\error.pug
create : views\index.pug
create : views\layout.pug
create : app.js
create : package.json
create : bin\
create : bin\www
install dependencies:
> npm install
run the app:
> SET DEBUG=expressgeneratorexample:* & npm start
Now as shown above first execute this command to install all dependencies
> npm install
and then run the app using npm start or go one step above
SET DEBUG=expressgeneratorexample:*; npm start
and check brower http://localhost:3000/ you will be able to see first defualt expressgenerator screen
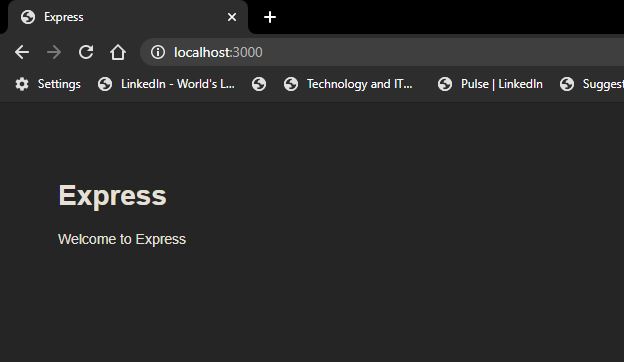
We can also use other Template Engine Basic Introduction
Express frame work give us many UI template to display the runtime data on the screen web/mobile using nodejs. Few of them are pug, jade etc. You can find list of the template on this location supported by expressjs.
https://expressjs.com/en/resources/template-engines.html
Lets discuss one more template engine We will discuss few of them
1- ejs
To use this first we need to install pug view using command
npm install ejs --save
once it is installed use below line to inform express to indicate which engine need to be used for rendring page on ui.
app.set('view engine', 'ejs')
'<html>
This is EJS file example
'<head>'</head>
'<body>
'<p>'<%=title %>'</p>
'</body>
'</html>
Now lets run the applications and check the url
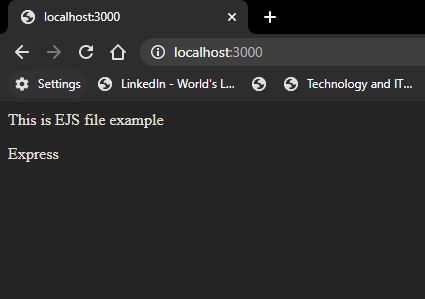
Download:- https://github.com/shdhumale/expressgeneratorexample
No comments:
Post a Comment